mirror of https://github.com/inolen/redream.git
updated to custom imgui fork
This commit is contained in:
parent
0263b127d6
commit
47b600e2da
|
@ -2,6 +2,10 @@
|
|||
#include "../imgui/imgui.h"
|
||||
#include "cimgui.h"
|
||||
|
||||
// to use placement new
|
||||
#define IMGUI_DEFINE_PLACEMENT_NEW
|
||||
#include "../imgui/imgui_internal.h"
|
||||
|
||||
CIMGUI_API ImGuiIO* igGetIO()
|
||||
{
|
||||
return &ImGui::GetIO();
|
||||
|
@ -406,6 +410,16 @@ CIMGUI_API void igPopButtonRepeat()
|
|||
return ImGui::PopButtonRepeat();
|
||||
}
|
||||
|
||||
CIMGUI_API void igPushNavDefaultFocus(bool default_focus)
|
||||
{
|
||||
return ImGui::PushNavDefaultFocus(default_focus);
|
||||
}
|
||||
|
||||
CIMGUI_API void igPopNavDefaultFocus()
|
||||
{
|
||||
return ImGui::PopNavDefaultFocus();
|
||||
}
|
||||
|
||||
// Tooltip
|
||||
CIMGUI_API void igSetTooltip(CONST char* fmt, ...)
|
||||
{
|
||||
|
@ -633,43 +647,43 @@ CIMGUI_API int igGetColumnsCount()
|
|||
// ID scopes
|
||||
// If you are creating widgets in a loop you most likely want to push a unique identifier so ImGui can differentiate them
|
||||
// You can also use "##extra" within your widget name to distinguish them from each others (see 'Programmer Guide')
|
||||
CIMGUI_API void igPushIdStr(CONST char* str_id)
|
||||
CIMGUI_API void igPushIDStr(CONST char* str_id)
|
||||
{
|
||||
return ImGui::PushID(str_id);
|
||||
}
|
||||
|
||||
CIMGUI_API void igPushIdStrRange(CONST char* str_begin, CONST char* str_end)
|
||||
CIMGUI_API void igPushIDStrRange(CONST char* str_begin, CONST char* str_end)
|
||||
{
|
||||
return ImGui::PushID(str_begin, str_end);
|
||||
}
|
||||
|
||||
|
||||
CIMGUI_API void igPushIdPtr(CONST void* ptr_id)
|
||||
CIMGUI_API void igPushIDPtr(CONST void* ptr_id)
|
||||
{
|
||||
return ImGui::PushID(ptr_id);
|
||||
}
|
||||
|
||||
CIMGUI_API void igPushIdInt(int int_id)
|
||||
CIMGUI_API void igPushIDInt(int int_id)
|
||||
{
|
||||
return ImGui::PushID(int_id);
|
||||
}
|
||||
|
||||
CIMGUI_API void igPopId()
|
||||
CIMGUI_API void igPopID()
|
||||
{
|
||||
return ImGui::PopID();
|
||||
}
|
||||
|
||||
CIMGUI_API ImGuiID igGetIdStr(CONST char* str_id)
|
||||
CIMGUI_API ImGuiID igGetIDStr(CONST char* str_id)
|
||||
{
|
||||
return ImGui::GetID(str_id);
|
||||
}
|
||||
|
||||
CIMGUI_API ImGuiID igGetIdStrRange(CONST char* str_begin, CONST char* str_end)
|
||||
CIMGUI_API ImGuiID igGetIDStrRange(CONST char* str_begin, CONST char* str_end)
|
||||
{
|
||||
return ImGui::GetID(str_begin, str_end);
|
||||
}
|
||||
|
||||
CIMGUI_API ImGuiID igGetIdPtr(CONST void* ptr_id)
|
||||
CIMGUI_API ImGuiID igGetIDPtr(CONST void* ptr_id)
|
||||
{
|
||||
return ImGui::GetID(ptr_id);
|
||||
}
|
||||
|
@ -922,7 +936,7 @@ CIMGUI_API bool igColorPicker3(CONST char* label, float col[3], ImGuiColorEditFl
|
|||
|
||||
CIMGUI_API bool igColorPicker4(CONST char* label, float col[4], ImGuiColorEditFlags flags, CONST float* ref_col)
|
||||
{
|
||||
return ImGui::ColorPicker4(label, col, flags);
|
||||
return ImGui::ColorPicker4(label, col, flags, ref_col);
|
||||
}
|
||||
|
||||
CIMGUI_API bool igColorButton(CONST char* desc_id, CONST ImVec4 col, ImGuiColorEditFlags flags, CONST ImVec2 size)
|
||||
|
@ -1277,7 +1291,7 @@ CIMGUI_API void igLogText(CONST char* fmt, ...)
|
|||
char buffer[256];
|
||||
va_list args;
|
||||
va_start(args, fmt);
|
||||
snprintf(buffer, 256, fmt, args);
|
||||
vsnprintf(buffer, 256, fmt, args);
|
||||
va_end(args);
|
||||
|
||||
ImGui::LogText("%s",buffer);
|
||||
|
@ -1299,11 +1313,6 @@ CIMGUI_API bool igIsItemHovered()
|
|||
return ImGui::IsItemHovered();
|
||||
}
|
||||
|
||||
CIMGUI_API bool igIsItemRectHovered()
|
||||
{
|
||||
return ImGui::IsItemRectHovered();
|
||||
}
|
||||
|
||||
CIMGUI_API bool igIsItemActive()
|
||||
{
|
||||
return ImGui::IsItemActive();
|
||||
|
@ -1334,11 +1343,6 @@ CIMGUI_API bool igIsAnyItemActive()
|
|||
return ImGui::IsAnyItemActive();
|
||||
}
|
||||
|
||||
CIMGUI_API bool igIsAnyItemFocused()
|
||||
{
|
||||
return ImGui::IsAnyItemFocused();
|
||||
}
|
||||
|
||||
CIMGUI_API void igGetItemRectMin(ImVec2* pOut)
|
||||
{
|
||||
*pOut = ImGui::GetItemRectMin();
|
||||
|
@ -1359,11 +1363,6 @@ CIMGUI_API void igSetItemAllowOverlap()
|
|||
ImGui::SetItemAllowOverlap();
|
||||
}
|
||||
|
||||
CIMGUI_API void igSetItemDefaultFocus()
|
||||
{
|
||||
ImGui::SetItemDefaultFocus();
|
||||
}
|
||||
|
||||
CIMGUI_API bool igIsWindowFocused()
|
||||
{
|
||||
return ImGui::IsWindowFocused();
|
||||
|
@ -1374,11 +1373,6 @@ CIMGUI_API bool igIsWindowHovered()
|
|||
return ImGui::IsWindowHovered();
|
||||
}
|
||||
|
||||
CIMGUI_API bool igIsWindowRectHovered()
|
||||
{
|
||||
return ImGui::IsWindowRectHovered();
|
||||
}
|
||||
|
||||
CIMGUI_API bool igIsRootWindowFocused()
|
||||
{
|
||||
return ImGui::IsRootWindowFocused();
|
||||
|
@ -1429,11 +1423,6 @@ CIMGUI_API bool igIsKeyReleased(int user_key_index)
|
|||
return ImGui::IsKeyReleased(user_key_index);
|
||||
}
|
||||
|
||||
CIMGUI_API int igGetKeyPressedAmount(int key_index, float repeat_delay, float rate)
|
||||
{
|
||||
return ImGui::GetKeyPressedAmount(key_index, repeat_delay, rate);
|
||||
}
|
||||
|
||||
CIMGUI_API bool igIsMouseDown(int button)
|
||||
{
|
||||
return ImGui::IsMouseDown(button);
|
||||
|
@ -1624,121 +1613,202 @@ CIMGUI_API void ImGuiIO_ClearInputCharacters()
|
|||
return ImGui::GetIO().ClearInputCharacters();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextFilter_Init(struct ImGuiTextFilter* filter, const char* default_filter)
|
||||
CIMGUI_API struct ImGuiTextFilter* ImGuiTextFilter_Create(const char* default_filter)
|
||||
{
|
||||
*filter = ImGuiTextFilter(default_filter);
|
||||
ImGuiTextFilter* filter = (ImGuiTextFilter*)ImGui::MemAlloc(sizeof(ImGuiTextFilter));
|
||||
IM_PLACEMENT_NEW(filter) ImGuiTextFilter();
|
||||
return filter;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextFilter_Clear(struct ImGuiTextFilter* filter)
|
||||
CIMGUI_API void ImGuiTextFilter_Destroy(struct ImGuiTextFilter* filter)
|
||||
{
|
||||
filter->Clear();
|
||||
filter->~ImGuiTextFilter();
|
||||
ImGui::MemFree(filter);
|
||||
}
|
||||
|
||||
CIMGUI_API bool ImGuiTextFilter_Draw(struct ImGuiTextFilter* filter, const char* label, float width)
|
||||
CIMGUI_API void ImGuiTextFilter_Clear(struct ImGuiTextFilter* filter)
|
||||
{
|
||||
return filter->Draw(label, width);
|
||||
filter->Clear();
|
||||
}
|
||||
|
||||
CIMGUI_API bool ImGuiTextFilter_PassFilter(struct ImGuiTextFilter* filter, const char* text, const char* text_end)
|
||||
CIMGUI_API bool ImGuiTextFilter_Draw(struct ImGuiTextFilter* filter, const char* label, float width)
|
||||
{
|
||||
return filter->PassFilter(text, text_end);
|
||||
return filter->Draw(label, width);
|
||||
}
|
||||
|
||||
CIMGUI_API bool ImGuiTextFilter_IsActive(struct ImGuiTextFilter* filter)
|
||||
CIMGUI_API bool ImGuiTextFilter_PassFilter(const struct ImGuiTextFilter* filter, const char* text, const char* text_end)
|
||||
{
|
||||
return filter->IsActive();
|
||||
}
|
||||
CIMGUI_API void ImGuiTextFilter_Build(struct ImGuiTextFilter* filter)
|
||||
{
|
||||
filter->Build();
|
||||
return filter->PassFilter(text, text_end);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextEditCallbackData_DeleteChars(struct ImGuiTextEditCallbackData* data, int pos, int bytes_count)
|
||||
CIMGUI_API bool ImGuiTextFilter_IsActive(const struct ImGuiTextFilter* filter)
|
||||
{
|
||||
data->DeleteChars(pos, bytes_count);
|
||||
return filter->IsActive();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextEditCallbackData_InsertChars(struct ImGuiTextEditCallbackData* data, int pos, const char* text, const char* text_end)
|
||||
CIMGUI_API void ImGuiTextFilter_Build(struct ImGuiTextFilter* filter)
|
||||
{
|
||||
data->InsertChars(pos, text, text_end);
|
||||
filter->Build();
|
||||
}
|
||||
|
||||
CIMGUI_API bool ImGuiTextEditCallbackData_HasSelection(struct ImGuiTextEditCallbackData* data)
|
||||
CIMGUI_API const char* ImGuiTextFilter_GetInputBuf(struct ImGuiTextFilter* filter)
|
||||
{
|
||||
return data->HasSelection();
|
||||
return filter->InputBuf;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiStorage_Init(struct ImGuiStorage* storage)
|
||||
CIMGUI_API struct ImGuiTextBuffer* ImGuiTextBuffer_Create()
|
||||
{
|
||||
*storage = ImGuiStorage();
|
||||
ImGuiTextBuffer* buffer = (ImGuiTextBuffer*)ImGui::MemAlloc(sizeof(ImGuiTextBuffer));
|
||||
IM_PLACEMENT_NEW(buffer) ImGuiTextBuffer();
|
||||
return buffer;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextBuffer_Destroy(struct ImGuiTextBuffer* buffer)
|
||||
{
|
||||
buffer->~ImGuiTextBuffer();
|
||||
ImGui::MemFree(buffer);
|
||||
}
|
||||
|
||||
CIMGUI_API char ImGuiTextBuffer_index(struct ImGuiTextBuffer* buffer, int i)
|
||||
{
|
||||
return (*buffer)[i];
|
||||
}
|
||||
|
||||
CIMGUI_API const char* ImGuiTextBuffer_begin(const struct ImGuiTextBuffer* buffer)
|
||||
{
|
||||
return buffer->begin();
|
||||
}
|
||||
|
||||
CIMGUI_API const char* ImGuiTextBuffer_end(const struct ImGuiTextBuffer* buffer)
|
||||
{
|
||||
return buffer->end();
|
||||
}
|
||||
|
||||
CIMGUI_API int ImGuiTextBuffer_size(const struct ImGuiTextBuffer* buffer)
|
||||
{
|
||||
return buffer->size();
|
||||
}
|
||||
|
||||
CIMGUI_API bool ImGuiTextBuffer_empty(struct ImGuiTextBuffer* buffer)
|
||||
{
|
||||
return buffer->empty();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextBuffer_clear(struct ImGuiTextBuffer* buffer)
|
||||
{
|
||||
buffer->clear();
|
||||
}
|
||||
|
||||
CIMGUI_API const char* ImGuiTextBuffer_c_str(const struct ImGuiTextBuffer* buffer)
|
||||
{
|
||||
return buffer->c_str();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextBuffer_append(struct ImGuiTextBuffer* buffer, const char* fmt, ...)
|
||||
{
|
||||
va_list args;
|
||||
va_start(args, fmt);
|
||||
buffer->appendv(fmt, args);
|
||||
va_end(args);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextBuffer_appendv(struct ImGuiTextBuffer* buffer, const char* fmt, va_list args)
|
||||
{
|
||||
buffer->appendv(fmt, args);
|
||||
}
|
||||
|
||||
CIMGUI_API struct ImGuiStorage* ImGuiStorage_Create() {
|
||||
ImGuiStorage* storage = (ImGuiStorage*)ImGui::MemAlloc(sizeof(ImGuiStorage));
|
||||
IM_PLACEMENT_NEW(storage) ImGuiStorage();
|
||||
return storage;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiStorage_Destroy(struct ImGuiStorage* storage) {
|
||||
storage->~ImGuiStorage();
|
||||
ImGui::MemFree(storage);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiStorage_Clear(struct ImGuiStorage* storage)
|
||||
{
|
||||
storage->Clear();
|
||||
storage->Clear();
|
||||
}
|
||||
|
||||
CIMGUI_API int ImGuiStorage_GetInt(struct ImGuiStorage* storage, ImGuiID key, int default_val)
|
||||
{
|
||||
return storage->GetInt(key, default_val);
|
||||
return storage->GetInt(key, default_val);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiStorage_SetInt(struct ImGuiStorage* storage, ImGuiID key, int val)
|
||||
{
|
||||
storage->SetInt(key, val);
|
||||
storage->SetInt(key, val);
|
||||
}
|
||||
|
||||
CIMGUI_API bool ImGuiStorage_GetBool(struct ImGuiStorage* storage, ImGuiID key, bool default_val)
|
||||
{
|
||||
return storage->GetBool(key, default_val);
|
||||
return storage->GetBool(key, default_val);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiStorage_SetBool(struct ImGuiStorage* storage, ImGuiID key, bool val)
|
||||
{
|
||||
storage->SetBool(key, val);
|
||||
storage->SetBool(key, val);
|
||||
}
|
||||
|
||||
CIMGUI_API float ImGuiStorage_GetFloat(struct ImGuiStorage* storage, ImGuiID key, float default_val)
|
||||
{
|
||||
return storage->GetFloat(key, default_val);
|
||||
return storage->GetFloat(key, default_val);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiStorage_SetFloat(struct ImGuiStorage* storage, ImGuiID key, float val)
|
||||
{
|
||||
storage->SetFloat(key, val);
|
||||
storage->SetFloat(key, val);
|
||||
}
|
||||
|
||||
CIMGUI_API void* ImGuiStorage_GetVoidPtr(struct ImGuiStorage* storage, ImGuiID key)
|
||||
{
|
||||
return storage->GetVoidPtr(key);
|
||||
return storage->GetVoidPtr(key);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiStorage_SetVoidPtr(struct ImGuiStorage* storage, ImGuiID key, void* val)
|
||||
{
|
||||
storage->SetVoidPtr(key, val);
|
||||
storage->SetVoidPtr(key, val);
|
||||
}
|
||||
|
||||
CIMGUI_API int* ImGuiStorage_GetIntRef(struct ImGuiStorage* storage, ImGuiID key, int default_val)
|
||||
{
|
||||
return storage->GetIntRef(key, default_val);
|
||||
return storage->GetIntRef(key, default_val);
|
||||
}
|
||||
|
||||
CIMGUI_API bool* ImGuiStorage_GetBoolRef(struct ImGuiStorage* storage, ImGuiID key, bool default_val)
|
||||
{
|
||||
return storage->GetBoolRef(key, default_val);
|
||||
return storage->GetBoolRef(key, default_val);
|
||||
}
|
||||
|
||||
CIMGUI_API float* ImGuiStorage_GetFloatRef(struct ImGuiStorage* storage, ImGuiID key, float default_val)
|
||||
{
|
||||
return storage->GetFloatRef(key, default_val);
|
||||
return storage->GetFloatRef(key, default_val);
|
||||
}
|
||||
|
||||
CIMGUI_API void** ImGuiStorage_GetVoidPtrRef(struct ImGuiStorage* storage, ImGuiID key, void* default_val)
|
||||
{
|
||||
return storage->GetVoidPtrRef(key, default_val);
|
||||
return storage->GetVoidPtrRef(key, default_val);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiStorage_SetAllInt(struct ImGuiStorage* storage, int val)
|
||||
{
|
||||
storage->SetAllInt(val);
|
||||
storage->SetAllInt(val);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextEditCallbackData_DeleteChars(struct ImGuiTextEditCallbackData* data, int pos, int bytes_count)
|
||||
{
|
||||
data->DeleteChars(pos, bytes_count);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImGuiTextEditCallbackData_InsertChars(struct ImGuiTextEditCallbackData* data, int pos, const char* text, const char* text_end)
|
||||
{
|
||||
data->InsertChars(pos, text, text_end);
|
||||
}
|
||||
|
||||
CIMGUI_API bool ImGuiTextEditCallbackData_HasSelection(struct ImGuiTextEditCallbackData* data)
|
||||
{
|
||||
return data->HasSelection();
|
||||
}
|
||||
|
|
|
@ -7,7 +7,7 @@
|
|||
#else
|
||||
#define API __declspec(dllexport)
|
||||
#endif
|
||||
#ifdef __GNUC__
|
||||
#ifndef __GNUC__
|
||||
#define snprintf sprintf_s
|
||||
#endif
|
||||
#else
|
||||
|
@ -37,6 +37,7 @@ struct ImGuiStorage;
|
|||
struct ImFont;
|
||||
struct ImFontConfig;
|
||||
struct ImFontAtlas;
|
||||
struct ImFontGlyph;
|
||||
struct ImDrawCmd;
|
||||
struct ImGuiListClipper;
|
||||
struct ImGuiTextFilter;
|
||||
|
@ -93,7 +94,10 @@ enum {
|
|||
ImGuiWindowFlags_AlwaysVerticalScrollbar = 1 << 14,
|
||||
ImGuiWindowFlags_AlwaysHorizontalScrollbar = 1 << 15,
|
||||
ImGuiWindowFlags_AlwaysUseWindowPadding = 1 << 16,
|
||||
ImGuiWindowFlags_NavFlattened = 1 << 19,
|
||||
ImGuiWindowFlags_NoNavFocus = 1 << 17,
|
||||
ImGuiWindowFlags_NoNavInputs = 1 << 18,
|
||||
ImGuiWindowFlags_NavFlattened = 1 << 19,
|
||||
ImGuiWindowFlags_NoNavScroll = 1 << 20,
|
||||
};
|
||||
|
||||
enum {
|
||||
|
@ -202,6 +206,8 @@ enum {
|
|||
ImGuiCol_PlotHistogramHovered,
|
||||
ImGuiCol_TextSelectedBg,
|
||||
ImGuiCol_ModalWindowDarkening,
|
||||
ImGuiCol_NavHighlight,
|
||||
ImGuiCol_NavWindowingHighlight,
|
||||
ImGuiCol_COUNT
|
||||
};
|
||||
|
||||
|
@ -510,6 +516,8 @@ CIMGUI_API void igPushAllowKeyboardFocus(bool v);
|
|||
CIMGUI_API void igPopAllowKeyboardFocus();
|
||||
CIMGUI_API void igPushButtonRepeat(bool repeat);
|
||||
CIMGUI_API void igPopButtonRepeat();
|
||||
CIMGUI_API void igPushNavDefaultFocus(bool default_focus);
|
||||
CIMGUI_API void igPopNavDefaultFocus();
|
||||
|
||||
// Layout
|
||||
CIMGUI_API void igSeparator();
|
||||
|
@ -548,14 +556,14 @@ CIMGUI_API int igGetColumnsCount();
|
|||
// ID scopes
|
||||
// If you are creating widgets in a loop you most likely want to push a unique identifier so ImGui can differentiate them
|
||||
// You can also use "##extra" within your widget name to distinguish them from each others (see 'Programmer Guide')
|
||||
CIMGUI_API void igPushIdStr(CONST char* str_id);
|
||||
CIMGUI_API void igPushIdStrRange(CONST char* str_begin, CONST char* str_end);
|
||||
CIMGUI_API void igPushIdPtr(CONST void* ptr_id);
|
||||
CIMGUI_API void igPushIdInt(int int_id);
|
||||
CIMGUI_API void igPopId();
|
||||
CIMGUI_API ImGuiID igGetIdStr(CONST char* str_id);
|
||||
CIMGUI_API ImGuiID igGetIdStrRange(CONST char* str_begin,CONST char* str_end);
|
||||
CIMGUI_API ImGuiID igGetIdPtr(CONST void* ptr_id);
|
||||
CIMGUI_API void igPushIDStr(CONST char* str_id);
|
||||
CIMGUI_API void igPushIDStrRange(CONST char* str_begin, CONST char* str_end);
|
||||
CIMGUI_API void igPushIDPtr(CONST void* ptr_id);
|
||||
CIMGUI_API void igPushIDInt(int int_id);
|
||||
CIMGUI_API void igPopID();
|
||||
CIMGUI_API ImGuiID igGetIDStr(CONST char* str_id);
|
||||
CIMGUI_API ImGuiID igGetIDStrRange(CONST char* str_begin,CONST char* str_end);
|
||||
CIMGUI_API ImGuiID igGetIDPtr(CONST void* ptr_id);
|
||||
|
||||
// Widgets
|
||||
CIMGUI_API void igText(CONST char* fmt, ...);
|
||||
|
@ -714,22 +722,18 @@ CIMGUI_API void igPopClipRect();
|
|||
|
||||
// Utilities
|
||||
CIMGUI_API bool igIsItemHovered();
|
||||
CIMGUI_API bool igIsItemRectHovered();
|
||||
CIMGUI_API bool igIsItemActive();
|
||||
CIMGUI_API bool igIsItemFocused();
|
||||
CIMGUI_API bool igIsItemClicked(int mouse_button);
|
||||
CIMGUI_API bool igIsItemVisible();
|
||||
CIMGUI_API bool igIsAnyItemHovered();
|
||||
CIMGUI_API bool igIsAnyItemActive();
|
||||
CIMGUI_API bool igIsAnyItemFocused();
|
||||
CIMGUI_API void igGetItemRectMin(struct ImVec2* pOut);
|
||||
CIMGUI_API void igGetItemRectMax(struct ImVec2* pOut);
|
||||
CIMGUI_API void igGetItemRectSize(struct ImVec2* pOut);
|
||||
CIMGUI_API void igSetItemAllowOverlap();
|
||||
CIMGUI_API void igSetItemDefaultFocus();
|
||||
CIMGUI_API bool igIsWindowFocused();
|
||||
CIMGUI_API bool igIsWindowHovered();
|
||||
CIMGUI_API bool igIsWindowRectHovered();
|
||||
CIMGUI_API bool igIsRootWindowFocused();
|
||||
CIMGUI_API bool igIsRootWindowOrAnyChildFocused();
|
||||
CIMGUI_API bool igIsRootWindowOrAnyChildHovered();
|
||||
|
@ -755,7 +759,6 @@ CIMGUI_API int igGetKeyIndex(ImGuiKey imgui_key);
|
|||
CIMGUI_API bool igIsKeyDown(int user_key_index);
|
||||
CIMGUI_API bool igIsKeyPressed(int user_key_index, bool repeat);
|
||||
CIMGUI_API bool igIsKeyReleased(int user_key_index);
|
||||
CIMGUI_API int igGetKeyPressedAmount(int key_index, float repeat_delay, float rate);
|
||||
CIMGUI_API bool igIsMouseDown(int button);
|
||||
CIMGUI_API bool igIsMouseClicked(int button, bool repeat);
|
||||
CIMGUI_API bool igIsMouseDoubleClicked(int button);
|
||||
|
@ -778,47 +781,76 @@ CIMGUI_API CONST char* igGetClipboardText();
|
|||
CIMGUI_API void igSetClipboardText(CONST char* text);
|
||||
|
||||
// Internal state access - if you want to share ImGui state between modules (e.g. DLL) or allocate it yourself
|
||||
CIMGUI_API CONST char* igGetVersion();
|
||||
CIMGUI_API struct ImGuiContext* igCreateContext(void* (*malloc_fn)(size_t), void (*free_fn)(void*));
|
||||
CIMGUI_API void igDestroyContext(struct ImGuiContext* ctx);
|
||||
CIMGUI_API struct ImGuiContext* igGetCurrentContext();
|
||||
CIMGUI_API void igSetCurrentContext(struct ImGuiContext* ctx);
|
||||
CIMGUI_API CONST char* igGetVersion();
|
||||
CIMGUI_API struct ImGuiContext* igCreateContext(void* (*malloc_fn)(size_t), void (*free_fn)(void*));
|
||||
CIMGUI_API void igDestroyContext(struct ImGuiContext* ctx);
|
||||
CIMGUI_API struct ImGuiContext* igGetCurrentContext();
|
||||
CIMGUI_API void igSetCurrentContext(struct ImGuiContext* ctx);
|
||||
|
||||
CIMGUI_API void ImFontConfig_DefaultConstructor(struct ImFontConfig* config);
|
||||
// ImGuiIO
|
||||
CIMGUI_API void ImGuiIO_AddInputCharacter(unsigned short c);
|
||||
CIMGUI_API void ImGuiIO_AddInputCharactersUTF8(CONST char* utf8_chars);
|
||||
CIMGUI_API void ImGuiIO_ClearInputCharacters();
|
||||
|
||||
CIMGUI_API void ImFontAtlas_GetTexDataAsRGBA32(struct ImFontAtlas* atlas, unsigned char** out_pixels, int* out_width, int* out_height, int* out_bytes_per_pixel);
|
||||
CIMGUI_API void ImFontAtlas_GetTexDataAsAlpha8(struct ImFontAtlas* atlas, unsigned char** out_pixels, int* out_width, int* out_height, int* out_bytes_per_pixel);
|
||||
CIMGUI_API void ImFontAtlas_SetTexID(struct ImFontAtlas* atlas, ImTextureID id);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFont(struct ImFontAtlas* atlas, CONST struct ImFontConfig* font_cfg);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontDefault(struct ImFontAtlas* atlas, CONST struct ImFontConfig* font_cfg);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontFromFileTTF(struct ImFontAtlas* atlas, CONST char* filename, float size_pixels, CONST struct ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontFromMemoryTTF(struct ImFontAtlas* atlas, void* font_data, int font_size, float size_pixels, CONST struct ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontFromMemoryCompressedTTF(struct ImFontAtlas* atlas, CONST void* compressed_font_data, int compressed_font_size, float size_pixels, CONST struct ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontFromMemoryCompressedBase85TTF(struct ImFontAtlas* atlas, CONST char* compressed_font_data_base85, float size_pixels, CONST struct ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges);
|
||||
CIMGUI_API void ImFontAtlas_ClearTexData(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API void ImFontAtlas_Clear(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesDefault(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesKorean(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesJapanese(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesChinese(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesCyrillic(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesThai(struct ImFontAtlas* atlas);
|
||||
// ImGuiTextFilter
|
||||
CIMGUI_API struct ImGuiTextFilter* ImGuiTextFilter_Create(const char* default_filter);
|
||||
CIMGUI_API void ImGuiTextFilter_Destroy(struct ImGuiTextFilter* filter);
|
||||
CIMGUI_API void ImGuiTextFilter_Clear(struct ImGuiTextFilter* filter);
|
||||
CIMGUI_API bool ImGuiTextFilter_Draw(struct ImGuiTextFilter* filter, const char* label, float width);
|
||||
CIMGUI_API bool ImGuiTextFilter_PassFilter(const struct ImGuiTextFilter* filter, const char* text, const char* text_end);
|
||||
CIMGUI_API bool ImGuiTextFilter_IsActive(const struct ImGuiTextFilter* filter);
|
||||
CIMGUI_API void ImGuiTextFilter_Build(struct ImGuiTextFilter* filter);
|
||||
CIMGUI_API const char* ImGuiTextFilter_GetInputBuf(struct ImGuiTextFilter* filter);
|
||||
|
||||
CIMGUI_API void ImGuiIO_AddInputCharacter(unsigned short c);
|
||||
CIMGUI_API void ImGuiIO_AddInputCharactersUTF8(CONST char* utf8_chars);
|
||||
CIMGUI_API void ImGuiIO_ClearInputCharacters();
|
||||
// ImGuiTextBuffer
|
||||
CIMGUI_API struct ImGuiTextBuffer* ImGuiTextBuffer_Create();
|
||||
CIMGUI_API void ImGuiTextBuffer_Destroy(struct ImGuiTextBuffer* buffer);
|
||||
CIMGUI_API char ImGuiTextBuffer_index(struct ImGuiTextBuffer* buffer, int i);
|
||||
CIMGUI_API const char* ImGuiTextBuffer_begin(const struct ImGuiTextBuffer* buffer);
|
||||
CIMGUI_API const char* ImGuiTextBuffer_end(const struct ImGuiTextBuffer* buffer);
|
||||
CIMGUI_API int ImGuiTextBuffer_size(const struct ImGuiTextBuffer* buffer);
|
||||
CIMGUI_API bool ImGuiTextBuffer_empty(struct ImGuiTextBuffer* buffer);
|
||||
CIMGUI_API void ImGuiTextBuffer_clear(struct ImGuiTextBuffer* buffer);
|
||||
CIMGUI_API const char* ImGuiTextBuffer_c_str(const struct ImGuiTextBuffer* buffer);
|
||||
CIMGUI_API void ImGuiTextBuffer_append(struct ImGuiTextBuffer* buffer, const char* fmt, ...);
|
||||
CIMGUI_API void ImGuiTextBuffer_appendv(struct ImGuiTextBuffer* buffer, const char* fmt, va_list args);
|
||||
|
||||
//ImDrawData
|
||||
CIMGUI_API void ImDrawData_DeIndexAllBuffers(struct ImDrawData* drawData);
|
||||
CIMGUI_API void ImDrawData_ScaleClipRects(struct ImDrawData* drawData, struct ImVec2 sc);
|
||||
// ImGuiStorage
|
||||
CIMGUI_API struct ImGuiStorage* ImGuiStorage_Create();
|
||||
CIMGUI_API void ImGuiStorage_Destroy(struct ImGuiStorage* storage);
|
||||
CIMGUI_API int ImGuiStorage_GetInt(struct ImGuiStorage* storage, ImGuiID key, int default_val);
|
||||
CIMGUI_API void ImGuiStorage_SetInt(struct ImGuiStorage* storage, ImGuiID key, int val);
|
||||
CIMGUI_API bool ImGuiStorage_GetBool(struct ImGuiStorage* storage, ImGuiID key, bool default_val);
|
||||
CIMGUI_API void ImGuiStorage_SetBool(struct ImGuiStorage* storage, ImGuiID key, bool val);
|
||||
CIMGUI_API float ImGuiStorage_GetFloat(struct ImGuiStorage* storage, ImGuiID key, float default_val);
|
||||
CIMGUI_API void ImGuiStorage_SetFloat(struct ImGuiStorage* storage, ImGuiID key, float val);
|
||||
CIMGUI_API void* ImGuiStorage_GetVoidPtr(struct ImGuiStorage* storage, ImGuiID key);
|
||||
CIMGUI_API void ImGuiStorage_SetVoidPtr(struct ImGuiStorage* storage, ImGuiID key, void* val);
|
||||
CIMGUI_API int* ImGuiStorage_GetIntRef(struct ImGuiStorage* storage, ImGuiID key, int default_val);
|
||||
CIMGUI_API bool* ImGuiStorage_GetBoolRef(struct ImGuiStorage* storage, ImGuiID key, bool default_val);
|
||||
CIMGUI_API float* ImGuiStorage_GetFloatRef(struct ImGuiStorage* storage, ImGuiID key, float default_val);
|
||||
CIMGUI_API void** ImGuiStorage_GetVoidPtrRef(struct ImGuiStorage* storage, ImGuiID key, void* default_val);
|
||||
CIMGUI_API void ImGuiStorage_SetAllInt(struct ImGuiStorage* storage, int val);
|
||||
|
||||
// ImGuiTextEditCallbackData
|
||||
CIMGUI_API void ImGuiTextEditCallbackData_DeleteChars(struct ImGuiTextEditCallbackData* data, int pos, int bytes_count);
|
||||
CIMGUI_API void ImGuiTextEditCallbackData_InsertChars(struct ImGuiTextEditCallbackData* data, int pos, const char* text, const char* text_end);
|
||||
CIMGUI_API bool ImGuiTextEditCallbackData_HasSelection(struct ImGuiTextEditCallbackData* data);
|
||||
|
||||
// ImGuiListClipper
|
||||
CIMGUI_API void ImGuiListClipper_Begin(struct ImGuiListClipper* clipper, int count, float items_height);
|
||||
CIMGUI_API void ImGuiListClipper_End(struct ImGuiListClipper* clipper);
|
||||
CIMGUI_API bool ImGuiListClipper_Step(struct ImGuiListClipper* clipper);
|
||||
CIMGUI_API int ImGuiListClipper_GetDisplayStart(struct ImGuiListClipper* clipper);
|
||||
CIMGUI_API int ImGuiListClipper_GetDisplayEnd(struct ImGuiListClipper* clipper);
|
||||
|
||||
//ImDrawList
|
||||
CIMGUI_API int ImDrawList_GetVertexBufferSize(struct ImDrawList* list);
|
||||
CIMGUI_API struct ImDrawVert* ImDrawList_GetVertexPtr(struct ImDrawList* list, int n);
|
||||
CIMGUI_API int ImDrawList_GetIndexBufferSize(struct ImDrawList* list);
|
||||
CIMGUI_API ImDrawIdx* ImDrawList_GetIndexPtr(struct ImDrawList* list, int n);
|
||||
CIMGUI_API int ImDrawList_GetCmdSize(struct ImDrawList* list);
|
||||
CIMGUI_API struct ImDrawCmd* ImDrawList_GetCmdPtr(struct ImDrawList* list, int n);
|
||||
CIMGUI_API int ImDrawList_GetVertexBufferSize(struct ImDrawList* list);
|
||||
CIMGUI_API struct ImDrawVert* ImDrawList_GetVertexPtr(struct ImDrawList* list, int n);
|
||||
CIMGUI_API int ImDrawList_GetIndexBufferSize(struct ImDrawList* list);
|
||||
CIMGUI_API ImDrawIdx* ImDrawList_GetIndexPtr(struct ImDrawList* list, int n);
|
||||
CIMGUI_API int ImDrawList_GetCmdSize(struct ImDrawList* list);
|
||||
CIMGUI_API struct ImDrawCmd* ImDrawList_GetCmdPtr(struct ImDrawList* list, int n);
|
||||
|
||||
CIMGUI_API void ImDrawList_Clear(struct ImDrawList* list);
|
||||
CIMGUI_API void ImDrawList_ClearFreeMemory(struct ImDrawList* list);
|
||||
|
@ -873,46 +905,83 @@ CIMGUI_API void ImDrawList_AddDrawCmd(struct ImDrawList* list); // T
|
|||
CIMGUI_API void ImDrawList_PrimReserve(struct ImDrawList* list, int idx_count, int vtx_count);
|
||||
CIMGUI_API void ImDrawList_PrimRect(struct ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, ImU32 col);
|
||||
CIMGUI_API void ImDrawList_PrimRectUV(struct ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 uv_a, CONST struct ImVec2 uv_b, ImU32 col);
|
||||
CIMGUI_API void ImDrawList_PrimQuadUV(struct ImDrawList* list,CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 c, CONST struct ImVec2 d, CONST struct ImVec2 uv_a, CONST struct ImVec2 uv_b, CONST struct ImVec2 uv_c, CONST struct ImVec2 uv_d, ImU32 col);
|
||||
CIMGUI_API void ImDrawList_PrimQuadUV(struct ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 c, CONST struct ImVec2 d, CONST struct ImVec2 uv_a, CONST struct ImVec2 uv_b, CONST struct ImVec2 uv_c, CONST struct ImVec2 uv_d, ImU32 col);
|
||||
CIMGUI_API void ImDrawList_PrimWriteVtx(struct ImDrawList* list, CONST struct ImVec2 pos, CONST struct ImVec2 uv, ImU32 col);
|
||||
CIMGUI_API void ImDrawList_PrimWriteIdx(struct ImDrawList* list, ImDrawIdx idx);
|
||||
CIMGUI_API void ImDrawList_PrimVtx(struct ImDrawList* list, CONST struct ImVec2 pos, CONST struct ImVec2 uv, ImU32 col);
|
||||
CIMGUI_API void ImDrawList_UpdateClipRect(struct ImDrawList* list);
|
||||
CIMGUI_API void ImDrawList_UpdateTextureID(struct ImDrawList* list);
|
||||
|
||||
// ImGuiListClipper
|
||||
CIMGUI_API void ImGuiListClipper_Begin(struct ImGuiListClipper* clipper, int count, float items_height);
|
||||
CIMGUI_API void ImGuiListClipper_End(struct ImGuiListClipper* clipper);
|
||||
CIMGUI_API bool ImGuiListClipper_Step(struct ImGuiListClipper* clipper);
|
||||
CIMGUI_API int ImGuiListClipper_GetDisplayStart(struct ImGuiListClipper* clipper);
|
||||
CIMGUI_API int ImGuiListClipper_GetDisplayEnd(struct ImGuiListClipper* clipper);
|
||||
// ImDrawData
|
||||
CIMGUI_API void ImDrawData_DeIndexAllBuffers(struct ImDrawData* drawData);
|
||||
CIMGUI_API void ImDrawData_ScaleClipRects(struct ImDrawData* drawData, const struct ImVec2 sc);
|
||||
|
||||
// ImGuiTextFilter
|
||||
CIMGUI_API void ImGuiTextFilter_Init(struct ImGuiTextFilter* filter, const char* default_filter);
|
||||
CIMGUI_API void ImGuiTextFilter_Clear(struct ImGuiTextFilter* filter);
|
||||
CIMGUI_API bool ImGuiTextFilter_Draw(struct ImGuiTextFilter* filter, const char* label, float width);
|
||||
CIMGUI_API bool ImGuiTextFilter_PassFilter(struct ImGuiTextFilter* filter, const char* text, const char* text_end);
|
||||
CIMGUI_API bool ImGuiTextFilter_IsActive(struct ImGuiTextFilter* filter);
|
||||
CIMGUI_API void ImGuiTextFilter_Build(struct ImGuiTextFilter* filter);
|
||||
// ImFontAtlas
|
||||
CIMGUI_API void ImFontAtlas_GetTexDataAsRGBA32(struct ImFontAtlas* atlas, unsigned char** out_pixels, int* out_width, int* out_height, int* out_bytes_per_pixel);
|
||||
CIMGUI_API void ImFontAtlas_GetTexDataAsAlpha8(struct ImFontAtlas* atlas, unsigned char** out_pixels, int* out_width, int* out_height, int* out_bytes_per_pixel);
|
||||
CIMGUI_API void ImFontAtlas_SetTexID(struct ImFontAtlas* atlas, ImTextureID id);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFont(struct ImFontAtlas* atlas, CONST struct ImFontConfig* font_cfg);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontDefault(struct ImFontAtlas* atlas, CONST struct ImFontConfig* font_cfg);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontFromFileTTF(struct ImFontAtlas* atlas, CONST char* filename, float size_pixels, CONST struct ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontFromMemoryTTF(struct ImFontAtlas* atlas, void* font_data, int font_size, float size_pixels, CONST struct ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontFromMemoryCompressedTTF(struct ImFontAtlas* atlas, CONST void* compressed_font_data, int compressed_font_size, float size_pixels, CONST struct ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_AddFontFromMemoryCompressedBase85TTF(struct ImFontAtlas* atlas, CONST char* compressed_font_data_base85, float size_pixels, CONST struct ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges);
|
||||
CIMGUI_API void ImFontAtlas_ClearTexData(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API void ImFontAtlas_Clear(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesDefault(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesKorean(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesJapanese(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesChinese(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesCyrillic(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesThai(struct ImFontAtlas* atlas);
|
||||
|
||||
// ImGuiTextEditCallbackData
|
||||
CIMGUI_API void ImGuiTextEditCallbackData_DeleteChars(struct ImGuiTextEditCallbackData* data, int pos, int bytes_count);
|
||||
CIMGUI_API void ImGuiTextEditCallbackData_InsertChars(struct ImGuiTextEditCallbackData* data, int pos, const char* text, const char* text_end);
|
||||
CIMGUI_API bool ImGuiTextEditCallbackData_HasSelection(struct ImGuiTextEditCallbackData* data);
|
||||
CIMGUI_API ImTextureID ImFontAtlas_GetTexID(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API unsigned char* ImFontAtlas_GetTexPixelsAlpha8(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API unsigned int* ImFontAtlas_GetTexPixelsRGBA32(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API int ImFontAtlas_GetTexWidth(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API int ImFontAtlas_GetTexHeight(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API int ImFontAtlas_GetTexDesiredWidth(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API void ImFontAtlas_SetTexDesiredWidth(struct ImFontAtlas* atlas, int TexDesiredWidth_);
|
||||
CIMGUI_API int ImFontAtlas_GetTexGlyphPadding(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API void ImFontAtlas_SetTexGlyphPadding(struct ImFontAtlas* atlas, int TexGlyphPadding_);
|
||||
CIMGUI_API void ImFontAtlas_GetTexUvWhitePixel(struct ImFontAtlas* atlas, struct ImVec2* pOut);
|
||||
|
||||
// ImGuiStorage
|
||||
CIMGUI_API void ImGuiStorage_Init(struct ImGuiStorage* storage);
|
||||
CIMGUI_API void ImGuiStorage_Clear(struct ImGuiStorage* storage);
|
||||
CIMGUI_API int ImGuiStorage_GetInt(struct ImGuiStorage* storage, ImGuiID key, int default_val);
|
||||
CIMGUI_API void ImGuiStorage_SetInt(struct ImGuiStorage* storage, ImGuiID key, int val);
|
||||
CIMGUI_API bool ImGuiStorage_GetBool(struct ImGuiStorage* storage, ImGuiID key, bool default_val);
|
||||
CIMGUI_API void ImGuiStorage_SetBool(struct ImGuiStorage* storage, ImGuiID key, bool val);
|
||||
CIMGUI_API float ImGuiStorage_GetFloat(struct ImGuiStorage* storage, ImGuiID key, float default_val);
|
||||
CIMGUI_API void ImGuiStorage_SetFloat(struct ImGuiStorage* storage, ImGuiID key, float val);
|
||||
CIMGUI_API void* ImGuiStorage_GetVoidPtr(struct ImGuiStorage* storage, ImGuiID key);
|
||||
CIMGUI_API void ImGuiStorage_SetVoidPtr(struct ImGuiStorage* storage, ImGuiID key, void* val);
|
||||
CIMGUI_API int* ImGuiStorage_GetIntRef(struct ImGuiStorage* storage, ImGuiID key, int default_val);
|
||||
CIMGUI_API bool* ImGuiStorage_GetBoolRef(struct ImGuiStorage* storage, ImGuiID key, bool default_val);
|
||||
CIMGUI_API float* ImGuiStorage_GetFloatRef(struct ImGuiStorage* storage, ImGuiID key, float default_val);
|
||||
CIMGUI_API void** ImGuiStorage_GetVoidPtrRef(struct ImGuiStorage* storage, ImGuiID key, void* default_val);
|
||||
CIMGUI_API void ImGuiStorage_SetAllInt(struct ImGuiStorage* storage, int val);
|
||||
// ImFontAtlas::Fonts;
|
||||
CIMGUI_API int ImFontAtlas_Fonts_size(struct ImFontAtlas* atlas);
|
||||
CIMGUI_API struct ImFont* ImFontAtlas_Fonts_index(struct ImFontAtlas* atlas, int index);
|
||||
|
||||
// ImFont
|
||||
CIMGUI_API float ImFont_GetFontSize(const struct ImFont* font);
|
||||
CIMGUI_API void ImFont_SetFontSize(struct ImFont* font, float FontSize_);
|
||||
CIMGUI_API float ImFont_GetScale(const struct ImFont* font);
|
||||
CIMGUI_API void ImFont_SetScale(struct ImFont* font, float Scale_);
|
||||
CIMGUI_API void ImFont_GetDisplayOffset(const struct ImFont* font, struct ImVec2* pOut);
|
||||
CIMGUI_API const struct ImFontGlyph* ImFont_GetFallbackGlyph(const struct ImFont* font);
|
||||
CIMGUI_API void ImFont_SetFallbackGlyph(struct ImFont* font, const struct ImFontGlyph* FallbackGlyph_);
|
||||
CIMGUI_API float ImFont_GetFallbackAdvanceX(const struct ImFont* font);
|
||||
CIMGUI_API ImWchar ImFont_GetFallbackChar(const struct ImFont* font);
|
||||
CIMGUI_API short ImFont_GetConfigDataCount(const struct ImFont* font);
|
||||
CIMGUI_API struct ImFontConfig* ImFont_GetConfigData(struct ImFont* font);
|
||||
CIMGUI_API struct ImFontAtlas* ImFont_GetContainerAtlas(struct ImFont* font);
|
||||
CIMGUI_API float ImFont_GetAscent(const struct ImFont* font);
|
||||
CIMGUI_API float ImFont_GetDescent(const struct ImFont* font);
|
||||
CIMGUI_API int ImFont_GetMetricsTotalSurface(const struct ImFont* font);
|
||||
CIMGUI_API void ImFont_ClearOutputData(struct ImFont* font);
|
||||
CIMGUI_API void ImFont_BuildLookupTable(struct ImFont* font);
|
||||
CIMGUI_API const struct ImFontGlyph* ImFont_FindGlyph(const struct ImFont* font, ImWchar c);
|
||||
CIMGUI_API void ImFont_SetFallbackChar(struct ImFont* font, ImWchar c);
|
||||
CIMGUI_API float ImFont_GetCharAdvance(const struct ImFont* font, ImWchar c);
|
||||
CIMGUI_API bool ImFont_IsLoaded(const struct ImFont* font);
|
||||
CIMGUI_API void ImFont_CalcTextSizeA(const struct ImFont* font, struct ImVec2* pOut, float size, float max_width, float wrap_width, const char* text_begin, const char* text_end, const char** remaining); // utf8
|
||||
CIMGUI_API const char* ImFont_CalcWordWrapPositionA(const struct ImFont* font, float scale, const char* text, const char* text_end, float wrap_width);
|
||||
CIMGUI_API void ImFont_RenderChar(const struct ImFont* font, struct ImDrawList* draw_list, float size, struct ImVec2 pos, ImU32 col, unsigned short c);
|
||||
CIMGUI_API void ImFont_RenderText(const struct ImFont* font, struct ImDrawList* draw_list, float size, struct ImVec2 pos, ImU32 col, const struct ImVec4* clip_rect, const char* text_begin, const char* text_end, float wrap_width, bool cpu_fine_clip);
|
||||
// ImFont::Glyph
|
||||
CIMGUI_API int ImFont_Glyphs_size(const struct ImFont* font);
|
||||
CIMGUI_API struct ImFontGlyph* ImFont_Glyphs_index(struct ImFont* font, int index);
|
||||
// ImFont::IndexAdvanceX
|
||||
CIMGUI_API int ImFont_IndexAdvanceX_size(const struct ImFont* font);
|
||||
CIMGUI_API float ImFont_IndexAdvanceX_index(const struct ImFont* font, int index);
|
||||
// ImFont::IndexLookup
|
||||
CIMGUI_API int ImFont_IndexLookup_size(const struct ImFont* font);
|
||||
CIMGUI_API unsigned short ImFont_IndexLookup_index(const struct ImFont* font, int index);
|
||||
|
|
|
@ -2,287 +2,287 @@
|
|||
#include "../imgui/imgui.h"
|
||||
#include "cimgui.h"
|
||||
|
||||
CIMGUI_API void ImDrawData_DeIndexAllBuffers(ImDrawData* drawData)
|
||||
{
|
||||
return drawData->DeIndexAllBuffers();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawData_ScaleClipRects(ImDrawData* drawData, const struct ImVec2 sc)
|
||||
{
|
||||
return drawData->ScaleClipRects(sc);
|
||||
}
|
||||
|
||||
CIMGUI_API int ImDrawList_GetVertexBufferSize(ImDrawList* list)
|
||||
{
|
||||
return list->VtxBuffer.size();
|
||||
return list->VtxBuffer.size();
|
||||
}
|
||||
|
||||
CIMGUI_API ImDrawVert* ImDrawList_GetVertexPtr(ImDrawList* list, int n)
|
||||
{
|
||||
return &list->VtxBuffer[n];
|
||||
return &list->VtxBuffer[n];
|
||||
}
|
||||
|
||||
CIMGUI_API int ImDrawList_GetIndexBufferSize(ImDrawList* list)
|
||||
{
|
||||
return list->IdxBuffer.size();
|
||||
return list->IdxBuffer.size();
|
||||
}
|
||||
|
||||
CIMGUI_API ImDrawIdx* ImDrawList_GetIndexPtr(ImDrawList* list, int n)
|
||||
{
|
||||
return &list->IdxBuffer[n];
|
||||
return &list->IdxBuffer[n];
|
||||
}
|
||||
|
||||
CIMGUI_API int ImDrawList_GetCmdSize(ImDrawList* list)
|
||||
{
|
||||
return list->CmdBuffer.size();
|
||||
return list->CmdBuffer.size();
|
||||
}
|
||||
|
||||
CIMGUI_API ImDrawCmd* ImDrawList_GetCmdPtr(ImDrawList* list, int n)
|
||||
{
|
||||
return &list->CmdBuffer[n];
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawData_DeIndexAllBuffers(ImDrawData* drawData)
|
||||
{
|
||||
return drawData->DeIndexAllBuffers();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawData_ScaleClipRects(ImDrawData* drawData, struct ImVec2 sc)
|
||||
{
|
||||
return drawData->ScaleClipRects(sc);
|
||||
return &list->CmdBuffer[n];
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_Clear(ImDrawList* list)
|
||||
{
|
||||
return list->Clear();
|
||||
return list->Clear();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_ClearFreeMemory(ImDrawList* list)
|
||||
{
|
||||
return list->ClearFreeMemory();
|
||||
return list->ClearFreeMemory();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PushClipRect(ImDrawList* list, struct ImVec2 clip_rect_min, struct ImVec2 clip_rect_max, bool intersect_with_current_clip_rect)
|
||||
{
|
||||
return list->PushClipRect(clip_rect_min,clip_rect_max,intersect_with_current_clip_rect);
|
||||
return list->PushClipRect(clip_rect_min,clip_rect_max,intersect_with_current_clip_rect);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PushClipRectFullScreen(ImDrawList* list)
|
||||
{
|
||||
return list->PushClipRectFullScreen();
|
||||
return list->PushClipRectFullScreen();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PopClipRect(ImDrawList* list)
|
||||
{
|
||||
return list->PopClipRect();
|
||||
return list->PopClipRect();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PushTextureID(ImDrawList* list, CONST ImTextureID texture_id)
|
||||
{
|
||||
return list->PushTextureID(texture_id);
|
||||
return list->PushTextureID(texture_id);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PopTextureID(ImDrawList* list)
|
||||
{
|
||||
return list->PopTextureID();
|
||||
return list->PopTextureID();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_GetClipRectMin(ImVec2* pOut, ImDrawList* list)
|
||||
{
|
||||
*pOut = list->GetClipRectMin();
|
||||
*pOut = list->GetClipRectMin();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_GetClipRectMax(ImVec2* pOut, ImDrawList* list)
|
||||
{
|
||||
*pOut = list->GetClipRectMax();
|
||||
*pOut = list->GetClipRectMax();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddLine(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, ImU32 col, float thickness)
|
||||
{
|
||||
return list->AddLine(a, b, col, thickness);
|
||||
return list->AddLine(a, b, col, thickness);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddRect(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, ImU32 col, float rounding, int rounding_corners_flags, float thickness)
|
||||
{
|
||||
return list->AddRect(a, b, col, rounding, rounding_corners_flags, thickness);
|
||||
return list->AddRect(a, b, col, rounding, rounding_corners_flags, thickness);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddRectFilled(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, ImU32 col, float rounding, int rounding_corners_flags)
|
||||
{
|
||||
return list->AddRectFilled(a, b, col, rounding, rounding_corners_flags);
|
||||
return list->AddRectFilled(a, b, col, rounding, rounding_corners_flags);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddRectFilledMultiColor(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, ImU32 col_upr_left, ImU32 col_upr_right, ImU32 col_bot_right, ImU32 col_bot_left)
|
||||
{
|
||||
return list->AddRectFilledMultiColor(a, b, col_upr_left, col_upr_right, col_bot_right, col_bot_left);
|
||||
return list->AddRectFilledMultiColor(a, b, col_upr_left, col_upr_right, col_bot_right, col_bot_left);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddQuad(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 c, CONST struct ImVec2 d, ImU32 col, float thickness)
|
||||
{
|
||||
return list->AddQuad(a, b, c, d, col, thickness);
|
||||
return list->AddQuad(a, b, c, d, col, thickness);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddQuadFilled(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 c, CONST struct ImVec2 d, ImU32 col)
|
||||
{
|
||||
return list->AddQuadFilled(a, b, c, d, col);
|
||||
return list->AddQuadFilled(a, b, c, d, col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddTriangle(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 c, ImU32 col, float thickness)
|
||||
{
|
||||
return list->AddTriangle(a,b,c,col,thickness);
|
||||
return list->AddTriangle(a,b,c,col,thickness);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddTriangleFilled(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 c, ImU32 col)
|
||||
{
|
||||
return list->AddTriangleFilled(a, b, c, col);
|
||||
return list->AddTriangleFilled(a, b, c, col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddCircle(ImDrawList* list, CONST struct ImVec2 centre, float radius, ImU32 col, int num_segments, float thickness)
|
||||
{
|
||||
return list->AddCircle(centre, radius, col, num_segments, thickness);
|
||||
return list->AddCircle(centre, radius, col, num_segments, thickness);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddCircleFilled(ImDrawList* list, CONST struct ImVec2 centre, float radius, ImU32 col, int num_segments)
|
||||
{
|
||||
return list->AddCircleFilled(centre, radius, col, num_segments);
|
||||
return list->AddCircleFilled(centre, radius, col, num_segments);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddText(ImDrawList* list, CONST struct ImVec2 pos, ImU32 col, CONST char* text_begin, CONST char* text_end)
|
||||
{
|
||||
return list->AddText(pos, col, text_begin, text_end);
|
||||
return list->AddText(pos, col, text_begin, text_end);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddTextExt(ImDrawList* list, CONST ImFont* font, float font_size, CONST struct ImVec2 pos, ImU32 col, CONST char* text_begin, CONST char* text_end, float wrap_width, CONST ImVec4* cpu_fine_clip_rect)
|
||||
{
|
||||
return list->AddText(font, font_size, pos, col, text_begin, text_end, wrap_width, cpu_fine_clip_rect);
|
||||
return list->AddText(font, font_size, pos, col, text_begin, text_end, wrap_width, cpu_fine_clip_rect);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddImage(ImDrawList* list, ImTextureID user_texture_id, CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 uv_a, CONST struct ImVec2 uv_b, ImU32 col)
|
||||
{
|
||||
return list->AddImage(user_texture_id, a, b, uv_a, uv_b, col);
|
||||
return list->AddImage(user_texture_id, a, b, uv_a, uv_b, col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddImageQuad(struct ImDrawList* list, ImTextureID user_texture_id, CONST struct ImVec2 a, CONST ImVec2 b, CONST ImVec2 c, CONST ImVec2 d, CONST ImVec2 uv_a, CONST ImVec2 uv_b, CONST ImVec2 uv_c, CONST ImVec2 uv_d, ImU32 col)
|
||||
{
|
||||
return list->AddImageQuad(user_texture_id, a, b, c, d, uv_a, uv_b, uv_c, uv_d, col);
|
||||
return list->AddImageQuad(user_texture_id, a, b, c, d, uv_a, uv_b, uv_c, uv_d, col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddPolyline(ImDrawList* list, CONST ImVec2* points, CONST int num_points, ImU32 col, bool closed, float thickness, bool anti_aliased)
|
||||
{
|
||||
return list->AddPolyline(points, num_points, col, closed, thickness, anti_aliased);
|
||||
return list->AddPolyline(points, num_points, col, closed, thickness, anti_aliased);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddConvexPolyFilled(ImDrawList* list, CONST ImVec2* points, CONST int num_points, ImU32 col, bool anti_aliased)
|
||||
{
|
||||
return list->AddConvexPolyFilled(points, num_points, col, anti_aliased);
|
||||
return list->AddConvexPolyFilled(points, num_points, col, anti_aliased);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddBezierCurve(ImDrawList* list, CONST struct ImVec2 pos0, CONST struct ImVec2 cp0, CONST struct ImVec2 cp1, CONST struct ImVec2 pos1, ImU32 col, float thickness, int num_segments)
|
||||
{
|
||||
return list->AddBezierCurve(pos0, cp0, cp1, pos1, col, thickness, num_segments);
|
||||
return list->AddBezierCurve(pos0, cp0, cp1, pos1, col, thickness, num_segments);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PathClear(ImDrawList* list)
|
||||
{
|
||||
return list->PathClear();
|
||||
return list->PathClear();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PathLineTo(ImDrawList* list, CONST struct ImVec2 pos)
|
||||
{
|
||||
return list->PathLineTo(pos);
|
||||
return list->PathLineTo(pos);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PathLineToMergeDuplicate(ImDrawList* list, CONST struct ImVec2 pos)
|
||||
{
|
||||
return list->PathLineToMergeDuplicate(pos);
|
||||
return list->PathLineToMergeDuplicate(pos);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PathFillConvex(ImDrawList* list, ImU32 col)
|
||||
{
|
||||
return list->PathFillConvex(col);
|
||||
return list->PathFillConvex(col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PathStroke(ImDrawList* list, ImU32 col, bool closed, float thickness)
|
||||
{
|
||||
return list->PathStroke(col, closed, thickness);
|
||||
return list->PathStroke(col, closed, thickness);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PathArcTo(ImDrawList* list, CONST struct ImVec2 centre, float radius, float a_min, float a_max, int num_segments)
|
||||
{
|
||||
return list->PathArcTo(centre, radius, a_min, a_max, num_segments);
|
||||
return list->PathArcTo(centre, radius, a_min, a_max, num_segments);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PathArcToFast(ImDrawList* list, CONST struct ImVec2 centre, float radius, int a_min_of_12, int a_max_of_12)
|
||||
{
|
||||
return list->PathArcToFast(centre, radius, a_min_of_12, a_max_of_12);
|
||||
return list->PathArcToFast(centre, radius, a_min_of_12, a_max_of_12);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PathBezierCurveTo(ImDrawList* list, CONST struct ImVec2 p1, CONST struct ImVec2 p2, CONST struct ImVec2 p3, int num_segments)
|
||||
{
|
||||
return list->PathBezierCurveTo(p1, p2, p3, num_segments);
|
||||
return list->PathBezierCurveTo(p1, p2, p3, num_segments);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PathRect(ImDrawList* list, CONST struct ImVec2 rect_min, CONST struct ImVec2 rect_max, float rounding, int rounding_corners_flags)
|
||||
{
|
||||
return list->PathRect(rect_min, rect_max, rounding, rounding_corners_flags);
|
||||
return list->PathRect(rect_min, rect_max, rounding, rounding_corners_flags);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_ChannelsSplit(ImDrawList* list, int channels_count)
|
||||
{
|
||||
return list->ChannelsSplit(channels_count);
|
||||
return list->ChannelsSplit(channels_count);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_ChannelsMerge(ImDrawList* list)
|
||||
{
|
||||
return list->ChannelsMerge();
|
||||
return list->ChannelsMerge();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_ChannelsSetCurrent(ImDrawList* list, int channel_index)
|
||||
{
|
||||
return list->ChannelsSetCurrent(channel_index);
|
||||
return list->ChannelsSetCurrent(channel_index);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddCallback(ImDrawList* list, ImDrawCallback callback, void* callback_data)
|
||||
{
|
||||
return list->AddCallback(callback, callback_data);
|
||||
return list->AddCallback(callback, callback_data);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_AddDrawCmd(ImDrawList* list)
|
||||
{
|
||||
return list->AddDrawCmd();
|
||||
return list->AddDrawCmd();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PrimReserve(ImDrawList* list, int idx_count, int vtx_count)
|
||||
{
|
||||
return list->PrimReserve(idx_count, vtx_count);
|
||||
return list->PrimReserve(idx_count, vtx_count);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PrimRect(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, ImU32 col)
|
||||
{
|
||||
return list->PrimRect(a, b, col);
|
||||
return list->PrimRect(a, b, col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PrimRectUV(ImDrawList* list, CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 uv_a, CONST struct ImVec2 uv_b, ImU32 col)
|
||||
{
|
||||
return list->PrimRectUV(a, b, uv_a, uv_b, col);
|
||||
return list->PrimRectUV(a, b, uv_a, uv_b, col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PrimQuadUV(ImDrawList* list,CONST struct ImVec2 a, CONST struct ImVec2 b, CONST struct ImVec2 c, CONST struct ImVec2 d, CONST struct ImVec2 uv_a, CONST struct ImVec2 uv_b, CONST struct ImVec2 uv_c, CONST struct ImVec2 uv_d, ImU32 col)
|
||||
{
|
||||
return list->PrimQuadUV(a,b,c,d,uv_a,uv_b,uv_c,uv_d,col);
|
||||
return list->PrimQuadUV(a,b,c,d,uv_a,uv_b,uv_c,uv_d,col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PrimVtx(ImDrawList* list, CONST struct ImVec2 pos, CONST struct ImVec2 uv, ImU32 col)
|
||||
{
|
||||
return list->PrimVtx(pos, uv, col);
|
||||
return list->PrimVtx(pos, uv, col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PrimWriteVtx(ImDrawList* list, CONST struct ImVec2 pos, CONST struct ImVec2 uv, ImU32 col)
|
||||
{
|
||||
return list->PrimWriteVtx(pos, uv, col);
|
||||
return list->PrimWriteVtx(pos, uv, col);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_PrimWriteIdx(ImDrawList* list, ImDrawIdx idx)
|
||||
{
|
||||
return list->PrimWriteIdx(idx);
|
||||
return list->PrimWriteIdx(idx);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_UpdateClipRect(ImDrawList* list)
|
||||
{
|
||||
return list->UpdateClipRect();
|
||||
return list->UpdateClipRect();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImDrawList_UpdateTextureID(ImDrawList* list)
|
||||
{
|
||||
return list->UpdateTextureID();
|
||||
return list->UpdateTextureID();
|
||||
}
|
||||
|
|
|
@ -2,92 +2,317 @@
|
|||
#include "../imgui/imgui.h"
|
||||
#include "cimgui.h"
|
||||
|
||||
CIMGUI_API void ImFontConfig_DefaultConstructor(ImFontConfig* config)
|
||||
{
|
||||
*config = ImFontConfig();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_GetTexDataAsRGBA32(ImFontAtlas* atlas, unsigned char** out_pixels, int* out_width, int* out_height, int* out_bytes_per_pixel)
|
||||
{
|
||||
atlas->GetTexDataAsRGBA32(out_pixels, out_width, out_height, out_bytes_per_pixel);
|
||||
atlas->GetTexDataAsRGBA32(out_pixels, out_width, out_height, out_bytes_per_pixel);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_GetTexDataAsAlpha8(ImFontAtlas* atlas, unsigned char** out_pixels, int* out_width, int* out_height, int* out_bytes_per_pixel)
|
||||
{
|
||||
atlas->GetTexDataAsAlpha8(out_pixels, out_width, out_height, out_bytes_per_pixel);
|
||||
atlas->GetTexDataAsAlpha8(out_pixels, out_width, out_height, out_bytes_per_pixel);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_SetTexID(ImFontAtlas* atlas, ImTextureID id)
|
||||
{
|
||||
atlas->TexID = id;
|
||||
atlas->TexID = id;
|
||||
}
|
||||
|
||||
CIMGUI_API ImFont* ImFontAtlas_AddFont(ImFontAtlas* atlas, CONST ImFontConfig* font_cfg)
|
||||
{
|
||||
return atlas->AddFont(font_cfg);
|
||||
return atlas->AddFont(font_cfg);
|
||||
}
|
||||
|
||||
CIMGUI_API ImFont* ImFontAtlas_AddFontDefault(ImFontAtlas* atlas, CONST ImFontConfig* font_cfg)
|
||||
{
|
||||
return atlas->AddFontDefault(font_cfg);
|
||||
return atlas->AddFontDefault(font_cfg);
|
||||
}
|
||||
|
||||
CIMGUI_API ImFont* ImFontAtlas_AddFontFromFileTTF(ImFontAtlas* atlas,CONST char* filename, float size_pixels, CONST ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges)
|
||||
{
|
||||
return atlas->AddFontFromFileTTF(filename, size_pixels, font_cfg, glyph_ranges);
|
||||
return atlas->AddFontFromFileTTF(filename, size_pixels, font_cfg, glyph_ranges);
|
||||
}
|
||||
|
||||
CIMGUI_API ImFont* ImFontAtlas_AddFontFromMemoryTTF(ImFontAtlas* atlas, void* font_data, int font_size, float size_pixels, CONST ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges)
|
||||
{
|
||||
return atlas->AddFontFromMemoryTTF(font_data, font_size, size_pixels, font_cfg, glyph_ranges);
|
||||
return atlas->AddFontFromMemoryTTF(font_data, font_size, size_pixels, font_cfg, glyph_ranges);
|
||||
}
|
||||
|
||||
CIMGUI_API ImFont* ImFontAtlas_AddFontFromMemoryCompressedTTF(ImFontAtlas* atlas, CONST void* compressed_font_data, int compressed_font_size, float size_pixels, CONST ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges)
|
||||
{
|
||||
return atlas->AddFontFromMemoryCompressedTTF(compressed_font_data, compressed_font_size, size_pixels, font_cfg, glyph_ranges);
|
||||
return atlas->AddFontFromMemoryCompressedTTF(compressed_font_data, compressed_font_size, size_pixels, font_cfg, glyph_ranges);
|
||||
}
|
||||
|
||||
CIMGUI_API ImFont* ImFontAtlas_AddFontFromMemoryCompressedBase85TTF(ImFontAtlas* atlas, CONST char* compressed_font_data_base85, float size_pixels, CONST ImFontConfig* font_cfg, CONST ImWchar* glyph_ranges)
|
||||
{
|
||||
return atlas->AddFontFromMemoryCompressedBase85TTF(compressed_font_data_base85, size_pixels, font_cfg, glyph_ranges);
|
||||
return atlas->AddFontFromMemoryCompressedBase85TTF(compressed_font_data_base85, size_pixels, font_cfg, glyph_ranges);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_ClearTexData(ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->ClearTexData();
|
||||
return atlas->ClearTexData();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_ClearInputData(ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->ClearInputData();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_ClearFonts(ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->ClearFonts();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_Clear(ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->Clear();
|
||||
return atlas->Clear();
|
||||
}
|
||||
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesDefault(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->GetGlyphRangesDefault();
|
||||
return atlas->GetGlyphRangesDefault();
|
||||
}
|
||||
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesKorean(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->GetGlyphRangesKorean();
|
||||
return atlas->GetGlyphRangesKorean();
|
||||
}
|
||||
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesJapanese(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->GetGlyphRangesJapanese();
|
||||
return atlas->GetGlyphRangesJapanese();
|
||||
}
|
||||
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesChinese(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->GetGlyphRangesChinese();
|
||||
return atlas->GetGlyphRangesChinese();
|
||||
}
|
||||
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesCyrillic(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->GetGlyphRangesCyrillic();
|
||||
return atlas->GetGlyphRangesCyrillic();
|
||||
}
|
||||
|
||||
CIMGUI_API CONST ImWchar* ImFontAtlas_GetGlyphRangesThai(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->GetGlyphRangesThai();
|
||||
return atlas->GetGlyphRangesThai();
|
||||
}
|
||||
|
||||
CIMGUI_API ImTextureID ImFontAtlas_GetTexID(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->TexID;
|
||||
}
|
||||
|
||||
CIMGUI_API unsigned char* ImFontAtlas_GetTexPixelsAlpha8(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->TexPixelsAlpha8;
|
||||
}
|
||||
|
||||
CIMGUI_API unsigned int* ImFontAtlas_GetTexPixelsRGBA32(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->TexPixelsRGBA32;
|
||||
}
|
||||
|
||||
CIMGUI_API int ImFontAtlas_GetTexWidth(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->TexWidth;
|
||||
}
|
||||
|
||||
CIMGUI_API int ImFontAtlas_GetTexHeight(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->TexHeight;
|
||||
}
|
||||
|
||||
CIMGUI_API int ImFontAtlas_GetTexDesiredWidth(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->TexDesiredWidth;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_SetTexDesiredWidth(struct ImFontAtlas* atlas, int TexDesiredWidth_)
|
||||
{
|
||||
atlas->TexDesiredWidth = TexDesiredWidth_;
|
||||
}
|
||||
|
||||
CIMGUI_API int ImFontAtlas_GetTexGlyphPadding(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->TexGlyphPadding;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_SetTexGlyphPadding(struct ImFontAtlas* atlas, int TexGlyphPadding_)
|
||||
{
|
||||
atlas->TexGlyphPadding = TexGlyphPadding_;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFontAtlas_GetTexUvWhitePixel(struct ImFontAtlas* atlas, ImVec2* pOut)
|
||||
{
|
||||
*pOut = atlas->TexUvWhitePixel;
|
||||
}
|
||||
|
||||
// ImFontAtlas::Fonts;
|
||||
CIMGUI_API int ImFontAtlas_Fonts_size(struct ImFontAtlas* atlas)
|
||||
{
|
||||
return atlas->Fonts.size();
|
||||
}
|
||||
|
||||
CIMGUI_API ImFont* ImFontAtlas_Fonts_index(struct ImFontAtlas* atlas, int index)
|
||||
{
|
||||
return atlas->Fonts[index];
|
||||
}
|
||||
|
||||
// ImFont
|
||||
CIMGUI_API float ImFont_GetFontSize(const struct ImFont* font)
|
||||
{
|
||||
return font->FontSize;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_SetFontSize(struct ImFont* font, float FontSize_)
|
||||
{
|
||||
font->FontSize = FontSize_;
|
||||
}
|
||||
|
||||
CIMGUI_API float ImFont_GetScale(const struct ImFont* font)
|
||||
{
|
||||
return font->Scale;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_SetScale(struct ImFont* font, float Scale_)
|
||||
{
|
||||
font->Scale = Scale_;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_GetDisplayOffset(const struct ImFont* font, ImVec2* pOut)
|
||||
{
|
||||
*pOut = font->DisplayOffset;
|
||||
}
|
||||
|
||||
CIMGUI_API const struct ImFontGlyph* ImFont_GetFallbackGlyph(const struct ImFont* font)
|
||||
{
|
||||
return font->FallbackGlyph;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_SetFallbackGlyph(struct ImFont* font, const struct ImFontGlyph* FallbackGlyph_)
|
||||
{
|
||||
font->FallbackGlyph = FallbackGlyph_;
|
||||
}
|
||||
|
||||
CIMGUI_API float ImFont_GetFallbackAdvanceX(const struct ImFont* font)
|
||||
{
|
||||
return font->FallbackAdvanceX;
|
||||
}
|
||||
|
||||
CIMGUI_API ImWchar ImFont_GetFallbackChar(const struct ImFont* font)
|
||||
{
|
||||
return font->FallbackChar;
|
||||
}
|
||||
|
||||
CIMGUI_API short ImFont_GetConfigDataCount(const struct ImFont* font)
|
||||
{
|
||||
return font->ConfigDataCount;
|
||||
}
|
||||
|
||||
CIMGUI_API struct ImFontConfig* ImFont_GetConfigData(struct ImFont* font)
|
||||
{
|
||||
return font->ConfigData;
|
||||
}
|
||||
|
||||
CIMGUI_API struct ImFontAtlas* ImFont_GetContainerAtlas(struct ImFont* font)
|
||||
{
|
||||
return font->ContainerAtlas;
|
||||
}
|
||||
|
||||
CIMGUI_API float ImFont_GetAscent(const struct ImFont* font)
|
||||
{
|
||||
return font->Ascent;
|
||||
}
|
||||
|
||||
CIMGUI_API float ImFont_GetDescent(const struct ImFont* font)
|
||||
{
|
||||
return font->Descent;
|
||||
}
|
||||
|
||||
CIMGUI_API int ImFont_GetMetricsTotalSurface(const struct ImFont* font)
|
||||
{
|
||||
return font->MetricsTotalSurface;
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_ClearOutputData(struct ImFont* font)
|
||||
{
|
||||
font->ClearOutputData();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_BuildLookupTable(struct ImFont* font)
|
||||
{
|
||||
font->BuildLookupTable();
|
||||
}
|
||||
|
||||
CIMGUI_API const struct ImFontGlyph* ImFont_FindGlyph(const struct ImFont* font, ImWchar c)
|
||||
{
|
||||
return font->FindGlyph(c);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_SetFallbackChar(struct ImFont* font, ImWchar c)
|
||||
{
|
||||
font->SetFallbackChar(c);
|
||||
}
|
||||
|
||||
CIMGUI_API float ImFont_GetCharAdvance(const struct ImFont* font, ImWchar c)
|
||||
{
|
||||
return font->GetCharAdvance(c);
|
||||
}
|
||||
|
||||
CIMGUI_API bool ImFont_IsLoaded(const struct ImFont* font)
|
||||
{
|
||||
return font->IsLoaded();
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_CalcTextSizeA(const struct ImFont* font, ImVec2* pOut, float size, float max_width, float wrap_width, const char* text_begin, const char* text_end, const char** remaining)
|
||||
{
|
||||
*pOut = font->CalcTextSizeA(size, max_width, wrap_width, text_begin, text_end, remaining);
|
||||
}
|
||||
|
||||
CIMGUI_API const char* ImFont_CalcWordWrapPositionA(const struct ImFont* font, float scale, const char* text, const char* text_end, float wrap_width)
|
||||
{
|
||||
return font->CalcWordWrapPositionA(scale, text, text_end, wrap_width);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_RenderChar(const struct ImFont* font, ImDrawList* draw_list, float size, ImVec2 pos, ImU32 col, unsigned short c)
|
||||
{
|
||||
return font->RenderChar(draw_list, size, pos, col, c);
|
||||
}
|
||||
|
||||
CIMGUI_API void ImFont_RenderText(const struct ImFont* font, ImDrawList* draw_list, float size, ImVec2 pos, ImU32 col, const ImVec4* clip_rect, const char* text_begin, const char* text_end, float wrap_width, bool cpu_fine_clip)
|
||||
{
|
||||
return font->RenderText(draw_list, size, pos, col, *clip_rect, text_begin, text_end, wrap_width, cpu_fine_clip);
|
||||
}
|
||||
|
||||
// ImFontGlyph
|
||||
CIMGUI_API int ImFont_Glyphs_size(const struct ImFont* font)
|
||||
{
|
||||
return font->Glyphs.size();
|
||||
}
|
||||
|
||||
CIMGUI_API struct ImFontGlyph* ImFont_Glyphs_index(struct ImFont* font, int index)
|
||||
{
|
||||
return &font->Glyphs[index];
|
||||
}
|
||||
|
||||
// ImFont::IndexXAdvance
|
||||
CIMGUI_API int ImFont_IndexAdvanceX_size(const struct ImFont* font)
|
||||
{
|
||||
return font->IndexAdvanceX.size();
|
||||
}
|
||||
|
||||
CIMGUI_API float ImFont_IndexAdvanceX_index(const struct ImFont* font, int index)
|
||||
{
|
||||
return font->IndexAdvanceX[index];
|
||||
}
|
||||
|
||||
// ImFont::IndexLookup
|
||||
CIMGUI_API int ImFont_IndexLookup_size(const struct ImFont* font)
|
||||
{
|
||||
return font->IndexLookup.size();
|
||||
}
|
||||
|
||||
CIMGUI_API unsigned short ImFont_IndexLookup_index(const struct ImFont* font, int index)
|
||||
{
|
||||
return font->IndexLookup[index];
|
||||
}
|
||||
|
|
|
@ -45,44 +45,44 @@ Binaries/Demo
|
|||
-------------
|
||||
|
||||
You should be able to build the examples from sources (tested on Windows/Mac/Linux). If you don't, let me know! If you want to have a quick look at some Dear ImGui features, you can download Windows binaries of the demo app here:
|
||||
- [imgui-demo-binaries-20170723.zip](http://www.miracleworld.net/imgui/binaries/imgui-demo-binaries-20170723.zip) (Windows binaries, Dear ImGui 1.51+ 2017/07/23, 5 executables, 808 KB)
|
||||
- [imgui-demo-binaries-20171013.zip](http://www.miracleworld.net/imgui/binaries/imgui-demo-binaries-20171013.zip) (Windows binaries, Dear ImGui 1.52 WIP built 2017/10/13, 5 executables)
|
||||
|
||||
Bindings
|
||||
--------
|
||||
|
||||
Integrating Dear ImGui within your custom engine is a matter of wiring mouse/keyboard inputs and providing a render function that can bind a texture and render simple textured triangles. The examples/ folder is populated with applications doing just that. If you are an experienced programmer it should take you less than an hour to integrate Dear ImGui in your custom engine, but make sure to spend time reading the FAQ, the comments and other documentation!
|
||||
|
||||
_NB: those third-party bindings may be more or less maintained, more or less close to the spirit of original API and therefore I cannot give much guarantee about them. People who create language bindings sometimes haven't used the C++ API themselves (for the good reason that they aren't C++ users). Dear ImGui was designed with C++ in mind and some of the subtleties may be lost in translation with other languages. If your language supports it, I would suggest replicating the function overloading and default parameters used in the original, else the API may be harder to use. In doubt, please check the original C++ version first!_
|
||||
|
||||
_Integrating Dear ImGui within your custom engine is a matter of wiring mouse/keyboard inputs and providing a render function that can bind a texture and render simple textured triangles. The examples/ folder is populated with applications doing just that. If you are an experienced programmer it should take you less than an hour to integrate Dear ImGui in your custom engine, but make sure to spend time reading the FAQ, the comments and other documentation!_
|
||||
|
||||
Languages:
|
||||
- C - cimgui: thin c-api wrapper for ImGui https://github.com/Extrawurst/cimgui
|
||||
- C#/.Net - ImGui.NET: An ImGui wrapper for .NET Core https://github.com/mellinoe/ImGui.NET
|
||||
- D - DerelictImgui: Dynamic bindings for the D programming language: https://github.com/Extrawurst/DerelictImgui
|
||||
- Go - go-imgui https://github.com/Armored-Dragon/go-imgui
|
||||
- Lua - https://github.com/patrickriordan/imgui_lua_bindings
|
||||
- Pascal - imgui-pas https://github.com/dpethes/imgui-pas
|
||||
- Python - CyImGui: Python bindings for dear imgui using Cython: https://github.com/chromy/cyimgui
|
||||
- Python - pyimgui: Another Python bindings for dear imgui: https://github.com/swistakm/pyimgui
|
||||
- Rust - imgui-rs: Rust bindings for dear imgui https://github.com/Gekkio/imgui-rs
|
||||
- C (cimgui): thin c-api wrapper for ImGui https://github.com/Extrawurst/cimgui
|
||||
- C#/.Net (ImGui.NET): An ImGui wrapper for .NET Core https://github.com/mellinoe/ImGui.NET
|
||||
- D (DerelictImgui): Dynamic bindings for the D programming language: https://github.com/Extrawurst/DerelictImgui
|
||||
- Go (go-imgui): https://github.com/Armored-Dragon/go-imgui
|
||||
- Lua: https://github.com/patrickriordan/imgui_lua_bindings
|
||||
- Pascal (imgui-pas) https://github.com/dpethes/imgui-pas
|
||||
- Python (CyImGui): Python bindings for dear imgui using Cython: https://github.com/chromy/cyimgui
|
||||
- Python (pyimgui): Another Python bindings for dear imgui: https://github.com/swistakm/pyimgui
|
||||
- Rust (imgui-rs): Rust bindings for dear imgui https://github.com/Gekkio/imgui-rs
|
||||
|
||||
Frameworks:
|
||||
- Main ImGui repository include examples for DirectX9, DirectX10, DirectX11, OpenGL2/3, Vulkan, Allegro 5, SDL+GL2/3, iOS and Marmalade: https://github.com/ocornut/imgui/tree/master/examples
|
||||
- Unmerged PR: DirectX12 example (with issues) https://github.com/ocornut/imgui/pull/301
|
||||
- Unmerged PR: SDL2 + OpenGLES + Emscripten example https://github.com/ocornut/imgui/pull/336
|
||||
- Unmerged PR: FreeGlut + OpenGL2 example https://github.com/ocornut/imgui/pull/801
|
||||
- Unmerged PR: Native Win32 and OSX example https://github.com/ocornut/imgui/pull/281
|
||||
- Unmerged PR: Android Example https://github.com/ocornut/imgui/pull/421
|
||||
- Cinder backend for dear imgui https://github.com/simongeilfus/Cinder-ImGui
|
||||
- FlexGUI: Flexium/SFML backend for dear imgui https://github.com/DXsmiley/FlexGUI
|
||||
- IrrIMGUI: Irrlicht backend for dear imgui https://github.com/ZahlGraf/IrrIMGUI
|
||||
- UnrealEngine_ImGui: Unreal Engine 4 backend for dear imgui https://github.com/sronsse/UnrealEngine_ImGui
|
||||
- LÖVE backend for dear imgui https://github.com/slages/love-imgui
|
||||
- Ogre backend for dear imgui https://bitbucket.org/LMCrashy/ogreimgui/src
|
||||
- ofxImGui: openFrameworks backend for dear imgui https://github.com/jvcleave/ofxImGui
|
||||
- SFML backend for dear imgui https://github.com/EliasD/imgui-sfml
|
||||
- SFML backend for dear imgui https://github.com/Mischa-Alff/imgui-backends
|
||||
- cocos2d-x with imgui https://github.com/c0i/imguix https://github.com/ocornut/imgui/issues/551
|
||||
- NanoRT: software raytraced version https://github.com/syoyo/imgui/tree/nanort/examples/raytrace_example
|
||||
- Unmerged PR: DirectX12: https://github.com/ocornut/imgui/pull/301
|
||||
- Unmerged PR: SDL2 + OpenGLES + Emscripten: https://github.com/ocornut/imgui/pull/336
|
||||
- Unmerged PR: FreeGlut + OpenGL2: https://github.com/ocornut/imgui/pull/801
|
||||
- Unmerged PR: Native Win32 and OSX: https://github.com/ocornut/imgui/pull/281
|
||||
- Unmerged PR: Android: https://github.com/ocornut/imgui/pull/421
|
||||
- Cinder: https://github.com/simongeilfus/Cinder-ImGui
|
||||
- cocos2d-x: https://github.com/c0i/imguix https://github.com/ocornut/imgui/issues/551
|
||||
- Flexium/SFML (FlexGUI): https://github.com/DXsmiley/FlexGUI
|
||||
- Irrlicht (IrrIMGUI): https://github.com/ZahlGraf/IrrIMGUI
|
||||
- Ogre: https://bitbucket.org/LMCrashy/ogreimgui/src
|
||||
- openFrameworks (ofxImGui): https://github.com/jvcleave/ofxImGui
|
||||
- LÖVE: https://github.com/slages/love-imgui
|
||||
- NanoRT (software raytraced) https://github.com/syoyo/imgui/tree/nanort/examples/raytrace_example
|
||||
- Qt3d https://github.com/alpqr/imgui-qt3d
|
||||
- Unreal Engine 4: https://github.com/segross/UnrealImGui or https://github.com/sronsse/UnrealEngine_ImGui
|
||||
- SFML: https://github.com/EliasD/imgui-sfml or https://github.com/Mischa-Alff/imgui-backends
|
||||
|
||||
For other bindings: see [this page](https://github.com/ocornut/imgui/wiki/Links/).
|
||||
Please contact me with the Issues tracker or Twitter to fix/update this list.
|
||||
|
@ -91,6 +91,7 @@ Gallery
|
|||
-------
|
||||
|
||||
See the [Screenshots Thread](https://github.com/ocornut/imgui/issues/123) for some user creations.
|
||||
Also see the [Mega screenshots](https://github.com/ocornut/imgui/issues/1273) for an idea of the available features.
|
||||
|
||||
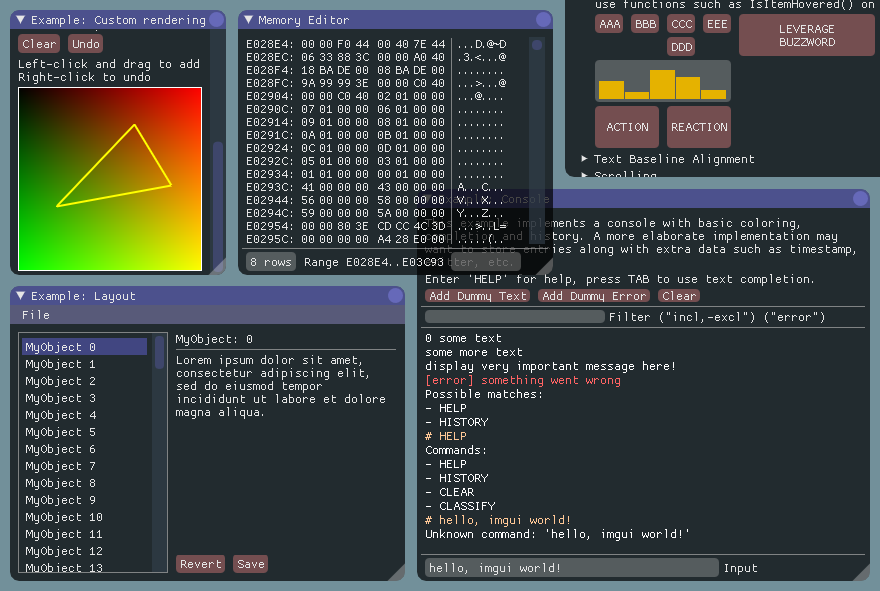
|
||||
[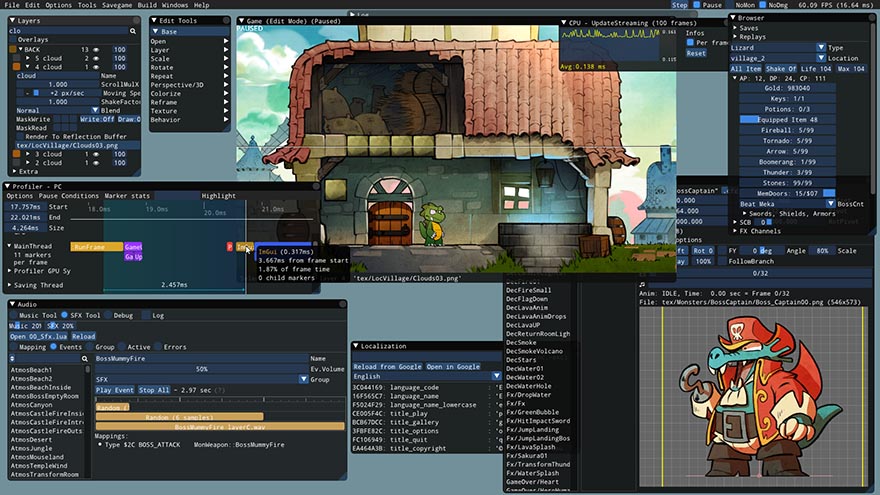](https://cloud.githubusercontent.com/assets/8225057/20628927/33e14cac-b329-11e6-80f6-9524e93b048a.png)
|
||||
|
@ -137,11 +138,15 @@ Frequently Asked Question (FAQ)
|
|||
- Standalone example applications using e.g. OpenGL/DirectX are provided in the examples/ folder.
|
||||
- We obviously needs better documentation! Consider contributing or becoming a [Patron](http://www.patreon.com/imgui) to promote this effort.
|
||||
|
||||
<b>Which version should I get?</b>
|
||||
|
||||
I occasionally tag [Releases](https://github.com/ocornut/imgui/releases) but it is generally safe and recommended to sync to master. The library is fairly stable and regressions tend to be fixed fast when reported. You may also want to checkout the [navigation branch](https://github.com/ocornut/imgui/tree/navigation) if you want to use Dear ImGui with a gamepad (it is also possible to map keyboard inputs to some degree). The Navigation branch is being kept up to date with Master.
|
||||
|
||||
<b>Why the odd dual naming, "dear imgui" vs "ImGui"?</b>
|
||||
|
||||
The library started its life and is best known as "ImGui" only due to the fact that I didn't give it a proper name when I released it. However, the term IMGUI (immediate-mode graphical user interface) was coined before and is being used in variety of other situations. It seemed confusing and unfair to hog the name. To reduce the ambiguity without affecting existing codebases, I have decided on an alternate, longer name "dear imgui" that people can use to refer to this specific library in ambiguous situations.
|
||||
|
||||
<br><b>What is ImTextureID and how do I display an image?</b>
|
||||
<b>What is ImTextureID and how do I display an image?</b>
|
||||
<br><b>I integrated Dear ImGui in my engine and the text or lines are blurry..</b>
|
||||
<br><b>I integrated Dear ImGui in my engine and some elements are disappearing when I move windows around..</b>
|
||||
<br><b>How can I have multiple widgets with the same label? Can I have widget without a label? (Yes). A primer on labels/IDs.</b>
|
||||
|
@ -157,13 +162,13 @@ See the FAQ in imgui.cpp for answers.
|
|||
|
||||
<b>How do you use Dear ImGui on a platform that may not have a mouse or keyboard?</b>
|
||||
|
||||
I recommend using [Synergy](http://synergy-project.org) ([sources](https://github.com/symless/synergy)). In particular, the _src/micro/uSynergy.c_ file contains a small client that you can use on any platform to connect to your host PC. You can seamlessly use your PC input devices from a video game console or a tablet. Dear ImGui allows to increase the hit box of widgets (via the _TouchPadding_ setting) to accommodate a little for the lack of precision of touch inputs, but it is recommended you use a mouse to allow optimising for screen real-estate.
|
||||
I recommend using [Synergy](http://synergy-project.org) ([sources](https://github.com/symless/synergy)). In particular, the _src/micro/uSynergy.c_ file contains a small client that you can use on any platform to connect to your host PC. You can seamlessly use your PC input devices from a video game console or a tablet. Dear ImGui allows to increase the hit box of widgets (via the _style.TouchPadding_ setting) to accommodate a little for the lack of precision of touch inputs, but it is recommended you use a mouse to allow optimising for screen real-estate. You can also checkout the beta [navigation branch](https://github.com/ocornut/imgui/tree/navigation) which provides support for using Dear ImGui with a game controller.
|
||||
|
||||
<b>Can you create elaborate/serious tools with Dear ImGui?</b>
|
||||
|
||||
Yes. I have written data browsers, debuggers, profilers and all sort of non-trivial tools with the library. In my experience the simplicity of the API is very empowering. Your UI runs close to your live data. Make the tools always-on and everybody in the team will be inclined to create new tools (as opposed to more "offline" UI toolkits where only a fraction of your team effectively creates tools).
|
||||
Yes. People have written game editors, data browsers, debuggers, profilers and all sort of non-trivial tools with the library. In my experience the simplicity of the API is very empowering. Your UI runs close to your live data. Make the tools always-on and everybody in the team will be inclined to create new tools (as opposed to more "offline" UI toolkits where only a fraction of your team effectively creates tools).
|
||||
|
||||
Dear ImGui is very programmer centric and the immediate-mode GUI paradigm might requires you to readjust some habits before you can realize its full potential. Many programmers have unfortunately been taught by their environment to make unnecessarily complicated things. Dear ImGui is about making things that are simple, efficient and powerful.
|
||||
Dear ImGui is very programmer centric and the immediate-mode GUI paradigm might requires you to readjust some habits before you can realize its full potential. Dear ImGui is about making things that are simple, efficient and powerful.
|
||||
|
||||
<b>Is Dear ImGui fast?</b>
|
||||
|
||||
|
@ -179,17 +184,15 @@ If you intend to display large lists of items (say, 1000+) it can be beneficial
|
|||
|
||||
<b>Can you reskin the look of Dear ImGui?</b>
|
||||
|
||||
You can alter the look of the interface to some degree: changing colors, sizes, padding, rounding, fonts. However, as Dear ImGui is designed and optimised to create debug tools, the amount of skinning you can apply is limited. There is only so much you can stray away from the default look and feel of the interface.
|
||||
You can alter the look of the interface to some degree: changing colors, sizes, padding, rounding, fonts. However, as Dear ImGui is designed and optimised to create debug tools, the amount of skinning you can apply is limited. There is only so much you can stray away from the default look and feel of the interface. Below is a screenshot from [LumixEngine](https://github.com/nem0/LumixEngine) with custom colors + a docking/tabs extension (both of which you can find in the Issues section and will eventually be merged):
|
||||
|
||||
This is [LumixEngine](https://github.com/nem0/LumixEngine) with a minor skinning hack + a docking/tabs extension (both of which you can find in the Issues section and will eventually be merged).
|
||||
|
||||
[](https://cloud.githubusercontent.com/assets/8225057/13044612/59f07aec-d3cf-11e5-8ccb-39adf2e13e69.png)
|
||||
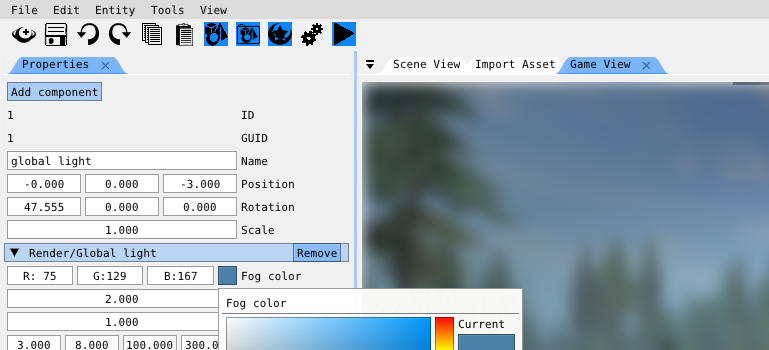
|
||||
|
||||
<b>Why using C++ (as opposed to C)?</b>
|
||||
|
||||
Dear ImGui takes advantage of a few C++ languages features for convenience but nothing anywhere Boost-insanity/quagmire. Dear ImGui doesn't use any C++ header file. Language-wise, function overloading and default parameters are used to make the API easier to use and code more terse. Doing so I believe the API is sitting on a sweet spot and giving up on those features would make the API more cumbersome. Other features such as namespace, constructors and templates (in the case of the ImVector<> class) are also relied on as a convenience.
|
||||
Dear ImGui takes advantage of a few C++ languages features for convenience but nothing anywhere Boost-insanity/quagmire. Dear ImGui does NOT require C++11 so it can be used with most old C++ compilers. Dear ImGui doesn't use any C++ header file. Language-wise, function overloading and default parameters are used to make the API easier to use and code more terse. Doing so I believe the API is sitting on a sweet spot and giving up on those features would make the API more cumbersome. Other features such as namespace, constructors and templates (in the case of the ImVector<> class) are also relied on as a convenience.
|
||||
|
||||
There is an unofficial but reasonably maintained [c-api for ImGui](https://github.com/Extrawurst/cimgui) by Stephan Dilly. I would suggest using your target language functionality to try replicating the function overloading and default parameters used in C++ else the API may be harder to use. It was really designed with C++ in mind and may not make the same amount of sense with another language. Also see [Links](https://github.com/ocornut/imgui/wiki/Links) for third-party bindings to other languages.
|
||||
There is an reasonably maintained [c-api for ImGui](https://github.com/Extrawurst/cimgui) by Stephan Dilly designed for binding in other languages. I would suggest using your target language functionalities to try replicating the function overloading and default parameters used in C++ else the API may be harder to use. Also see [Links](https://github.com/ocornut/imgui/wiki/Links) for third-party bindings to other languages.
|
||||
|
||||
Support dear imgui
|
||||
------------------
|
||||
|
|
|
@ -7,6 +7,7 @@ It's mostly a bunch of personal notes, probably incomplete. Feel free to query i
|
|||
|
||||
- doc/test: add a proper documentation+regression testing system (#435)
|
||||
- doc/test: checklist app to verify binding/integration of imgui (test inputs, rendering, callback, etc.).
|
||||
- doc/tips: tips of the day: website? applet in imgui_club?
|
||||
- project: folder or separate repository with maintained helpers (e.g. imgui_memory_editor.h, imgui_stl.h, maybe imgui_dock would be there?)
|
||||
|
||||
- window: calling SetNextWindowSize() every frame with <= 0 doesn't do anything, may be useful to allow (particularly when used for a single axis). (#690)
|
||||
|
@ -20,7 +21,6 @@ It's mostly a bunch of personal notes, probably incomplete. Feel free to query i
|
|||
- window: a collapsed window can be stuck behind the main menu bar?
|
||||
- window: when window is very small, prioritize resize button over close button.
|
||||
- window: detect extra End() call that pop the "Debug" window out and assert at End() call site instead of at end of frame.
|
||||
- window/tooltip: allow to set the width of a tooltip to allow TextWrapped() etc. while keeping the height automatic.
|
||||
- window: increase minimum size of a window with menus or fix the menu rendering so that it doesn't look odd.
|
||||
- window: double-clicking on title bar to minimize isn't consistent, perhaps move to single-click on left-most collapse icon?
|
||||
- window: expose contents size. (#1045)
|
||||
|
@ -86,6 +86,7 @@ It's mostly a bunch of personal notes, probably incomplete. Feel free to query i
|
|||
- columns: optional sorting modifiers (up/down), sort list so sorting can be done multi-critera. notify user when sort order changed.
|
||||
- columns: option to alternate background colors on odd/even scanlines.
|
||||
- columns: allow columns to recurse.
|
||||
- columns: allow a same columns set to be interrupted by e.g. CollapsingHeader and resume with columns in sync when moving them.
|
||||
- columns: separator function or parameter that works within the column (currently Separator() bypass all columns) (#125)
|
||||
- columns: flag to add horizontal separator above/below?
|
||||
- columns/layout: setup minimum line height (equivalent of automatically calling AlignFirstTextHeightToWidgets)
|
||||
|
@ -152,7 +153,9 @@ It's mostly a bunch of personal notes, probably incomplete. Feel free to query i
|
|||
- popups: add variant using global identifier similar to Begin/End (#402)
|
||||
- popups: border options. richer api like BeginChild() perhaps? (#197)
|
||||
- tooltip: tooltip that doesn't fit in entire screen seems to lose their "last preferred direction" and may teleport when moving mouse.
|
||||
|
||||
- tooltip: allow to set the width of a tooltip to allow TextWrapped() etc. while keeping the height automatic.
|
||||
- tooltip: allow tooltips with timers? or general timer policy? (instaneous vs timed)
|
||||
|
||||
- menus: calling BeginMenu() twice with a same name doesn't append as Begin() does for regular windows (#1207)
|
||||
- statusbar: add a per-window status bar helper similar to what menubar does.
|
||||
- shortcuts: local-style shortcut api, e.g. parse "&Save"
|
||||
|
@ -205,7 +208,8 @@ It's mostly a bunch of personal notes, probably incomplete. Feel free to query i
|
|||
- markup: simple markup language for color change?
|
||||
|
||||
!- font: better CalcTextSizeA() API, at least for simple use cases. current one is horrible (perhaps have simple vs extended versions).
|
||||
- font: enforce monospace through ImFontConfig (for icons?)
|
||||
- font: better vertical centering (based e.g on height of lowercase 'x'?). currently Roboto-Medium size 16 px isn't currently centered.
|
||||
- font: enforce monospace through ImFontConfig (for icons?) + create dual ImFont output from same input, reusing rasterized data but with different glyphs/AdvanceX
|
||||
- font: finish CustomRectRegister() to allow mapping unicode codepoint to custom texture data
|
||||
- font: PushFontSize API (#1018)
|
||||
- font/atlas: incremental updates
|
||||
|
@ -236,6 +240,7 @@ It's mostly a bunch of personal notes, probably incomplete. Feel free to query i
|
|||
- misc: provide a way to compile out the entire implementation while providing a dummy API (e.g. #define IMGUI_DUMMY_IMPL)
|
||||
- misc: provide HoveredTime and ActivatedTime to ease the creation of animations.
|
||||
- misc: fix for compilation settings where stdcall isn't the default (e.g. vectorcall) (#1230)
|
||||
- misc: detect user not calling Render() and suggest to call EndFrame()?
|
||||
- remote: make a system like RemoteImGui first-class citizen/project (#75)
|
||||
|
||||
- demo: add vertical separator demo
|
||||
|
|
|
@ -8,15 +8,16 @@ Third party languages and frameworks bindings: https://github.com/ocornut/imgui/
|
|||
|
||||
TL;DR;
|
||||
- Newcomers, read 'PROGRAMMER GUIDE' in imgui.cpp for notes on how to setup ImGui in your codebase.
|
||||
- To LEARN how the library is setup, you may refer to 'opengl2_example' because is the simplest one.
|
||||
The other examples requires more boilerplate and are harder to read.
|
||||
However, USE 'opengl3_example' in your application if you are using any modern OpenGL3+ calls.
|
||||
Mixing old fixed pipeline OpenGL2 and programmable pipeline OpenGL3+ isn't well supported by some drivers.
|
||||
If you are not sure, in doubt, use 'opengl3_example'.
|
||||
- If you are using of the backend provided here, so you can copy the imgui_impl_xxx.cpp/h files
|
||||
to your project and use them unmodified.
|
||||
- If you have your own engine, you probably want to start from one of the OpenGL example and adapt it to
|
||||
your engine, but you can read the other examples as well.
|
||||
- To LEARN how the library is setup, you may refer to 'opengl2_example' because is the simplest one to read.
|
||||
However, do NOT USE the 'opengl2_example' if your code is using any modern GL3+ calls.
|
||||
Mixing old fixed-pipeline OpenGL2 and modern OpenGL3+ is going to make everything more complicated.
|
||||
Read comments below for details. If you are not sure, in doubt, use 'opengl3_example'.
|
||||
- If you have your own engine, you probably want to read a few of the examples first then adapt it to
|
||||
your engine. Please note that if your engine is based on OpenGL/DirectX you can perfectly use the
|
||||
existing rendering backends, don't feel forced to rewrite them with your own engine API, or you can
|
||||
do that later when you already got things to work.
|
||||
|
||||
ImGui is highly portable and only requires a few things to run:
|
||||
- Providing mouse/keyboard inputs
|
||||
|
@ -45,12 +46,12 @@ Also note that some setup or GPU drivers may be causing extra lag (possibly by e
|
|||
leaving you with no option but sadness/anger (Intel GPU drivers were reported as such).
|
||||
|
||||
opengl2_example/
|
||||
*DO NOT USE THIS CODE IF YOUR CODE/ENGINE IS USING MODERN OPENGL*
|
||||
GLFW + OpenGL example (old, fixed graphic pipeline).
|
||||
This is only provided as a reference to learn how ImGui integration works, because it is easier to read.
|
||||
However, if your code is using GL3+ context, using this may confuse your driver. Please use the GL3 example below.
|
||||
(You might be able to use this code in a GL3/GL4 context but make sure you disable the programmable
|
||||
pipeline by calling "glUseProgram(0)" before ImGui::Render. It appears that many librairies and drivers
|
||||
are having issues mixing GL2 calls and newer GL3/GL4 calls. So it isn't recommended that you use that.)
|
||||
This is mostly provided as a reference to learn how ImGui integration works, because it is easier to read.
|
||||
If your code is using GL3+ context or any semi modern OpenGL calls, using this is likely to make everything
|
||||
more complicated, will require your code to reset every single OpenGL attributes to their initial state,
|
||||
and might confuse your GPU driver. Prefer using opengl3_example.
|
||||
|
||||
opengl3_example/
|
||||
GLFW + OpenGL example (programmable pipeline, binding modern functions with GL3W).
|
||||
|
@ -74,7 +75,12 @@ apple_example/
|
|||
Synergy keyboard integration is rather hacky.
|
||||
|
||||
sdl_opengl2_example/
|
||||
*DO NOT USE THIS CODE IF YOUR CODE/ENGINE IS USING MODERN OPENGL*
|
||||
SDL2 + OpenGL example (old fixed pipeline).
|
||||
This is mostly provided as a reference to learn how ImGui integration works, because it is easier to read.
|
||||
If your code is using GL3+ context or any semi modern OpenGL calls, using this is likely to make everything
|
||||
more complicated, will require your code to reset every single OpenGL attributes to their initial state,
|
||||
and might confuse your GPU driver. Prefer using sdl_opengl3_example.
|
||||
|
||||
sdl_opengl3_example/
|
||||
SDL2 + OpenGL3 example.
|
||||
|
|
|
@ -204,6 +204,10 @@ void ImGui_ImplA5_Shutdown()
|
|||
ImGui::Shutdown();
|
||||
}
|
||||
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
bool ImGui_ImplA5_ProcessEvent(ALLEGRO_EVENT *ev)
|
||||
{
|
||||
ImGuiIO &io = ImGui::GetIO();
|
||||
|
@ -227,7 +231,6 @@ bool ImGui_ImplA5_ProcessEvent(ALLEGRO_EVENT *ev)
|
|||
return false;
|
||||
}
|
||||
|
||||
|
||||
void ImGui_ImplA5_NewFrame()
|
||||
{
|
||||
if (!g_Texture)
|
||||
|
@ -290,6 +293,6 @@ void ImGui_ImplA5_NewFrame()
|
|||
al_set_system_mouse_cursor(g_Display, cursor_id);
|
||||
}
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
}
|
||||
|
|
|
@ -26,14 +26,20 @@ int main(int, char**)
|
|||
ImGui_ImplA5_Init(display);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
bool show_test_window = true;
|
||||
bool show_another_window = false;
|
||||
|
@ -43,11 +49,16 @@ int main(int, char**)
|
|||
bool running = true;
|
||||
while (running)
|
||||
{
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
ALLEGRO_EVENT ev;
|
||||
while (al_get_next_event(queue, &ev))
|
||||
{
|
||||
ImGui_ImplA5_ProcessEvent(&ev);
|
||||
if (ev.type == ALLEGRO_EVENT_DISPLAY_CLOSE) running = false;
|
||||
if (ev.type == ALLEGRO_EVENT_DISPLAY_CLOSE)
|
||||
running = false;
|
||||
if (ev.type == ALLEGRO_EVENT_DISPLAY_RESIZE)
|
||||
{
|
||||
ImGui_ImplA5_InvalidateDeviceObjects();
|
||||
|
@ -57,8 +68,8 @@ int main(int, char**)
|
|||
}
|
||||
ImGui_ImplA5_NewFrame();
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// 1. Show a simple window.
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -69,7 +80,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f/ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -77,7 +88,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver);
|
||||
|
|
|
@ -225,49 +225,74 @@ void ImGui_ImplDX10_RenderDrawLists(ImDrawData* draw_data)
|
|||
ctx->IASetInputLayout(old.InputLayout); if (old.InputLayout) old.InputLayout->Release();
|
||||
}
|
||||
|
||||
IMGUI_API LRESULT ImGui_ImplDX10_WndProcHandler(HWND, UINT msg, WPARAM wParam, LPARAM lParam)
|
||||
static bool IsAnyMouseButtonDown()
|
||||
{
|
||||
ImGuiIO& io = ImGui::GetIO();
|
||||
for (int n = 0; n < ARRAYSIZE(io.MouseDown); n++)
|
||||
if (io.MouseDown[n])
|
||||
return true;
|
||||
return false;
|
||||
}
|
||||
|
||||
// Process Win32 mouse/keyboard inputs.
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
// PS: In this Win32 handler, we use the capture API (GetCapture/SetCapture/ReleaseCapture) to be able to read mouse coordinations when dragging mouse outside of our window bounds.
|
||||
IMGUI_API LRESULT ImGui_ImplWin32_WndProcHandler(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
|
||||
{
|
||||
ImGuiIO& io = ImGui::GetIO();
|
||||
switch (msg)
|
||||
{
|
||||
case WM_LBUTTONDOWN:
|
||||
io.MouseDown[0] = true;
|
||||
return true;
|
||||
case WM_LBUTTONUP:
|
||||
io.MouseDown[0] = false;
|
||||
return true;
|
||||
case WM_RBUTTONDOWN:
|
||||
io.MouseDown[1] = true;
|
||||
return true;
|
||||
case WM_RBUTTONUP:
|
||||
io.MouseDown[1] = false;
|
||||
return true;
|
||||
case WM_MBUTTONDOWN:
|
||||
io.MouseDown[2] = true;
|
||||
return true;
|
||||
{
|
||||
int button = 0;
|
||||
if (msg == WM_LBUTTONDOWN) button = 0;
|
||||
if (msg == WM_RBUTTONDOWN) button = 1;
|
||||
if (msg == WM_MBUTTONDOWN) button = 2;
|
||||
if (!IsAnyMouseButtonDown() && GetCapture() == NULL)
|
||||
SetCapture(hwnd);
|
||||
io.MouseDown[button] = true;
|
||||
return 0;
|
||||
}
|
||||
case WM_LBUTTONUP:
|
||||
case WM_RBUTTONUP:
|
||||
case WM_MBUTTONUP:
|
||||
io.MouseDown[2] = false;
|
||||
return true;
|
||||
{
|
||||
int button = 0;
|
||||
if (msg == WM_LBUTTONUP) button = 0;
|
||||
if (msg == WM_RBUTTONUP) button = 1;
|
||||
if (msg == WM_MBUTTONUP) button = 2;
|
||||
io.MouseDown[button] = false;
|
||||
if (!IsAnyMouseButtonDown() && GetCapture() == hwnd)
|
||||
ReleaseCapture();
|
||||
return 0;
|
||||
}
|
||||
case WM_MOUSEWHEEL:
|
||||
io.MouseWheel += GET_WHEEL_DELTA_WPARAM(wParam) > 0 ? +1.0f : -1.0f;
|
||||
return true;
|
||||
return 0;
|
||||
case WM_MOUSEMOVE:
|
||||
io.MousePos.x = (signed short)(lParam);
|
||||
io.MousePos.y = (signed short)(lParam >> 16);
|
||||
return true;
|
||||
return 0;
|
||||
case WM_KEYDOWN:
|
||||
case WM_SYSKEYDOWN:
|
||||
if (wParam < 256)
|
||||
io.KeysDown[wParam] = 1;
|
||||
return true;
|
||||
return 0;
|
||||
case WM_KEYUP:
|
||||
case WM_SYSKEYUP:
|
||||
if (wParam < 256)
|
||||
io.KeysDown[wParam] = 0;
|
||||
return true;
|
||||
return 0;
|
||||
case WM_CHAR:
|
||||
// You can also use ToAscii()+GetKeyboardState() to retrieve characters.
|
||||
if (wParam > 0 && wParam < 0x10000)
|
||||
io.AddInputCharacter((unsigned short)wParam);
|
||||
return true;
|
||||
return 0;
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
|
@ -584,6 +609,6 @@ void ImGui_ImplDX10_NewFrame()
|
|||
if (io.MouseDrawCursor)
|
||||
SetCursor(NULL);
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
}
|
||||
|
|
|
@ -74,10 +74,10 @@ void CleanupDeviceD3D()
|
|||
if (g_pd3dDevice) { g_pd3dDevice->Release(); g_pd3dDevice = NULL; }
|
||||
}
|
||||
|
||||
extern LRESULT ImGui_ImplDX10_WndProcHandler(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam);
|
||||
extern LRESULT ImGui_ImplWin32_WndProcHandler(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam);
|
||||
LRESULT WINAPI WndProc(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam)
|
||||
{
|
||||
if (ImGui_ImplDX10_WndProcHandler(hWnd, msg, wParam, lParam))
|
||||
if (ImGui_ImplWin32_WndProcHandler(hWnd, msg, wParam, lParam))
|
||||
return true;
|
||||
|
||||
switch (msg)
|
||||
|
@ -126,14 +126,20 @@ int main(int, char**)
|
|||
ImGui_ImplDX10_Init(hwnd, g_pd3dDevice);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
bool show_test_window = true;
|
||||
bool show_another_window = false;
|
||||
|
@ -144,6 +150,10 @@ int main(int, char**)
|
|||
ZeroMemory(&msg, sizeof(msg));
|
||||
while (msg.message != WM_QUIT)
|
||||
{
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
if (PeekMessage(&msg, NULL, 0U, 0U, PM_REMOVE))
|
||||
{
|
||||
TranslateMessage(&msg);
|
||||
|
@ -152,8 +162,8 @@ int main(int, char**)
|
|||
}
|
||||
ImGui_ImplDX10_NewFrame();
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// 1. Show a simple window.
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f = 0.0f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -164,7 +174,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -172,7 +182,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver); // Normally user code doesn't need/want to call it because positions are saved in .ini file anyway. Here we just want to make the demo initial state a bit more friendly!
|
||||
|
|
|
@ -232,49 +232,74 @@ void ImGui_ImplDX11_RenderDrawLists(ImDrawData* draw_data)
|
|||
ctx->IASetInputLayout(old.InputLayout); if (old.InputLayout) old.InputLayout->Release();
|
||||
}
|
||||
|
||||
IMGUI_API LRESULT ImGui_ImplDX11_WndProcHandler(HWND, UINT msg, WPARAM wParam, LPARAM lParam)
|
||||
static bool IsAnyMouseButtonDown()
|
||||
{
|
||||
ImGuiIO& io = ImGui::GetIO();
|
||||
for (int n = 0; n < ARRAYSIZE(io.MouseDown); n++)
|
||||
if (io.MouseDown[n])
|
||||
return true;
|
||||
return false;
|
||||
}
|
||||
|
||||
// Process Win32 mouse/keyboard inputs.
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
// PS: In this Win32 handler, we use the capture API (GetCapture/SetCapture/ReleaseCapture) to be able to read mouse coordinations when dragging mouse outside of our window bounds.
|
||||
IMGUI_API LRESULT ImGui_ImplWin32_WndProcHandler(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
|
||||
{
|
||||
ImGuiIO& io = ImGui::GetIO();
|
||||
switch (msg)
|
||||
{
|
||||
case WM_LBUTTONDOWN:
|
||||
io.MouseDown[0] = true;
|
||||
return true;
|
||||
case WM_LBUTTONUP:
|
||||
io.MouseDown[0] = false;
|
||||
return true;
|
||||
case WM_RBUTTONDOWN:
|
||||
io.MouseDown[1] = true;
|
||||
return true;
|
||||
case WM_RBUTTONUP:
|
||||
io.MouseDown[1] = false;
|
||||
return true;
|
||||
case WM_MBUTTONDOWN:
|
||||
io.MouseDown[2] = true;
|
||||
return true;
|
||||
{
|
||||
int button = 0;
|
||||
if (msg == WM_LBUTTONDOWN) button = 0;
|
||||
if (msg == WM_RBUTTONDOWN) button = 1;
|
||||
if (msg == WM_MBUTTONDOWN) button = 2;
|
||||
if (!IsAnyMouseButtonDown() && GetCapture() == NULL)
|
||||
SetCapture(hwnd);
|
||||
io.MouseDown[button] = true;
|
||||
return 0;
|
||||
}
|
||||
case WM_LBUTTONUP:
|
||||
case WM_RBUTTONUP:
|
||||
case WM_MBUTTONUP:
|
||||
io.MouseDown[2] = false;
|
||||
return true;
|
||||
{
|
||||
int button = 0;
|
||||
if (msg == WM_LBUTTONUP) button = 0;
|
||||
if (msg == WM_RBUTTONUP) button = 1;
|
||||
if (msg == WM_MBUTTONUP) button = 2;
|
||||
io.MouseDown[button] = false;
|
||||
if (!IsAnyMouseButtonDown() && GetCapture() == hwnd)
|
||||
ReleaseCapture();
|
||||
return 0;
|
||||
}
|
||||
case WM_MOUSEWHEEL:
|
||||
io.MouseWheel += GET_WHEEL_DELTA_WPARAM(wParam) > 0 ? +1.0f : -1.0f;
|
||||
return true;
|
||||
return 0;
|
||||
case WM_MOUSEMOVE:
|
||||
io.MousePos.x = (signed short)(lParam);
|
||||
io.MousePos.y = (signed short)(lParam >> 16);
|
||||
return true;
|
||||
return 0;
|
||||
case WM_KEYDOWN:
|
||||
case WM_SYSKEYDOWN:
|
||||
if (wParam < 256)
|
||||
io.KeysDown[wParam] = 1;
|
||||
return true;
|
||||
return 0;
|
||||
case WM_KEYUP:
|
||||
case WM_SYSKEYUP:
|
||||
if (wParam < 256)
|
||||
io.KeysDown[wParam] = 0;
|
||||
return true;
|
||||
return 0;
|
||||
case WM_CHAR:
|
||||
// You can also use ToAscii()+GetKeyboardState() to retrieve characters.
|
||||
if (wParam > 0 && wParam < 0x10000)
|
||||
io.AddInputCharacter((unsigned short)wParam);
|
||||
return true;
|
||||
return 0;
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
|
@ -587,6 +612,6 @@ void ImGui_ImplDX11_NewFrame()
|
|||
if (io.MouseDrawCursor)
|
||||
SetCursor(NULL);
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
}
|
||||
|
|
|
@ -77,10 +77,10 @@ void CleanupDeviceD3D()
|
|||
if (g_pd3dDevice) { g_pd3dDevice->Release(); g_pd3dDevice = NULL; }
|
||||
}
|
||||
|
||||
extern LRESULT ImGui_ImplDX11_WndProcHandler(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam);
|
||||
extern LRESULT ImGui_ImplWin32_WndProcHandler(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam);
|
||||
LRESULT WINAPI WndProc(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam)
|
||||
{
|
||||
if (ImGui_ImplDX11_WndProcHandler(hWnd, msg, wParam, lParam))
|
||||
if (ImGui_ImplWin32_WndProcHandler(hWnd, msg, wParam, lParam))
|
||||
return true;
|
||||
|
||||
switch (msg)
|
||||
|
@ -129,14 +129,20 @@ int main(int, char**)
|
|||
ImGui_ImplDX11_Init(hwnd, g_pd3dDevice, g_pd3dDeviceContext);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
bool show_test_window = true;
|
||||
bool show_another_window = false;
|
||||
|
@ -147,6 +153,10 @@ int main(int, char**)
|
|||
ZeroMemory(&msg, sizeof(msg));
|
||||
while (msg.message != WM_QUIT)
|
||||
{
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
if (PeekMessage(&msg, NULL, 0U, 0U, PM_REMOVE))
|
||||
{
|
||||
TranslateMessage(&msg);
|
||||
|
@ -155,8 +165,8 @@ int main(int, char**)
|
|||
}
|
||||
ImGui_ImplDX11_NewFrame();
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// 1. Show a simple window.
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f = 0.0f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -167,7 +177,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -175,7 +185,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver); // Normally user code doesn't need/want to call it because positions are saved in .ini file anyway. Here we just want to make the demo initial state a bit more friendly!
|
||||
|
|
|
@ -171,49 +171,74 @@ void ImGui_ImplDX9_RenderDrawLists(ImDrawData* draw_data)
|
|||
d3d9_state_block->Release();
|
||||
}
|
||||
|
||||
IMGUI_API LRESULT ImGui_ImplDX9_WndProcHandler(HWND, UINT msg, WPARAM wParam, LPARAM lParam)
|
||||
static bool IsAnyMouseButtonDown()
|
||||
{
|
||||
ImGuiIO& io = ImGui::GetIO();
|
||||
for (int n = 0; n < ARRAYSIZE(io.MouseDown); n++)
|
||||
if (io.MouseDown[n])
|
||||
return true;
|
||||
return false;
|
||||
}
|
||||
|
||||
// Process Win32 mouse/keyboard inputs.
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
// PS: In this Win32 handler, we use the capture API (GetCapture/SetCapture/ReleaseCapture) to be able to read mouse coordinations when dragging mouse outside of our window bounds.
|
||||
IMGUI_API LRESULT ImGui_ImplWin32_WndProcHandler(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
|
||||
{
|
||||
ImGuiIO& io = ImGui::GetIO();
|
||||
switch (msg)
|
||||
{
|
||||
case WM_LBUTTONDOWN:
|
||||
io.MouseDown[0] = true;
|
||||
return true;
|
||||
case WM_LBUTTONUP:
|
||||
io.MouseDown[0] = false;
|
||||
return true;
|
||||
case WM_RBUTTONDOWN:
|
||||
io.MouseDown[1] = true;
|
||||
return true;
|
||||
case WM_RBUTTONUP:
|
||||
io.MouseDown[1] = false;
|
||||
return true;
|
||||
case WM_MBUTTONDOWN:
|
||||
io.MouseDown[2] = true;
|
||||
return true;
|
||||
{
|
||||
int button = 0;
|
||||
if (msg == WM_LBUTTONDOWN) button = 0;
|
||||
if (msg == WM_RBUTTONDOWN) button = 1;
|
||||
if (msg == WM_MBUTTONDOWN) button = 2;
|
||||
if (!IsAnyMouseButtonDown() && GetCapture() == NULL)
|
||||
SetCapture(hwnd);
|
||||
io.MouseDown[button] = true;
|
||||
return 0;
|
||||
}
|
||||
case WM_LBUTTONUP:
|
||||
case WM_RBUTTONUP:
|
||||
case WM_MBUTTONUP:
|
||||
io.MouseDown[2] = false;
|
||||
return true;
|
||||
{
|
||||
int button = 0;
|
||||
if (msg == WM_LBUTTONUP) button = 0;
|
||||
if (msg == WM_RBUTTONUP) button = 1;
|
||||
if (msg == WM_MBUTTONUP) button = 2;
|
||||
io.MouseDown[button] = false;
|
||||
if (!IsAnyMouseButtonDown() && GetCapture() == hwnd)
|
||||
ReleaseCapture();
|
||||
return 0;
|
||||
}
|
||||
case WM_MOUSEWHEEL:
|
||||
io.MouseWheel += GET_WHEEL_DELTA_WPARAM(wParam) > 0 ? +1.0f : -1.0f;
|
||||
return true;
|
||||
return 0;
|
||||
case WM_MOUSEMOVE:
|
||||
io.MousePos.x = (signed short)(lParam);
|
||||
io.MousePos.y = (signed short)(lParam >> 16);
|
||||
return true;
|
||||
return 0;
|
||||
case WM_KEYDOWN:
|
||||
case WM_SYSKEYDOWN:
|
||||
if (wParam < 256)
|
||||
io.KeysDown[wParam] = 1;
|
||||
return true;
|
||||
return 0;
|
||||
case WM_KEYUP:
|
||||
case WM_SYSKEYUP:
|
||||
if (wParam < 256)
|
||||
io.KeysDown[wParam] = 0;
|
||||
return true;
|
||||
return 0;
|
||||
case WM_CHAR:
|
||||
// You can also use ToAscii()+GetKeyboardState() to retrieve characters.
|
||||
if (wParam > 0 && wParam < 0x10000)
|
||||
io.AddInputCharacter((unsigned short)wParam);
|
||||
return true;
|
||||
return 0;
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
|
@ -361,6 +386,6 @@ void ImGui_ImplDX9_NewFrame()
|
|||
if (io.MouseDrawCursor)
|
||||
SetCursor(NULL);
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
}
|
||||
|
|
|
@ -12,10 +12,10 @@
|
|||
static LPDIRECT3DDEVICE9 g_pd3dDevice = NULL;
|
||||
static D3DPRESENT_PARAMETERS g_d3dpp;
|
||||
|
||||
extern LRESULT ImGui_ImplDX9_WndProcHandler(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam);
|
||||
extern LRESULT ImGui_ImplWin32_WndProcHandler(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam);
|
||||
LRESULT WINAPI WndProc(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam)
|
||||
{
|
||||
if (ImGui_ImplDX9_WndProcHandler(hWnd, msg, wParam, lParam))
|
||||
if (ImGui_ImplWin32_WndProcHandler(hWnd, msg, wParam, lParam))
|
||||
return true;
|
||||
|
||||
switch (msg)
|
||||
|
@ -78,14 +78,20 @@ int main(int, char**)
|
|||
ImGui_ImplDX9_Init(hwnd, g_pd3dDevice);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
bool show_test_window = true;
|
||||
bool show_another_window = false;
|
||||
|
@ -98,6 +104,10 @@ int main(int, char**)
|
|||
UpdateWindow(hwnd);
|
||||
while (msg.message != WM_QUIT)
|
||||
{
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
if (PeekMessage(&msg, NULL, 0U, 0U, PM_REMOVE))
|
||||
{
|
||||
TranslateMessage(&msg);
|
||||
|
@ -106,8 +116,8 @@ int main(int, char**)
|
|||
}
|
||||
ImGui_ImplDX9_NewFrame();
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// 1. Show a simple window.
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f = 0.0f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -118,7 +128,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -126,7 +136,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver);
|
||||
|
|
Binary file not shown.
Binary file not shown.
|
@ -27,7 +27,7 @@ static char* g_ClipboardText = NULL;
|
|||
static bool g_osdKeyboardEnabled = false;
|
||||
|
||||
// use this setting to scale the interface - e.g. on device you could use 2 or 3 scale factor
|
||||
static ImVec2 g_scale = ImVec2(1.0f,1.0f);
|
||||
static ImVec2 g_RenderScale = ImVec2(1.0f,1.0f);
|
||||
|
||||
// This is the main rendering function that you have to implement and provide to ImGui (via setting up 'RenderDrawListsFn' in the ImGuiIO structure)
|
||||
void ImGui_Marmalade_RenderDrawLists(ImDrawData* draw_data)
|
||||
|
@ -48,9 +48,9 @@ void ImGui_Marmalade_RenderDrawLists(ImDrawData* draw_data)
|
|||
|
||||
for( int i=0; i < nVert; i++ )
|
||||
{
|
||||
// TODO: optimize multiplication on gpu using vertex shader
|
||||
pVertStream[i].x = cmd_list->VtxBuffer[i].pos.x * g_scale.x;
|
||||
pVertStream[i].y = cmd_list->VtxBuffer[i].pos.y * g_scale.y;
|
||||
// TODO: optimize multiplication on gpu using vertex shader/projection matrix.
|
||||
pVertStream[i].x = cmd_list->VtxBuffer[i].pos.x * g_RenderScale.x;
|
||||
pVertStream[i].y = cmd_list->VtxBuffer[i].pos.y * g_RenderScale.y;
|
||||
pUVStream[i].x = cmd_list->VtxBuffer[i].uv.x;
|
||||
pUVStream[i].y = cmd_list->VtxBuffer[i].uv.y;
|
||||
pColStream[i] = cmd_list->VtxBuffer[i].col;
|
||||
|
@ -287,7 +287,7 @@ void ImGui_Marmalade_NewFrame()
|
|||
// TODO: Hide OS mouse cursor if ImGui is drawing it
|
||||
// s3ePointerSetInt(S3E_POINTER_HIDE_CURSOR,(io.MouseDrawCursor ? 0 : 1));
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
|
||||
// Show/hide OSD keyboard
|
||||
|
|
|
@ -18,14 +18,20 @@ int main(int, char**)
|
|||
ImGui_Marmalade_Init(true);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
bool show_test_window = true;
|
||||
bool show_another_window = false;
|
||||
|
@ -37,12 +43,16 @@ int main(int, char**)
|
|||
if (s3eDeviceCheckQuitRequest())
|
||||
break;
|
||||
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
s3eKeyboardUpdate();
|
||||
s3ePointerUpdate();
|
||||
ImGui_Marmalade_NewFrame();
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// 1. Show a simple window.
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f = 0.0f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -53,7 +63,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -61,7 +71,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver);
|
||||
|
|
|
@ -1,10 +1,13 @@
|
|||
// ImGui GLFW binding with OpenGL
|
||||
// In this binding, ImTextureID is used to store an OpenGL 'GLuint' texture identifier. Read the FAQ about ImTextureID in imgui.cpp.
|
||||
// (GLFW is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
|
||||
// If your context or own usage of OpenGL involve anything GL3/GL4, prefer using the code in opengl3_example.
|
||||
// If you are not sure what that means, prefer using the code in opengl3_example.
|
||||
// You *might* use this code with a GL3/GL4 context but make sure you disable the programmable pipeline by calling "glUseProgram(0)" before ImGui::Render().
|
||||
// We cannot do that from GL2 code because the function doesn't exist. Mixing GL2 calls and GL3/GL4 calls is giving trouble to many librairies/drivers.
|
||||
// *DO NOT USE THIS CODE IF YOUR CODE/ENGINE IS USING MODERN OPENGL*
|
||||
// This is mostly provided as a reference to learn how ImGui integration works, because it is easier to read.
|
||||
// If your code is using GL3+ context or any semi modern OpenGL calls, using this is likely to make everything
|
||||
// more complicated, will require your code to reset every single OpenGL attributes to their initial state,
|
||||
// and might confuse your GPU driver. Prefer using opengl3_example.
|
||||
// The GL2 code is unable to reset attributes or even call e.g. "glUseProgram(0)" because they don't exist in that API.
|
||||
|
||||
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
||||
// If you use this binding you'll need to call 4 functions: ImGui_ImplXXXX_Init(), ImGui_ImplXXXX_NewFrame(), ImGui::Render() and ImGui_ImplXXXX_Shutdown().
|
||||
|
@ -26,7 +29,7 @@
|
|||
// Data
|
||||
static GLFWwindow* g_Window = NULL;
|
||||
static double g_Time = 0.0f;
|
||||
static bool g_MousePressed[3] = { false, false, false };
|
||||
static bool g_MouseJustPressed[3] = { false, false, false };
|
||||
static float g_MouseWheel = 0.0f;
|
||||
static GLuint g_FontTexture = 0;
|
||||
|
||||
|
@ -129,12 +132,12 @@ static void ImGui_ImplGlfwGL2_SetClipboardText(void* user_data, const char* text
|
|||
void ImGui_ImplGlfwGL2_MouseButtonCallback(GLFWwindow*, int button, int action, int /*mods*/)
|
||||
{
|
||||
if (action == GLFW_PRESS && button >= 0 && button < 3)
|
||||
g_MousePressed[button] = true;
|
||||
g_MouseJustPressed[button] = true;
|
||||
}
|
||||
|
||||
void ImGui_ImplGlfwGL2_ScrollCallback(GLFWwindow*, double /*xoffset*/, double yoffset)
|
||||
{
|
||||
g_MouseWheel += (float)yoffset; // Use fractional mouse wheel, 1.0 unit 5 lines.
|
||||
g_MouseWheel += (float)yoffset; // Use fractional mouse wheel.
|
||||
}
|
||||
|
||||
void ImGui_ImplGlfwGL2_KeyCallback(GLFWwindow*, int key, int, int action, int mods)
|
||||
|
@ -287,8 +290,9 @@ void ImGui_ImplGlfwGL2_NewFrame()
|
|||
|
||||
for (int i = 0; i < 3; i++)
|
||||
{
|
||||
io.MouseDown[i] = g_MousePressed[i] || glfwGetMouseButton(g_Window, i) != 0; // If a mouse press event came, always pass it as "mouse held this frame", so we don't miss click-release events that are shorter than 1 frame.
|
||||
g_MousePressed[i] = false;
|
||||
// If a mouse press event came, always pass it as "mouse held this frame", so we don't miss click-release events that are shorter than 1 frame.
|
||||
io.MouseDown[i] = g_MouseJustPressed[i] || glfwGetMouseButton(g_Window, i) != 0;
|
||||
g_MouseJustPressed[i] = false;
|
||||
}
|
||||
|
||||
io.MouseWheel = g_MouseWheel;
|
||||
|
@ -297,6 +301,6 @@ void ImGui_ImplGlfwGL2_NewFrame()
|
|||
// Hide OS mouse cursor if ImGui is drawing it
|
||||
glfwSetInputMode(g_Window, GLFW_CURSOR, io.MouseDrawCursor ? GLFW_CURSOR_HIDDEN : GLFW_CURSOR_NORMAL);
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
}
|
||||
|
|
|
@ -1,9 +1,9 @@
|
|||
// ImGui GLFW binding with OpenGL
|
||||
// In this binding, ImTextureID is used to store an OpenGL 'GLuint' texture identifier. Read the FAQ about ImTextureID in imgui.cpp.
|
||||
// (GLFW is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
|
||||
// If your context is GL3/GL3 then prefer using the code in opengl3_example.
|
||||
// You *might* use this code with a GL3/GL4 context but make sure you disable the programmable pipeline by calling "glUseProgram(0)" before ImGui::Render().
|
||||
// We cannot do that from GL2 code because the function doesn't exist.
|
||||
// *DO NOT USE THIS CODE IF YOUR CODE/ENGINE IS USING MODERN OPENGL*
|
||||
// See imgui_impl_glfw.cpp for details.
|
||||
|
||||
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
||||
// If you use this binding you'll need to call 4 functions: ImGui_ImplXXXX_Init(), ImGui_ImplXXXX_NewFrame(), ImGui::Render() and ImGui_ImplXXXX_Shutdown().
|
||||
|
|
|
@ -1,5 +1,9 @@
|
|||
// ImGui - standalone example application for Glfw + OpenGL 2, using fixed pipeline
|
||||
// ImGui - standalone example application for GLFW + OpenGL 2, using fixed pipeline
|
||||
// If you are new to ImGui, see examples/README.txt and documentation at the top of imgui.cpp.
|
||||
// (GLFW is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
|
||||
// *DO NOT USE THIS CODE IF YOUR CODE/ENGINE IS USING MODERN OPENGL*
|
||||
// See imgui_impl_glfw.cpp for details.
|
||||
|
||||
#include <imgui.h>
|
||||
#include "imgui_impl_glfw.h"
|
||||
|
@ -25,14 +29,20 @@ int main(int, char**)
|
|||
ImGui_ImplGlfwGL2_Init(window, true);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
bool show_test_window = true;
|
||||
bool show_another_window = false;
|
||||
|
@ -41,11 +51,15 @@ int main(int, char**)
|
|||
// Main loop
|
||||
while (!glfwWindowShouldClose(window))
|
||||
{
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
glfwPollEvents();
|
||||
ImGui_ImplGlfwGL2_NewFrame();
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// 1. Show a simple window.
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f = 0.0f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -56,7 +70,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -64,7 +78,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver);
|
||||
|
@ -77,7 +91,7 @@ int main(int, char**)
|
|||
glViewport(0, 0, display_w, display_h);
|
||||
glClearColor(clear_color.x, clear_color.y, clear_color.z, clear_color.w);
|
||||
glClear(GL_COLOR_BUFFER_BIT);
|
||||
//glUseProgram(0); // You may want this if using this code in an OpenGL 3+ context where shaders may be bound
|
||||
//glUseProgram(0); // You may want this if using this code in an OpenGL 3+ context where shaders may be bound, but prefer using the GL3+ code.
|
||||
ImGui::Render();
|
||||
glfwSwapBuffers(window);
|
||||
}
|
||||
|
|
|
@ -1,5 +1,7 @@
|
|||
// ImGui GLFW binding with OpenGL3 + shaders
|
||||
// In this binding, ImTextureID is used to store an OpenGL 'GLuint' texture identifier. Read the FAQ about ImTextureID in imgui.cpp.
|
||||
// (GLFW is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
// (GL3W is a helper library to access OpenGL functions since there is no standard header to access modern OpenGL functions easily. Alternatives are GLEW, Glad, etc.)
|
||||
|
||||
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
||||
// If you use this binding you'll need to call 4 functions: ImGui_ImplXXXX_Init(), ImGui_ImplXXXX_NewFrame(), ImGui::Render() and ImGui_ImplXXXX_Shutdown().
|
||||
|
@ -22,7 +24,7 @@
|
|||
// Data
|
||||
static GLFWwindow* g_Window = NULL;
|
||||
static double g_Time = 0.0f;
|
||||
static bool g_MousePressed[3] = { false, false, false };
|
||||
static bool g_MouseJustPressed[3] = { false, false, false };
|
||||
static float g_MouseWheel = 0.0f;
|
||||
static GLuint g_FontTexture = 0;
|
||||
static int g_ShaderHandle = 0, g_VertHandle = 0, g_FragHandle = 0;
|
||||
|
@ -150,12 +152,12 @@ static void ImGui_ImplGlfwGL3_SetClipboardText(void* user_data, const char* text
|
|||
void ImGui_ImplGlfwGL3_MouseButtonCallback(GLFWwindow*, int button, int action, int /*mods*/)
|
||||
{
|
||||
if (action == GLFW_PRESS && button >= 0 && button < 3)
|
||||
g_MousePressed[button] = true;
|
||||
g_MouseJustPressed[button] = true;
|
||||
}
|
||||
|
||||
void ImGui_ImplGlfwGL3_ScrollCallback(GLFWwindow*, double /*xoffset*/, double yoffset)
|
||||
{
|
||||
g_MouseWheel += (float)yoffset; // Use fractional mouse wheel, 1.0 unit 5 lines.
|
||||
g_MouseWheel += (float)yoffset; // Use fractional mouse wheel.
|
||||
}
|
||||
|
||||
void ImGui_ImplGlfwGL3_KeyCallback(GLFWwindow*, int key, int, int action, int mods)
|
||||
|
@ -401,8 +403,9 @@ void ImGui_ImplGlfwGL3_NewFrame()
|
|||
|
||||
for (int i = 0; i < 3; i++)
|
||||
{
|
||||
io.MouseDown[i] = g_MousePressed[i] || glfwGetMouseButton(g_Window, i) != 0; // If a mouse press event came, always pass it as "mouse held this frame", so we don't miss click-release events that are shorter than 1 frame.
|
||||
g_MousePressed[i] = false;
|
||||
// If a mouse press event came, always pass it as "mouse held this frame", so we don't miss click-release events that are shorter than 1 frame.
|
||||
io.MouseDown[i] = g_MouseJustPressed[i] || glfwGetMouseButton(g_Window, i) != 0;
|
||||
g_MouseJustPressed[i] = false;
|
||||
}
|
||||
|
||||
io.MouseWheel = g_MouseWheel;
|
||||
|
@ -411,6 +414,6 @@ void ImGui_ImplGlfwGL3_NewFrame()
|
|||
// Hide OS mouse cursor if ImGui is drawing it
|
||||
glfwSetInputMode(g_Window, GLFW_CURSOR, io.MouseDrawCursor ? GLFW_CURSOR_HIDDEN : GLFW_CURSOR_NORMAL);
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
}
|
||||
|
|
|
@ -1,5 +1,7 @@
|
|||
// ImGui GLFW binding with OpenGL3 + shaders
|
||||
// In this binding, ImTextureID is used to store an OpenGL 'GLuint' texture identifier. Read the FAQ about ImTextureID in imgui.cpp.
|
||||
// (GLFW is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
// (GL3W is a helper library to access OpenGL functions since there is no standard header to access modern OpenGL functions easily. Alternatives are GLEW, Glad, etc.)
|
||||
|
||||
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
||||
// If you use this binding you'll need to call 4 functions: ImGui_ImplXXXX_Init(), ImGui_ImplXXXX_NewFrame(), ImGui::Render() and ImGui_ImplXXXX_Shutdown().
|
||||
|
|
|
@ -1,5 +1,7 @@
|
|||
// ImGui - standalone example application for Glfw + OpenGL 3, using programmable pipeline
|
||||
// ImGui - standalone example application for GLFW + OpenGL 3, using programmable pipeline
|
||||
// If you are new to ImGui, see examples/README.txt and documentation at the top of imgui.cpp.
|
||||
// (GLFW is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
// (GL3W is a helper library to access OpenGL functions since there is no standard header to access modern OpenGL functions easily. Alternatives are GLEW, Glad, etc.)
|
||||
|
||||
#include <imgui.h>
|
||||
#include "imgui_impl_glfw_gl3.h"
|
||||
|
@ -33,14 +35,20 @@ int main(int, char**)
|
|||
ImGui_ImplGlfwGL3_Init(window, true);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
bool show_test_window = true;
|
||||
bool show_another_window = false;
|
||||
|
@ -49,11 +57,15 @@ int main(int, char**)
|
|||
// Main loop
|
||||
while (!glfwWindowShouldClose(window))
|
||||
{
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
glfwPollEvents();
|
||||
ImGui_ImplGlfwGL3_NewFrame();
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// 1. Show a simple window.
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f = 0.0f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -64,7 +76,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -72,7 +84,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver);
|
||||
|
|
|
@ -1,8 +1,13 @@
|
|||
// ImGui SDL2 binding with OpenGL
|
||||
// In this binding, ImTextureID is used to store an OpenGL 'GLuint' texture identifier. Read the FAQ about ImTextureID in imgui.cpp.
|
||||
// (SDL is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
|
||||
// If your context or own usage of OpenGL involve anything GL3/GL4, prefer using the code in sdl_opengl3_example.
|
||||
// If you are not sure what that means, prefer using the code in sdl_opengl3_example.
|
||||
// *DO NOT USE THIS CODE IF YOUR CODE/ENGINE IS USING MODERN OPENGL*
|
||||
// This is mostly provided as a reference to learn how ImGui integration works, because it is easier to read.
|
||||
// If your code is using GL3+ context or any semi modern OpenGL calls, using this is likely to make everything
|
||||
// more complicated, will require your code to reset every single OpenGL attributes to their initial state,
|
||||
// and might confuse your GPU driver. Prefer using sdl_opengl3_example.
|
||||
// The GL2 code is unable to reset attributes or even call e.g. "glUseProgram(0)" because they don't exist in that API.
|
||||
|
||||
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
||||
// If you use this binding you'll need to call 4 functions: ImGui_ImplXXXX_Init(), ImGui_ImplXXXX_NewFrame(), ImGui::Render() and ImGui_ImplXXXX_Shutdown().
|
||||
|
@ -117,6 +122,10 @@ static void ImGui_ImplSdl_SetClipboardText(void*, const char* text)
|
|||
SDL_SetClipboardText(text);
|
||||
}
|
||||
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
bool ImGui_ImplSdlGL2_ProcessEvent(SDL_Event* event)
|
||||
{
|
||||
ImGuiIO& io = ImGui::GetIO();
|
||||
|
@ -281,6 +290,6 @@ void ImGui_ImplSdlGL2_NewFrame(SDL_Window *window)
|
|||
// Hide OS mouse cursor if ImGui is drawing it
|
||||
SDL_ShowCursor(io.MouseDrawCursor ? 0 : 1);
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
}
|
||||
|
|
|
@ -1,5 +1,6 @@
|
|||
// ImGui SDL2 binding with OpenGL
|
||||
// In this binding, ImTextureID is used to store an OpenGL 'GLuint' texture identifier. Read the FAQ about ImTextureID in imgui.cpp.
|
||||
// (SDL is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
|
||||
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
||||
// If you use this binding you'll need to call 4 functions: ImGui_ImplXXXX_Init(), ImGui_ImplXXXX_NewFrame(), ImGui::Render() and ImGui_ImplXXXX_Shutdown().
|
||||
|
|
|
@ -1,5 +1,9 @@
|
|||
// ImGui - standalone example application for SDL2 + OpenGL
|
||||
// If you are new to ImGui, see examples/README.txt and documentation at the top of imgui.cpp.
|
||||
// (SDL is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
|
||||
// *DO NOT USE THIS CODE IF YOUR CODE/ENGINE IS USING MODERN OPENGL*
|
||||
// See imgui_impl_sdl.cpp for details.
|
||||
|
||||
#include <imgui.h>
|
||||
#include "imgui_impl_sdl.h"
|
||||
|
@ -31,14 +35,20 @@ int main(int, char**)
|
|||
ImGui_ImplSdlGL2_Init(window);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
bool show_test_window = true;
|
||||
bool show_another_window = false;
|
||||
|
@ -48,6 +58,10 @@ int main(int, char**)
|
|||
bool done = false;
|
||||
while (!done)
|
||||
{
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
SDL_Event event;
|
||||
while (SDL_PollEvent(&event))
|
||||
{
|
||||
|
@ -58,7 +72,7 @@ int main(int, char**)
|
|||
ImGui_ImplSdlGL2_NewFrame(window);
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f = 0.0f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -69,7 +83,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -77,7 +91,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver);
|
||||
|
|
|
@ -1,5 +1,7 @@
|
|||
// ImGui SDL2 binding with OpenGL3
|
||||
// In this binding, ImTextureID is used to store an OpenGL 'GLuint' texture identifier. Read the FAQ about ImTextureID in imgui.cpp.
|
||||
// (SDL is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
// (GL3W is a helper library to access OpenGL functions since there is no standard header to access modern OpenGL functions easily. Alternatives are GLEW, Glad, etc.)
|
||||
|
||||
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
||||
// If you use this binding you'll need to call 4 functions: ImGui_ImplXXXX_Init(), ImGui_ImplXXXX_NewFrame(), ImGui::Render() and ImGui_ImplXXXX_Shutdown().
|
||||
|
@ -141,6 +143,10 @@ static void ImGui_ImplSdlGL3_SetClipboardText(void*, const char* text)
|
|||
SDL_SetClipboardText(text);
|
||||
}
|
||||
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
bool ImGui_ImplSdlGL3_ProcessEvent(SDL_Event* event)
|
||||
{
|
||||
ImGuiIO& io = ImGui::GetIO();
|
||||
|
@ -396,6 +402,6 @@ void ImGui_ImplSdlGL3_NewFrame(SDL_Window* window)
|
|||
// Hide OS mouse cursor if ImGui is drawing it
|
||||
SDL_ShowCursor(io.MouseDrawCursor ? 0 : 1);
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
}
|
||||
|
|
|
@ -1,5 +1,7 @@
|
|||
// ImGui SDL2 binding with OpenGL3
|
||||
// In this binding, ImTextureID is used to store an OpenGL 'GLuint' texture identifier. Read the FAQ about ImTextureID in imgui.cpp.
|
||||
// (SDL is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
// (GL3W is a helper library to access OpenGL functions since there is no standard header to access modern OpenGL functions easily. Alternatives are GLEW, Glad, etc.)
|
||||
|
||||
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
||||
// If you use this binding you'll need to call 4 functions: ImGui_ImplXXXX_Init(), ImGui_ImplXXXX_NewFrame(), ImGui::Render() and ImGui_ImplXXXX_Shutdown().
|
||||
|
|
|
@ -1,5 +1,7 @@
|
|||
// ImGui - standalone example application for SDL2 + OpenGL
|
||||
// If you are new to ImGui, see examples/README.txt and documentation at the top of imgui.cpp.
|
||||
// (SDL is a cross-platform general purpose library for handling windows, inputs, OpenGL/Vulkan graphics context creation, etc.)
|
||||
// (GL3W is a helper library to access OpenGL functions since there is no standard header to access modern OpenGL functions easily. Alternatives are GLEW, Glad, etc.)
|
||||
|
||||
#include <imgui.h>
|
||||
#include "imgui_impl_sdl_gl3.h"
|
||||
|
@ -34,14 +36,20 @@ int main(int, char**)
|
|||
ImGui_ImplSdlGL3_Init(window);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
bool show_test_window = true;
|
||||
bool show_another_window = false;
|
||||
|
@ -51,6 +59,10 @@ int main(int, char**)
|
|||
bool done = false;
|
||||
while (!done)
|
||||
{
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
SDL_Event event;
|
||||
while (SDL_PollEvent(&event))
|
||||
{
|
||||
|
@ -60,8 +72,8 @@ int main(int, char**)
|
|||
}
|
||||
ImGui_ImplSdlGL3_NewFrame(window);
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// 1. Show a simple window.
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f = 0.0f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -72,7 +84,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -80,7 +92,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver);
|
||||
|
|
|
@ -0,0 +1,36 @@
|
|||
cmake_minimum_required(VERSION 2.8)
|
||||
project(ImGuiGLFWVulkanExample C CXX)
|
||||
|
||||
if(NOT CMAKE_BUILD_TYPE)
|
||||
set(CMAKE_BUILD_TYPE Debug CACHE STRING "" FORCE)
|
||||
endif()
|
||||
|
||||
set (CMAKE_C_FLAGS "${CMAKE_C_FLAGS} -DVK_PROTOTYPES")
|
||||
set (CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -DVK_PROTOTYPES")
|
||||
|
||||
# GLFW
|
||||
set(GLFW_DIR ../../../glfw) # Set this to point to an up-to-date GLFW repo
|
||||
option(GLFW_BUILD_EXAMPLES "Build the GLFW example programs" OFF)
|
||||
option(GLFW_BUILD_TESTS "Build the GLFW test programs" OFF)
|
||||
option(GLFW_BUILD_DOCS "Build the GLFW documentation" OFF)
|
||||
option(GLFW_INSTALL "Generate installation target" OFF)
|
||||
option(GLFW_DOCUMENT_INTERNALS "Include internals in documentation" OFF)
|
||||
add_subdirectory(${GLFW_DIR} binary_dir EXCLUDE_FROM_ALL)
|
||||
include_directories(${GLFW_DIR}/include)
|
||||
|
||||
# ImGui
|
||||
set(IMGUI_DIR ../../)
|
||||
include_directories(${IMGUI_DIR})
|
||||
|
||||
# Libraries
|
||||
find_library(VULKAN_LIBRARY
|
||||
NAMES vulkan vulkan-1)
|
||||
set(LIBRARIES "glfw;${VULKAN_LIBRARY}")
|
||||
|
||||
# Use vulkan headers from glfw:
|
||||
include_directories(${GLFW_DIR}/deps)
|
||||
|
||||
file(GLOB sources *.cpp)
|
||||
|
||||
add_executable(vulkan_example ${sources} ${IMGUI_DIR}/imgui.cpp ${IMGUI_DIR}/imgui_draw.cpp ${IMGUI_DIR}/imgui_demo.cpp)
|
||||
target_link_libraries(vulkan_example ${LIBRARIES})
|
|
@ -332,7 +332,7 @@ void ImGui_ImplGlfwVulkan_MouseButtonCallback(GLFWwindow*, int button, int actio
|
|||
|
||||
void ImGui_ImplGlfwVulkan_ScrollCallback(GLFWwindow*, double /*xoffset*/, double yoffset)
|
||||
{
|
||||
g_MouseWheel += (float)yoffset; // Use fractional mouse wheel, 1.0 unit 5 lines.
|
||||
g_MouseWheel += (float)yoffset; // Use fractional mouse wheel.
|
||||
}
|
||||
|
||||
void ImGui_ImplGlfwVulkan_KeyCallback(GLFWwindow*, int key, int, int action, int mods)
|
||||
|
@ -830,7 +830,7 @@ void ImGui_ImplGlfwVulkan_NewFrame()
|
|||
// Hide OS mouse cursor if ImGui is drawing it
|
||||
glfwSetInputMode(g_Window, GLFW_CURSOR, io.MouseDrawCursor ? GLFW_CURSOR_HIDDEN : GLFW_CURSOR_NORMAL);
|
||||
|
||||
// Start the frame
|
||||
// Start the frame. This call will update the io.WantCaptureMouse, io.WantCaptureKeyboard flag that you can use to dispatch inputs (or not) to your application.
|
||||
ImGui::NewFrame();
|
||||
}
|
||||
|
||||
|
|
|
@ -626,14 +626,20 @@ int main(int, char**)
|
|||
ImGui_ImplGlfwVulkan_Init(window, true, &init_data);
|
||||
|
||||
// Load Fonts
|
||||
// (there is a default font, this is only if you want to change it. see extra_fonts/README.txt for more details)
|
||||
// - If no fonts are loaded, dear imgui will use the default font. You can also load multiple fonts and use ImGui::PushFont()/PopFont() to select them.
|
||||
// - AddFontFromFileTTF() will return the ImFont* so you can store it if you need to select the font among multiple.
|
||||
// - If the file cannot be loaded, the function will return NULL. Please handle those errors in your application (e.g. use an assertion, or display an error and quit).
|
||||
// - The fonts will be rasterized at a given size (w/ oversampling) and stored into a texture when calling ImFontAtlas::Build()/GetTexDataAsXXXX(), which ImGui_ImplXXXX_NewFrame below will call.
|
||||
// - Read 'extra_fonts/README.txt' for more instructions and details.
|
||||
// - Remember that in C/C++ if you want to include a backslash \ in a string literal you need to write a double backslash \\ !
|
||||
//ImGuiIO& io = ImGui::GetIO();
|
||||
//io.Fonts->AddFontDefault();
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Cousine-Regular.ttf", 15.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/DroidSans.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/Roboto-Medium.ttf", 16.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("../../extra_fonts/ProggyTiny.ttf", 10.0f);
|
||||
//io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//ImFont* font = io.Fonts->AddFontFromFileTTF("c:\\Windows\\Fonts\\ArialUni.ttf", 18.0f, NULL, io.Fonts->GetGlyphRangesJapanese());
|
||||
//IM_ASSERT(font != NULL);
|
||||
|
||||
// Upload Fonts
|
||||
{
|
||||
|
@ -680,11 +686,15 @@ int main(int, char**)
|
|||
// Main loop
|
||||
while (!glfwWindowShouldClose(window))
|
||||
{
|
||||
// You can read the io.WantCaptureMouse, io.WantCaptureKeyboard flags to tell if dear imgui wants to use your inputs.
|
||||
// - When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application.
|
||||
// - When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application.
|
||||
// Generally you may always pass all inputs to dear imgui, and hide them from your application based on those two flags.
|
||||
glfwPollEvents();
|
||||
ImGui_ImplGlfwVulkan_NewFrame();
|
||||
|
||||
// 1. Show a simple window
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug"
|
||||
// 1. Show a simple window.
|
||||
// Tip: if we don't call ImGui::Begin()/ImGui::End() the widgets appears in a window automatically called "Debug".
|
||||
{
|
||||
static float f = 0.0f;
|
||||
ImGui::Text("Hello, world!");
|
||||
|
@ -695,7 +705,7 @@ int main(int, char**)
|
|||
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
|
||||
}
|
||||
|
||||
// 2. Show another simple window, this time using an explicit Begin/End pair
|
||||
// 2. Show another simple window. In most cases you will use an explicit Begin/End pair to name the window.
|
||||
if (show_another_window)
|
||||
{
|
||||
ImGui::Begin("Another Window", &show_another_window);
|
||||
|
@ -703,7 +713,7 @@ int main(int, char**)
|
|||
ImGui::End();
|
||||
}
|
||||
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow()
|
||||
// 3. Show the ImGui test window. Most of the sample code is in ImGui::ShowTestWindow().
|
||||
if (show_test_window)
|
||||
{
|
||||
ImGui::SetNextWindowPos(ImVec2(650, 20), ImGuiCond_FirstUseEver);
|
||||
|
|
|
@ -13,24 +13,27 @@
|
|||
//#define IMGUI_API __declspec( dllexport )
|
||||
//#define IMGUI_API __declspec( dllimport )
|
||||
|
||||
//---- Don't define obsolete functions names. Consider enabling from time to time or when updating to reduce like hood of using already obsolete function/names
|
||||
//#define IMGUI_DISABLE_OBSOLETE_FUNCTIONS
|
||||
|
||||
//---- Include imgui_user.h at the end of imgui.h
|
||||
//#define IMGUI_INCLUDE_IMGUI_USER_H
|
||||
|
||||
//---- Don't implement default handlers for Windows (so as not to link with OpenClipboard() and others Win32 functions)
|
||||
//#define IMGUI_DISABLE_WIN32_DEFAULT_CLIPBOARD_FUNCS
|
||||
//#define IMGUI_DISABLE_WIN32_DEFAULT_IME_FUNCS
|
||||
//#define IMGUI_DISABLE_WIN32_DEFAULT_CLIPBOARD_FUNCTIONS
|
||||
//#define IMGUI_DISABLE_WIN32_DEFAULT_IME_FUNCTIONS
|
||||
|
||||
//---- Don't implement test window functionality (ShowTestWindow()/ShowStyleEditor()/ShowUserGuide() methods will be empty)
|
||||
//---- It is very strongly recommended to NOT disable the test windows. Please read the comment at the top of imgui_demo.cpp to learn why.
|
||||
//#define IMGUI_DISABLE_TEST_WINDOWS
|
||||
|
||||
//---- Don't define obsolete functions names. Consider enabling from time to time or when updating to reduce like hood of using already obsolete function/names
|
||||
//#define IMGUI_DISABLE_OBSOLETE_FUNCTIONS
|
||||
//---- Don't implement ImFormatString(), ImFormatStringV() so you can reimplement them yourself.
|
||||
//#define IMGUI_DISABLE_FORMAT_STRING_FUNCTIONS
|
||||
|
||||
//---- Pack colors to BGRA instead of RGBA (remove need to post process vertex buffer in back ends)
|
||||
//#define IMGUI_USE_BGRA_PACKED_COLOR
|
||||
|
||||
//---- Implement STB libraries in a namespace to avoid conflicts
|
||||
//---- Implement STB libraries in a namespace to avoid linkage conflicts
|
||||
//#define IMGUI_STB_NAMESPACE ImGuiStb
|
||||
|
||||
//---- Define constructor and implicit cast operators to convert back<>forth from your math types and ImVec2/ImVec4.
|
||||
|
|
File diff suppressed because it is too large
Load Diff
|
@ -1,4 +1,4 @@
|
|||
// dear imgui, v1.52 WIP
|
||||
// dear imgui, v1.53 WIP
|
||||
// (headers)
|
||||
|
||||
// See imgui.cpp file for documentation.
|
||||
|
@ -16,7 +16,8 @@
|
|||
#include <stddef.h> // ptrdiff_t, NULL
|
||||
#include <string.h> // memset, memmove, memcpy, strlen, strchr, strcpy, strcmp
|
||||
|
||||
#define IMGUI_VERSION "1.52 WIP"
|
||||
#define IMGUI_VERSION "1.53 WIP"
|
||||
#define IMGUI_HAS_NAV // navigation branch
|
||||
|
||||
// Define attributes of all API symbols declarations, e.g. for DLL under Windows.
|
||||
#ifndef IMGUI_API
|
||||
|
@ -29,14 +30,16 @@
|
|||
#define IM_ASSERT(_EXPR) assert(_EXPR)
|
||||
#endif
|
||||
|
||||
// Helpers
|
||||
// Some compilers support applying printf-style warnings to user functions.
|
||||
#if defined(__clang__) || defined(__GNUC__)
|
||||
#define IM_FMTARGS(FMT) __attribute__((format(printf, FMT, FMT+1)))
|
||||
#define IM_FMTLIST(FMT) __attribute__((format(printf, FMT, 0)))
|
||||
#define IM_FMTARGS(FMT) __attribute__((format(printf, FMT, FMT+1)))
|
||||
#define IM_FMTLIST(FMT) __attribute__((format(printf, FMT, 0)))
|
||||
#else
|
||||
#define IM_FMTARGS(FMT)
|
||||
#define IM_FMTLIST(FMT)
|
||||
#endif
|
||||
#define IM_ARRAYSIZE(_ARR) ((int)(sizeof(_ARR)/sizeof(*_ARR)))
|
||||
|
||||
#if defined(__clang__)
|
||||
#pragma clang diagnostic push
|
||||
|
@ -81,6 +84,7 @@ typedef int ImGuiColumnsFlags; // flags: for *Columns*()
|
|||
typedef int ImGuiInputTextFlags; // flags: for InputText*() // enum ImGuiInputTextFlags_
|
||||
typedef int ImGuiSelectableFlags; // flags: for Selectable() // enum ImGuiSelectableFlags_
|
||||
typedef int ImGuiTreeNodeFlags; // flags: for TreeNode*(), Collapsing*() // enum ImGuiTreeNodeFlags_
|
||||
typedef int ImGuiHoveredFlags; // flags: for IsItemHovered() // enum ImGuiHoveredFlags_
|
||||
typedef int (*ImGuiTextEditCallback)(ImGuiTextEditCallbackData *data);
|
||||
typedef void (*ImGuiSizeConstraintCallback)(ImGuiSizeConstraintCallbackData* data);
|
||||
#ifdef _MSC_VER
|
||||
|
@ -127,13 +131,12 @@ namespace ImGui
|
|||
|
||||
// Demo/Debug/Info
|
||||
IMGUI_API void ShowTestWindow(bool* p_open = NULL); // create demo/test window. demonstrate most ImGui features. call this to learn about the library! try to make it always available in your application!
|
||||
IMGUI_API void ShowMetricsWindow(bool* p_open = NULL); // create metrics window. display ImGui internals: browse window list, draw commands, individual vertices, basic internal state, etc.
|
||||
IMGUI_API void ShowMetricsWindow(bool* p_open = NULL); // create metrics window. display ImGui internals: draw commands (with individual draw calls and vertices), window list, basic internal state, etc.
|
||||
IMGUI_API void ShowStyleEditor(ImGuiStyle* ref = NULL); // add style editor block (not a window). you can pass in a reference ImGuiStyle structure to compare to, revert to and save to (else it uses the default style)
|
||||
IMGUI_API void ShowUserGuide(); // add basic help/info block (not a window): how to manipulate ImGui as a end-user (mouse/keyboard controls).
|
||||
|
||||
// Window
|
||||
IMGUI_API bool Begin(const char* name, bool* p_open = NULL, ImGuiWindowFlags flags = 0); // push window to the stack and start appending to it. see .cpp for details. return false when window is collapsed, so you can early out in your code. 'bool* p_open' creates a widget on the upper-right to close the window (which sets your bool to false).
|
||||
IMGUI_API bool Begin(const char* name, bool* p_open, const ImVec2& size_on_first_use, float bg_alpha = -1.0f, ImGuiWindowFlags flags = 0); // OBSOLETE. this is the older/longer API. the extra parameters aren't very relevant. call SetNextWindowSize() instead if you want to set a window size. For regular windows, 'size_on_first_use' only applies to the first time EVER the window is created and probably not what you want! might obsolete this API eventually.
|
||||
IMGUI_API void End(); // finish appending to current window, pop it off the window stack.
|
||||
IMGUI_API bool BeginChild(const char* str_id, const ImVec2& size = ImVec2(0,0), bool border = false, ImGuiWindowFlags extra_flags = 0); // begin a scrolling region. size==0.0f: use remaining window size, size<0.0f: use remaining window size minus abs(size). size>0.0f: fixed size. each axis can use a different mode, e.g. ImVec2(0,400).
|
||||
IMGUI_API bool BeginChild(ImGuiID id, const ImVec2& size = ImVec2(0,0), bool border = false, ImGuiWindowFlags extra_flags = 0); // "
|
||||
|
@ -207,6 +210,8 @@ namespace ImGui
|
|||
IMGUI_API void PopAllowKeyboardFocus();
|
||||
IMGUI_API void PushButtonRepeat(bool repeat); // in 'repeat' mode, Button*() functions return repeated true in a typematic manner (using io.KeyRepeatDelay/io.KeyRepeatRate setting). Note that you can call IsItemActive() after any Button() to tell if the button is held in the current frame.
|
||||
IMGUI_API void PopButtonRepeat();
|
||||
IMGUI_API void PushNavDefaultFocus(bool default_focus);
|
||||
IMGUI_API void PopNavDefaultFocus();
|
||||
|
||||
// Cursor / Layout
|
||||
IMGUI_API void Separator(); // separator, generally horizontal. inside a menu bar or in horizontal layout mode, this becomes a vertical separator.
|
||||
|
@ -227,7 +232,7 @@ namespace ImGui
|
|||
IMGUI_API ImVec2 GetCursorStartPos(); // initial cursor position
|
||||
IMGUI_API ImVec2 GetCursorScreenPos(); // cursor position in absolute screen coordinates [0..io.DisplaySize] (useful to work with ImDrawList API)
|
||||
IMGUI_API void SetCursorScreenPos(const ImVec2& pos); // cursor position in absolute screen coordinates [0..io.DisplaySize]
|
||||
IMGUI_API void AlignFirstTextHeightToWidgets(); // call once if the first item on the line is a Text() item and you want to vertically lower it to match subsequent (bigger) widgets
|
||||
IMGUI_API void AlignTextToFramePadding(); // vertically align/lower upcoming text to FramePadding.y so that it will aligns to upcoming widgets (call if you have text on a line before regular widgets)
|
||||
IMGUI_API float GetTextLineHeight(); // height of font == GetWindowFontSize()
|
||||
IMGUI_API float GetTextLineHeightWithSpacing(); // distance (in pixels) between 2 consecutive lines of text == GetWindowFontSize() + GetStyle().ItemSpacing.y
|
||||
IMGUI_API float GetItemsLineHeightWithSpacing(); // distance (in pixels) between 2 consecutive lines of standard height widgets == GetWindowFontSize() + GetStyle().FramePadding.y*2 + GetStyle().ItemSpacing.y
|
||||
|
@ -391,11 +396,12 @@ namespace ImGui
|
|||
|
||||
// Popups
|
||||
IMGUI_API void OpenPopup(const char* str_id); // call to mark popup as open (don't call every frame!). popups are closed when user click outside, or if CloseCurrentPopup() is called within a BeginPopup()/EndPopup() block. By default, Selectable()/MenuItem() are calling CloseCurrentPopup(). Popup identifiers are relative to the current ID-stack (so OpenPopup and BeginPopup needs to be at the same level).
|
||||
IMGUI_API bool OpenPopupOnItemClick(const char* str_id = NULL, int mouse_button = 1); // helper to open popup when clicked on last item. return true when just opened.
|
||||
IMGUI_API bool BeginPopup(const char* str_id); // return true if the popup is open, and you can start outputting to it. only call EndPopup() if BeginPopup() returned true!
|
||||
IMGUI_API bool BeginPopupModal(const char* name, bool* p_open = NULL, ImGuiWindowFlags extra_flags = 0); // modal dialog (block interactions behind the modal window, can't close the modal window by clicking outside)
|
||||
IMGUI_API bool BeginPopupContextItem(const char* str_id, int mouse_button = 1); // helper to open and begin popup when clicked on last item. read comments in .cpp!
|
||||
IMGUI_API bool BeginPopupContextItem(const char* str_id = NULL, int mouse_button = 1); // helper to open and begin popup when clicked on last item. if you can pass a NULL str_id only if the previous item had an id. If you want to use that on a non-interactive item such as Text() you need to pass in an explicit ID here. read comments in .cpp!
|
||||
IMGUI_API bool BeginPopupContextWindow(const char* str_id = NULL, int mouse_button = 1, bool also_over_items = true); // helper to open and begin popup when clicked on current window.
|
||||
IMGUI_API bool BeginPopupContextVoid(const char* str_id = NULL, int mouse_button = 1); // helper to open and begin popup when clicked in void (no window).
|
||||
IMGUI_API bool BeginPopupContextVoid(const char* str_id = NULL, int mouse_button = 1); // helper to open and begin popup when clicked in void (where there are no imgui windows).
|
||||
IMGUI_API void EndPopup();
|
||||
IMGUI_API bool IsPopupOpen(const char* str_id); // return true if the popup is open
|
||||
IMGUI_API void CloseCurrentPopup(); // close the popup we have begin-ed into. clicking on a MenuItem or Selectable automatically close the current popup.
|
||||
|
@ -414,6 +420,7 @@ namespace ImGui
|
|||
|
||||
// Styles
|
||||
IMGUI_API void StyleColorsClassic(ImGuiStyle* dst = NULL);
|
||||
IMGUI_API void StyleColorsDark(ImGuiStyle* dst = NULL);
|
||||
|
||||
// Focus, Activation
|
||||
IMGUI_API void ActivateItem(ImGuiID id); // remotely activate a button, checkbox, tree node etc. given its unique ID. activation is queued and processed on the next frame when the item is encountered again.
|
||||
|
@ -422,8 +429,7 @@ namespace ImGui
|
|||
IMGUI_API void SetItemDefaultFocus(); // FIXME-NAVIGATION // make last item the default focused item of a window
|
||||
|
||||
// Utilities
|
||||
IMGUI_API bool IsItemHovered(); // is the last item hovered by mouse (and usable)? or we are currently using Nav and the item is focused.
|
||||
IMGUI_API bool IsItemRectHovered(); // is the last item hovered by mouse? even if another item is active or window is blocked by popup while we are hovering this
|
||||
IMGUI_API bool IsItemHovered(ImGuiHoveredFlags flags = 0); // is the last item hovered by mouse (and usable)? or we are currently using Nav and the item is focused.
|
||||
IMGUI_API bool IsItemActive(); // is the last item active? (e.g. button being held, text field being edited- items that don't interact will always return false)
|
||||
IMGUI_API bool IsItemFocused(); // is the last item focused for keyboard/gamepad navigation?
|
||||
IMGUI_API bool IsItemClicked(int mouse_button = 0); // is the last item clicked? (e.g. button/node just clicked on)
|
||||
|
@ -435,12 +441,11 @@ namespace ImGui
|
|||
IMGUI_API ImVec2 GetItemRectMax(); // "
|
||||
IMGUI_API ImVec2 GetItemRectSize(); // "
|
||||
IMGUI_API void SetItemAllowOverlap(); // allow last item to be overlapped by a subsequent item. sometimes useful with invisible buttons, selectables, etc. to catch unused area.
|
||||
IMGUI_API bool IsWindowFocused(); // is current window focused
|
||||
IMGUI_API bool IsWindowHovered(); // is current window hovered and hoverable (not blocked by a popup) (differentiate child windows from each others)
|
||||
IMGUI_API bool IsWindowRectHovered(); // is current window rectangle hovered, disregarding of any consideration of being blocked by a popup. (unlike IsWindowHovered() this will return true even if the window is blocked because of a popup)
|
||||
IMGUI_API bool IsRootWindowFocused(); // is current root window focused (root = top-most parent of a child, otherwise self)
|
||||
IMGUI_API bool IsRootWindowOrAnyChildFocused(); // is current root window or any of its child (including current window) focused
|
||||
IMGUI_API bool IsRootWindowOrAnyChildHovered(); // is current root window or any of its child (including current window) hovered and hoverable (not blocked by a popup)
|
||||
IMGUI_API bool IsWindowFocused(); // is current Begin()-ed window focused?
|
||||
IMGUI_API bool IsWindowHovered(ImGuiHoveredFlags flags = 0); // is current Begin()-ed window hovered (and typically: not blocked by a popup/modal)?
|
||||
IMGUI_API bool IsRootWindowFocused(); // is current Begin()-ed root window focused (root = top-most parent of a child, otherwise self)?
|
||||
IMGUI_API bool IsRootWindowOrAnyChildFocused(); // is current Begin()-ed root window or any of its child (including current window) focused?
|
||||
IMGUI_API bool IsRootWindowOrAnyChildHovered(ImGuiHoveredFlags flags = 0); // is current Begin()-ed root window or any of its child (including current window) hovered and hoverable (not blocked by a popup)?
|
||||
IMGUI_API bool IsAnyWindowFocused();
|
||||
IMGUI_API bool IsAnyWindowHovered(); // is mouse hovering any visible window
|
||||
IMGUI_API bool IsRectVisible(const ImVec2& size); // test if rectangle (of given size, starting from cursor position) is visible / not clipped.
|
||||
|
@ -496,19 +501,6 @@ namespace ImGui
|
|||
IMGUI_API ImGuiContext* GetCurrentContext();
|
||||
IMGUI_API void SetCurrentContext(ImGuiContext* ctx);
|
||||
|
||||
// Obsolete functions (Will be removed! Also see 'API BREAKING CHANGES' section in imgui.cpp)
|
||||
#ifndef IMGUI_DISABLE_OBSOLETE_FUNCTIONS
|
||||
void SetNextWindowPosCenter(ImGuiCond cond = 0); // OBSOLETE 1.52+
|
||||
static inline bool IsItemHoveredRect() { return IsItemRectHovered(); } // OBSOLETE 1.51+
|
||||
static inline bool IsPosHoveringAnyWindow(const ImVec2&) { IM_ASSERT(0); return false; } // OBSOLETE 1.51+. This was partly broken. You probably wanted to use ImGui::GetIO().WantCaptureMouse instead.
|
||||
static inline bool IsMouseHoveringAnyWindow() { return IsAnyWindowHovered(); } // OBSOLETE 1.51+
|
||||
static inline bool IsMouseHoveringWindow() { return IsWindowRectHovered(); } // OBSOLETE 1.51+
|
||||
static inline bool CollapsingHeader(const char* label, const char* str_id, bool framed = true, bool default_open = false) { (void)str_id; (void)framed; ImGuiTreeNodeFlags default_open_flags = 1<<5; return CollapsingHeader(label, (default_open ? default_open_flags : 0)); } // OBSOLETE 1.49+
|
||||
static inline ImFont* GetWindowFont() { return GetFont(); } // OBSOLETE 1.48+
|
||||
static inline float GetWindowFontSize() { return GetFontSize(); } // OBSOLETE 1.48+
|
||||
static inline void SetScrollPosHere() { SetScrollHere(); } // OBSOLETE 1.42+
|
||||
#endif
|
||||
|
||||
} // namespace ImGui
|
||||
|
||||
// Flags for ImGui::Begin()
|
||||
|
@ -524,7 +516,7 @@ enum ImGuiWindowFlags_
|
|||
ImGuiWindowFlags_AlwaysAutoResize = 1 << 6, // Resize every window to its content every frame
|
||||
ImGuiWindowFlags_ShowBorders = 1 << 7, // Show borders around windows and items
|
||||
ImGuiWindowFlags_NoSavedSettings = 1 << 8, // Never load/save settings in .ini file
|
||||
ImGuiWindowFlags_NoInputs = 1 << 9, // Disable catching mouse or keyboard inputs
|
||||
ImGuiWindowFlags_NoInputs = 1 << 9, // Disable catching mouse or keyboard inputs, hovering test with pass through.
|
||||
ImGuiWindowFlags_MenuBar = 1 << 10, // Has a menu-bar
|
||||
ImGuiWindowFlags_HorizontalScrollbar = 1 << 11, // Allow horizontal scrollbar to appear (off by default). You may use SetNextWindowContentSize(ImVec2(width,0.0f)); prior to calling Begin() to specify width. Read code in imgui_demo in the "Horizontal Scrolling" section.
|
||||
ImGuiWindowFlags_NoFocusOnAppearing = 1 << 12, // Disable taking focus when transitioning from hidden to visible state
|
||||
|
@ -534,7 +526,8 @@ enum ImGuiWindowFlags_
|
|||
ImGuiWindowFlags_AlwaysUseWindowPadding = 1 << 16, // Ensure child windows without border uses style.WindowPadding (ignored by default for non-bordered child windows, because more convenient)
|
||||
ImGuiWindowFlags_NoNavFocus = 1 << 17, // No focusing of this window with gamepad/keyboard navigation
|
||||
ImGuiWindowFlags_NoNavInputs = 1 << 18, // No gamepad/keyboard navigation within the window
|
||||
//ImGuiWindowFlags_NavFlattened = 1 << 19, // Allow gamepad/keyboard navigation to cross over parent border to this child (only use on child that have no scrolling!)
|
||||
ImGuiWindowFlags_NavFlattened = 1 << 19, // Allow gamepad/keyboard navigation to cross over parent border to this child (only use on child that have no scrolling!)
|
||||
ImGuiWindowFlags_NoNavScroll = 1 << 20, // No scrolling of this window with gamepad/keyboard navigation
|
||||
// [Internal]
|
||||
ImGuiWindowFlags_ChildWindow = 1 << 22, // Don't use! For internal use by BeginChild()
|
||||
ImGuiWindowFlags_ComboBox = 1 << 23, // Don't use! For internal use by ComboBox()
|
||||
|
@ -581,8 +574,9 @@ enum ImGuiTreeNodeFlags_
|
|||
ImGuiTreeNodeFlags_OpenOnArrow = 1 << 7, // Only open when clicking on the arrow part. If ImGuiTreeNodeFlags_OpenOnDoubleClick is also set, single-click arrow or double-click all box to open.
|
||||
ImGuiTreeNodeFlags_Leaf = 1 << 8, // No collapsing, no arrow (use as a convenience for leaf nodes).
|
||||
ImGuiTreeNodeFlags_Bullet = 1 << 9, // Display a bullet instead of arrow
|
||||
//ImGuITreeNodeFlags_SpanAllAvailWidth = 1 << 10, // FIXME: TODO: Extend hit box horizontally even if not framed
|
||||
//ImGuiTreeNodeFlags_NoScrollOnOpen = 1 << 11, // FIXME: TODO: Disable automatic scroll on TreePop() if node got just open and contents is not visible
|
||||
ImGuiTreeNodeFlags_FramePadding = 1 << 10, // Use FramePadding (even for an unframed text node) to vertically align text baseline to regular widget height. Equivalent to calling AlignTextToFramePadding().
|
||||
//ImGuITreeNodeFlags_SpanAllAvailWidth = 1 << 11, // FIXME: TODO: Extend hit box horizontally even if not framed
|
||||
//ImGuiTreeNodeFlags_NoScrollOnOpen = 1 << 12, // FIXME: TODO: Disable automatic scroll on TreePop() if node got just open and contents is not visible
|
||||
ImGuiTreeNodeFlags_CollapsingHeader = ImGuiTreeNodeFlags_Framed | ImGuiTreeNodeFlags_NoAutoOpenOnLog
|
||||
};
|
||||
|
||||
|
@ -595,6 +589,17 @@ enum ImGuiSelectableFlags_
|
|||
ImGuiSelectableFlags_AllowDoubleClick = 1 << 2 // Generate press events on double clicks too
|
||||
};
|
||||
|
||||
// Flags for ImGui::IsItemHovered(), ImGui::IsWindowHovered()
|
||||
enum ImGuiHoveredFlags_
|
||||
{
|
||||
ImGuiHoveredFlags_Default = 0, // Return true if directly over the item/window, not obstructed by another window, not obstructed by an active popup or modal blocking inputs under them.
|
||||
ImGuiHoveredFlags_AllowWhenBlockedByPopup = 1 << 0, // Return true even if a popup window is normally blocking access to this item/window
|
||||
//ImGuiHoveredFlags_AllowWhenBlockedByModal = 1 << 1, // Return true even if a modal popup window is normally blocking access to this item/window. FIXME-TODO: Unavailable yet.
|
||||
ImGuiHoveredFlags_AllowWhenBlockedByActiveItem = 1 << 2, // Return true even if an active item is blocking access to this item/window
|
||||
ImGuiHoveredFlags_AllowWhenOverlapped = 1 << 3, // Return true even if the position is overlapped by another window
|
||||
ImGuiHoveredFlags_RectOnly = ImGuiHoveredFlags_AllowWhenBlockedByPopup | ImGuiHoveredFlags_AllowWhenBlockedByActiveItem | ImGuiHoveredFlags_AllowWhenOverlapped
|
||||
};
|
||||
|
||||
// User fill ImGuiIO.KeyMap[] array with indices into the ImGuiIO.KeysDown[512] array
|
||||
enum ImGuiKey_
|
||||
{
|
||||
|
@ -643,6 +648,7 @@ enum ImGuiNavInput_
|
|||
ImGuiNavInput_PadFocusNext, // prev window (with PadMenu held) // e.g. R-trigger
|
||||
ImGuiNavInput_PadTweakSlow, // slower tweaks // e.g. L-trigger, analog
|
||||
ImGuiNavInput_PadTweakFast, // faster tweaks // e.g. R-trigger, analog
|
||||
ImGuiNavInput_KeyMenu, // access menu // e.g. ALT
|
||||
ImGuiNavInput_COUNT,
|
||||
};
|
||||
|
||||
|
@ -896,41 +902,63 @@ struct ImGuiIO
|
|||
// Output - Retrieve after calling NewFrame()
|
||||
//------------------------------------------------------------------
|
||||
|
||||
bool WantCaptureMouse; // Mouse is hovering a window or widget is active (= ImGui will use your mouse input). Use to hide mouse from the rest of your application
|
||||
bool WantCaptureKeyboard; // Widget is active (= ImGui will use your keyboard input). Use to hide keyboard from the rest of your application
|
||||
bool WantTextInput; // Some text input widget is active, which will read input characters from the InputCharacters array. Use to activate on screen keyboard if your system needs one
|
||||
bool WantMoveMouse; // MousePos has been altered. back-end should reposition mouse on next frame. used only if 'NavMovesMouse=true'.
|
||||
bool NavUsable; // Directional navigation is currently allowed (ImGuiKey_NavXXX events).
|
||||
bool NavActive; // Directional navigation is active/visible and currently allowed (ImGuiKey_NavXXX events).
|
||||
bool WantCaptureMouse; // When io.WantCaptureMouse is true, do not dispatch mouse input data to your main application. This is set by ImGui when it wants to use your mouse (e.g. unclicked mouse is hovering a window, or a widget is active).
|
||||
bool WantCaptureKeyboard; // When io.WantCaptureKeyboard is true, do not dispatch keyboard input data to your main application. This is set by ImGui when it wants to use your keyboard inputs.
|
||||
bool WantTextInput; // Mobile/console: when io.WantTextInput is true, you may display an on-screen keyboard. This is set by ImGui when it wants textual keyboard input to happen (e.g. when a InputText widget is active).
|
||||
bool WantMoveMouse; // MousePos has been altered, back-end should reposition mouse on next frame. Set only when 'NavMovesMouse=true'.
|
||||
bool NavUsable; // Directional navigation is currently allowed (will handle ImGuiKey_NavXXX events).
|
||||
bool NavActive; // Directional navigation is active/visible and currently allowed (will handle ImGuiKey_NavXXX events).
|
||||
float Framerate; // Application framerate estimation, in frame per second. Solely for convenience. Rolling average estimation based on IO.DeltaTime over 120 frames
|
||||
int MetricsAllocs; // Number of active memory allocations
|
||||
int MetricsRenderVertices; // Vertices output during last call to Render()
|
||||
int MetricsRenderIndices; // Indices output during last call to Render() = number of triangles * 3
|
||||
int MetricsActiveWindows; // Number of visible root windows (exclude child windows)
|
||||
ImVec2 MouseDelta; // Mouse delta. Note that this is zero if either current or previous position are negative, so a disappearing/reappearing mouse won't have a huge delta for one frame.
|
||||
ImVec2 MouseDelta; // Mouse delta. Note that this is zero if either current or previous position are invalid (-FLT_MAX,-FLT_MAX), so a disappearing/reappearing mouse won't have a huge delta.
|
||||
|
||||
//------------------------------------------------------------------
|
||||
// [Internal] ImGui will maintain those fields. Forward compatibility not guaranteed!
|
||||
//------------------------------------------------------------------
|
||||
|
||||
ImVec2 MousePosPrev; // Previous mouse position temporary storage (nb: not for public use, set to MousePos in NewFrame())
|
||||
bool MouseClicked[5]; // Mouse button went from !Down to Down
|
||||
ImVec2 MouseClickedPos[5]; // Position at time of clicking
|
||||
float MouseClickedTime[5]; // Time of last click (used to figure out double-click)
|
||||
bool MouseClicked[5]; // Mouse button went from !Down to Down
|
||||
bool MouseDoubleClicked[5]; // Has mouse button been double-clicked?
|
||||
bool MouseReleased[5]; // Mouse button went from Down to !Down
|
||||
bool MouseDownOwned[5]; // Track if button was clicked inside a window. We don't request mouse capture from the application if click started outside ImGui bounds.
|
||||
float MouseDownDuration[5]; // Duration the mouse button has been down (0.0f == just clicked)
|
||||
float MouseDownDurationPrev[5]; // Previous time the mouse button has been down
|
||||
float MouseDragMaxDistanceSqr[5]; // Squared maximum distance of how much mouse has traveled from the click point
|
||||
ImVec2 MouseDragMaxDistanceAbs[5]; // Maximum distance, absolute, on each axis, of how much mouse has traveled from the clicking point
|
||||
float MouseDragMaxDistanceSqr[5]; // Squared maximum distance of how much mouse has traveled from the clicking point
|
||||
float KeysDownDuration[512]; // Duration the keyboard key has been down (0.0f == just pressed)
|
||||
float KeysDownDurationPrev[512]; // Previous duration the key has been down
|
||||
float NavInputsDownDuration[ImGuiNavInput_COUNT];
|
||||
float NavInputsPrev[ImGuiNavInput_COUNT];
|
||||
float NavInputsDownDurationPrev[ImGuiNavInput_COUNT];
|
||||
|
||||
IMGUI_API ImGuiIO();
|
||||
};
|
||||
|
||||
//-----------------------------------------------------------------------------
|
||||
// Obsolete functions (Will be removed! Also see 'API BREAKING CHANGES' section in imgui.cpp)
|
||||
//-----------------------------------------------------------------------------
|
||||
|
||||
#ifndef IMGUI_DISABLE_OBSOLETE_FUNCTIONS
|
||||
namespace ImGui
|
||||
{
|
||||
bool Begin(const char* name, bool* p_open, const ImVec2& size_on_first_use, float bg_alpha_override = -1.0f, ImGuiWindowFlags flags = 0); // OBSOLETE 1.52+. use SetNextWindowSize() instead if you want to set a window size.
|
||||
static inline void AlignFirstTextHeightToWidgets() { AlignTextToFramePadding(); } // OBSOLETE 1.52+
|
||||
static inline void SetNextWindowPosCenter(ImGuiCond cond = 0) { SetNextWindowPos(ImVec2(GetIO().DisplaySize.x * 0.5f, GetIO().DisplaySize.y * 0.5f), cond, ImVec2(0.5f, 0.5f)); } // OBSOLETE 1.52+
|
||||
static inline bool IsItemHoveredRect() { return IsItemHovered(ImGuiHoveredFlags_RectOnly); } // OBSOLETE 1.51+
|
||||
static inline bool IsPosHoveringAnyWindow(const ImVec2&) { IM_ASSERT(0); return false; } // OBSOLETE 1.51+. This was partly broken. You probably wanted to use ImGui::GetIO().WantCaptureMouse instead.
|
||||
static inline bool IsMouseHoveringAnyWindow() { return IsAnyWindowHovered(); } // OBSOLETE 1.51+
|
||||
static inline bool IsMouseHoveringWindow() { return IsWindowHovered(ImGuiHoveredFlags_AllowWhenBlockedByPopup | ImGuiHoveredFlags_AllowWhenBlockedByActiveItem); } // OBSOLETE 1.51+
|
||||
static inline bool CollapsingHeader(const char* label, const char* str_id, bool framed = true, bool default_open = false) { (void)str_id; (void)framed; ImGuiTreeNodeFlags default_open_flags = 1 << 5; return CollapsingHeader(label, (default_open ? default_open_flags : 0)); } // OBSOLETE 1.49+
|
||||
static inline ImFont* GetWindowFont() { return GetFont(); } // OBSOLETE 1.48+
|
||||
static inline float GetWindowFontSize() { return GetFontSize(); } // OBSOLETE 1.48+
|
||||
static inline void SetScrollPosHere() { SetScrollHere(); } // OBSOLETE 1.42+
|
||||
}
|
||||
#endif
|
||||
|
||||
//-----------------------------------------------------------------------------
|
||||
// Helpers
|
||||
//-----------------------------------------------------------------------------
|
||||
|
@ -1417,9 +1445,9 @@ struct ImFontAtlas
|
|||
IMGUI_API ImFont* AddFont(const ImFontConfig* font_cfg);
|
||||
IMGUI_API ImFont* AddFontDefault(const ImFontConfig* font_cfg = NULL);
|
||||
IMGUI_API ImFont* AddFontFromFileTTF(const char* filename, float size_pixels, const ImFontConfig* font_cfg = NULL, const ImWchar* glyph_ranges = NULL);
|
||||
IMGUI_API ImFont* AddFontFromMemoryTTF(void* font_data, int font_size, float size_pixels, const ImFontConfig* font_cfg = NULL, const ImWchar* glyph_ranges = NULL); // Transfer ownership of 'ttf_data' to ImFontAtlas, will be deleted after Build()
|
||||
IMGUI_API ImFont* AddFontFromMemoryCompressedTTF(const void* compressed_font_data, int compressed_font_size, float size_pixels, const ImFontConfig* font_cfg = NULL, const ImWchar* glyph_ranges = NULL); // 'compressed_font_data' still owned by caller. Compress with binary_to_compressed_c.cpp
|
||||
IMGUI_API ImFont* AddFontFromMemoryCompressedBase85TTF(const char* compressed_font_data_base85, float size_pixels, const ImFontConfig* font_cfg = NULL, const ImWchar* glyph_ranges = NULL); // 'compressed_font_data_base85' still owned by caller. Compress with binary_to_compressed_c.cpp with -base85 paramaeter
|
||||
IMGUI_API ImFont* AddFontFromMemoryTTF(void* font_data, int font_size, float size_pixels, const ImFontConfig* font_cfg = NULL, const ImWchar* glyph_ranges = NULL); // Note: Transfer ownership of 'ttf_data' to ImFontAtlas! Will be deleted after Build(). Set font_cfg->FontDataOwnedByAtlas to false to keep ownership.
|
||||
IMGUI_API ImFont* AddFontFromMemoryCompressedTTF(const void* compressed_font_data, int compressed_font_size, float size_pixels, const ImFontConfig* font_cfg = NULL, const ImWchar* glyph_ranges = NULL); // 'compressed_font_data' still owned by caller. Compress with binary_to_compressed_c.cpp.
|
||||
IMGUI_API ImFont* AddFontFromMemoryCompressedBase85TTF(const char* compressed_font_data_base85, float size_pixels, const ImFontConfig* font_cfg = NULL, const ImWchar* glyph_ranges = NULL); // 'compressed_font_data_base85' still owned by caller. Compress with binary_to_compressed_c.cpp with -base85 parameter.
|
||||
IMGUI_API void ClearTexData(); // Clear the CPU-side texture data. Saves RAM once the texture has been copied to graphics memory.
|
||||
IMGUI_API void ClearInputData(); // Clear the input TTF data (inc sizes, glyph ranges)
|
||||
IMGUI_API void ClearFonts(); // Clear the ImGui-side font data (glyphs storage, UV coordinates)
|
||||
|
@ -1529,7 +1557,7 @@ struct ImFont
|
|||
// Methods
|
||||
IMGUI_API ImFont();
|
||||
IMGUI_API ~ImFont();
|
||||
IMGUI_API void Clear();
|
||||
IMGUI_API void ClearOutputData();
|
||||
IMGUI_API void BuildLookupTable();
|
||||
IMGUI_API const ImFontGlyph*FindGlyph(ImWchar c) const;
|
||||
IMGUI_API void SetFallbackChar(ImWchar c);
|
||||
|
|
|
@ -1,4 +1,4 @@
|
|||
// dear imgui, v1.52 WIP
|
||||
// dear imgui, v1.53 WIP
|
||||
// (demo code)
|
||||
|
||||
// Message to the person tempted to delete this file when integrating ImGui into their code base:
|
||||
|
@ -101,23 +101,23 @@ static void ShowHelpMarker(const char* desc)
|
|||
void ImGui::ShowUserGuide()
|
||||
{
|
||||
ImGui::BulletText("Double-click on title bar to collapse window.");
|
||||
ImGui::BulletText("Click and drag on lower right corner to resize window.");
|
||||
ImGui::BulletText("Click and drag on lower right corner to resize window\n(double-click to auto fit window to its contents).");
|
||||
ImGui::BulletText("Click and drag on any empty space to move window.");
|
||||
ImGui::BulletText("Mouse Wheel to scroll.");
|
||||
ImGui::BulletText("TAB/SHIFT+TAB to cycle through keyboard editable fields.");
|
||||
ImGui::BulletText("CTRL+Click on a slider or drag box to input value as text.");
|
||||
if (ImGui::GetIO().FontAllowUserScaling)
|
||||
ImGui::BulletText("CTRL+Mouse Wheel to zoom window contents.");
|
||||
ImGui::BulletText("TAB/SHIFT+TAB to cycle through keyboard editable fields.");
|
||||
ImGui::BulletText("CTRL+Click on a slider or drag box to input text.");
|
||||
ImGui::BulletText(
|
||||
"While editing text:\n"
|
||||
"- Hold SHIFT or use mouse to select text\n"
|
||||
"- CTRL+Left/Right to word jump\n"
|
||||
"- CTRL+A or double-click to select all\n"
|
||||
"- CTRL+X,CTRL+C,CTRL+V clipboard\n"
|
||||
"- CTRL+Z,CTRL+Y undo/redo\n"
|
||||
"- ESCAPE to revert\n"
|
||||
"- You can apply arithmetic operators +,*,/ on numerical values.\n"
|
||||
" Use +- to subtract.\n");
|
||||
ImGui::BulletText("Mouse Wheel to scroll.");
|
||||
ImGui::BulletText("While editing text:\n");
|
||||
ImGui::Indent();
|
||||
ImGui::BulletText("Hold SHIFT or use mouse to select text.");
|
||||
ImGui::BulletText("CTRL+Left/Right to word jump.");
|
||||
ImGui::BulletText("CTRL+A or double-click to select all.");
|
||||
ImGui::BulletText("CTRL+X,CTRL+C,CTRL+V to use clipboard.");
|
||||
ImGui::BulletText("CTRL+Z,CTRL+Y to undo/redo.");
|
||||
ImGui::BulletText("ESCAPE to revert.");
|
||||
ImGui::BulletText("You can apply arithmetic operators +,*,/ on numerical values.\nUse +- to subtract.");
|
||||
ImGui::Unindent();
|
||||
}
|
||||
|
||||
// Demonstrate most ImGui features (big function!)
|
||||
|
@ -232,7 +232,8 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
ImGui::Spacing();
|
||||
if (ImGui::CollapsingHeader("Help"))
|
||||
{
|
||||
ImGui::TextWrapped("This window is being created by the ShowTestWindow() function. Please refer to the code for programming reference.\n\nUser Guide:");
|
||||
ImGui::TextWrapped("This window is being created by the ShowTestWindow() function. Please refer to the code in imgui_demo.cpp for reference.\n\n");
|
||||
ImGui::Text("USER GUIDE:");
|
||||
ImGui::ShowUserGuide();
|
||||
}
|
||||
|
||||
|
@ -432,7 +433,8 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
else
|
||||
{
|
||||
// Leaf: The only reason we have a TreeNode at all is to allow selection of the leaf. Otherwise we can use BulletText() or TreeAdvanceToLabelPos()+Text().
|
||||
ImGui::TreeNodeEx((void*)(intptr_t)i, node_flags | ImGuiTreeNodeFlags_Leaf | ImGuiTreeNodeFlags_NoTreePushOnOpen, "Selectable Leaf %d", i);
|
||||
node_flags |= ImGuiTreeNodeFlags_Leaf | ImGuiTreeNodeFlags_NoTreePushOnOpen; // ImGuiTreeNodeFlags_Bullet
|
||||
ImGui::TreeNodeEx((void*)(intptr_t)i, node_flags, "Selectable Leaf %d", i);
|
||||
if (ImGui::IsItemClicked())
|
||||
node_clicked = i;
|
||||
}
|
||||
|
@ -459,11 +461,13 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
ImGui::Checkbox("Enable extra group", &closable_group);
|
||||
if (ImGui::CollapsingHeader("Header"))
|
||||
{
|
||||
ImGui::Text("IsItemHovered: %d", IsItemHovered());
|
||||
for (int i = 0; i < 5; i++)
|
||||
ImGui::Text("Some content %d", i);
|
||||
}
|
||||
if (ImGui::CollapsingHeader("Header with a close button", &closable_group))
|
||||
{
|
||||
ImGui::Text("IsItemHovered: %d", IsItemHovered());
|
||||
for (int i = 0; i < 5; i++)
|
||||
ImGui::Text("More content %d", i);
|
||||
}
|
||||
|
@ -542,17 +546,27 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
{
|
||||
ImGui::TextWrapped("Below we are displaying the font texture (which is the only texture we have access to in this demo). Use the 'ImTextureID' type as storage to pass pointers or identifier to your own texture data. Hover the texture for a zoomed view!");
|
||||
ImVec2 tex_screen_pos = ImGui::GetCursorScreenPos();
|
||||
float tex_w = (float)ImGui::GetIO().Fonts->TexWidth;
|
||||
float tex_h = (float)ImGui::GetIO().Fonts->TexHeight;
|
||||
ImTextureID tex_id = ImGui::GetIO().Fonts->TexID;
|
||||
ImGuiIO& io = ImGui::GetIO();
|
||||
|
||||
// Here we are grabbing the font texture because that's the only one we have access to inside the demo code.
|
||||
// Remember that ImTextureID is just storage for whatever you want it to be, it is essentially a value that will be passed to the render function inside the ImDrawCmd structure.
|
||||
// If you use one of the default imgui_impl_XXXX.cpp renderer, they all have comments at the top of their file to specify what they expect to be stored in ImTextureID.
|
||||
// (for example, the imgui_impl_dx11.cpp renderer expect a 'ID3D11ShaderResourceView*' pointer. The imgui_impl_glfw_gl3.cpp renderer expect a GLuint OpenGL texture identifier etc.)
|
||||
// If you decided that ImTextureID = MyEngineTexture*, then you can pass your MyEngineTexture* pointers to ImGui::Image(), and gather width/height through your own functions, etc.
|
||||
// Using ShowMetricsWindow() as a "debugger" to inspect the draw data that are being passed to your render will help you debug issues if you are confused about this.
|
||||
// Consider using the lower-level ImDrawList::AddImage() API, via ImGui::GetWindowDrawList()->AddImage().
|
||||
ImTextureID tex_id = io.Fonts->TexID;
|
||||
float tex_w = (float)io.Fonts->TexWidth;
|
||||
float tex_h = (float)io.Fonts->TexHeight;
|
||||
|
||||
ImGui::Text("%.0fx%.0f", tex_w, tex_h);
|
||||
ImGui::Image(tex_id, ImVec2(tex_w, tex_h), ImVec2(0,0), ImVec2(1,1), ImColor(255,255,255,255), ImColor(255,255,255,128));
|
||||
if (ImGui::IsItemHovered())
|
||||
{
|
||||
ImGui::BeginTooltip();
|
||||
float focus_sz = 32.0f;
|
||||
float focus_x = ImGui::GetMousePos().x - tex_screen_pos.x - focus_sz * 0.5f; if (focus_x < 0.0f) focus_x = 0.0f; else if (focus_x > tex_w - focus_sz) focus_x = tex_w - focus_sz;
|
||||
float focus_y = ImGui::GetMousePos().y - tex_screen_pos.y - focus_sz * 0.5f; if (focus_y < 0.0f) focus_y = 0.0f; else if (focus_y > tex_h - focus_sz) focus_y = tex_h - focus_sz;
|
||||
float focus_x = io.MousePos.x - tex_screen_pos.x - focus_sz * 0.5f; if (focus_x < 0.0f) focus_x = 0.0f; else if (focus_x > tex_w - focus_sz) focus_x = tex_w - focus_sz;
|
||||
float focus_y = io.MousePos.y - tex_screen_pos.y - focus_sz * 0.5f; if (focus_y < 0.0f) focus_y = 0.0f; else if (focus_y > tex_h - focus_sz) focus_y = tex_h - focus_sz;
|
||||
ImGui::Text("Min: (%.2f, %.2f)", focus_x, focus_y);
|
||||
ImGui::Text("Max: (%.2f, %.2f)", focus_x + focus_sz, focus_y + focus_sz);
|
||||
ImVec2 uv0 = ImVec2((focus_x) / tex_w, (focus_y) / tex_h);
|
||||
|
@ -1056,7 +1070,7 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
ImGui::TextColored(ImVec4(1,1,0,1), "Sailor");
|
||||
|
||||
// Button
|
||||
ImGui::AlignFirstTextHeightToWidgets();
|
||||
ImGui::AlignTextToFramePadding();
|
||||
ImGui::Text("Normal buttons"); ImGui::SameLine();
|
||||
ImGui::Button("Banana"); ImGui::SameLine();
|
||||
ImGui::Button("Apple"); ImGui::SameLine();
|
||||
|
@ -1182,7 +1196,7 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
ImGui::Text("TEST"); ImGui::SameLine();
|
||||
ImGui::SmallButton("TEST##2");
|
||||
|
||||
ImGui::AlignFirstTextHeightToWidgets(); // If your line starts with text, call this to align it to upcoming widgets.
|
||||
ImGui::AlignTextToFramePadding(); // If your line starts with text, call this to align it to upcoming widgets.
|
||||
ImGui::Text("Text aligned to Widget"); ImGui::SameLine();
|
||||
ImGui::Button("Widget##1"); ImGui::SameLine();
|
||||
ImGui::Text("Widget"); ImGui::SameLine();
|
||||
|
@ -1195,7 +1209,7 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
ImGui::SameLine(0.0f, spacing);
|
||||
if (ImGui::TreeNode("Node##1")) { for (int i = 0; i < 6; i++) ImGui::BulletText("Item %d..", i); ImGui::TreePop(); } // Dummy tree data
|
||||
|
||||
ImGui::AlignFirstTextHeightToWidgets(); // Vertically align text node a bit lower so it'll be vertically centered with upcoming widget. Otherwise you can use SmallButton (smaller fit).
|
||||
ImGui::AlignTextToFramePadding(); // Vertically align text node a bit lower so it'll be vertically centered with upcoming widget. Otherwise you can use SmallButton (smaller fit).
|
||||
bool node_open = ImGui::TreeNode("Node##2"); // Common mistake to avoid: if we want to SameLine after TreeNode we need to do it before we add child content.
|
||||
ImGui::SameLine(0.0f, spacing); ImGui::Button("Button##2");
|
||||
if (node_open) { for (int i = 0; i < 6; i++) ImGui::BulletText("Item %d..", i); ImGui::TreePop(); } // Dummy tree data
|
||||
|
@ -1205,7 +1219,7 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
ImGui::SameLine(0.0f, spacing);
|
||||
ImGui::BulletText("Bullet text");
|
||||
|
||||
ImGui::AlignFirstTextHeightToWidgets();
|
||||
ImGui::AlignTextToFramePadding();
|
||||
ImGui::BulletText("Node");
|
||||
ImGui::SameLine(0.0f, spacing); ImGui::Button("Button##4");
|
||||
|
||||
|
@ -1383,41 +1397,32 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
ImGui::EndPopup();
|
||||
}
|
||||
|
||||
ImGui::Spacing();
|
||||
ImGui::TextWrapped("Below we are testing adding menu items to a regular window. It's rather unusual but should work!");
|
||||
ImGui::Separator();
|
||||
// NB: As a quirk in this very specific example, we want to differentiate the parent of this menu from the parent of the various popup menus above.
|
||||
// To do so we are encloding the items in a PushID()/PopID() block to make them two different menusets. If we don't, opening any popup above and hovering our menu here
|
||||
// would open it. This is because once a menu is active, we allow to switch to a sibling menu by just hovering on it, which is the desired behavior for regular menus.
|
||||
ImGui::PushID("foo");
|
||||
ImGui::MenuItem("Menu item", "CTRL+M");
|
||||
if (ImGui::BeginMenu("Menu inside a regular window"))
|
||||
{
|
||||
ShowExampleMenuFile();
|
||||
ImGui::EndMenu();
|
||||
}
|
||||
ImGui::PopID();
|
||||
ImGui::Separator();
|
||||
|
||||
ImGui::TreePop();
|
||||
}
|
||||
|
||||
if (ImGui::TreeNode("Context menus"))
|
||||
{
|
||||
// BeginPopupContextItem() is a helper to provide common/simple popup behavior of essentially doing:
|
||||
// if (IsItemHovered() && IsMouseClicked(0))
|
||||
// OpenPopup(id);
|
||||
// return BeginPopup(id);
|
||||
// For more advanced uses you may want to replicate and cuztomize this code. This the comments inside BeginPopupContextItem() implementation.
|
||||
static float value = 0.5f;
|
||||
ImGui::Text("Value = %.3f (<-- right-click here)", value);
|
||||
if (ImGui::BeginPopupContextItem("item context menu"))
|
||||
{
|
||||
if (ImGui::Selectable("Set to zero")) value = 0.0f;
|
||||
if (ImGui::Selectable("Set to PI")) value = 3.1415f;
|
||||
ImGui::DragFloat("Value", &value, 0.1f, 0.0f, 0.0f);
|
||||
ImGui::PushItemWidth(-1);
|
||||
ImGui::DragFloat("##Value", &value, 0.1f, 0.0f, 0.0f);
|
||||
ImGui::PopItemWidth();
|
||||
ImGui::EndPopup();
|
||||
}
|
||||
|
||||
static char name[32] = "Label1";
|
||||
char buf[64]; sprintf(buf, "Button: %s###Button", name); // ### operator override ID ignoring the preceeding label
|
||||
char buf[64]; sprintf(buf, "Button: %s###Button", name); // ### operator override ID ignoring the preceding label
|
||||
ImGui::Button(buf);
|
||||
if (ImGui::BeginPopupContextItem("rename context menu"))
|
||||
if (ImGui::BeginPopupContextItem()) // When used after an item that has an ID (here the Button), we can skip providing an ID to BeginPopupContextItem().
|
||||
{
|
||||
ImGui::Text("Edit name:");
|
||||
ImGui::InputText("##edit", name, IM_ARRAYSIZE(name));
|
||||
|
@ -1463,6 +1468,8 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
ImGui::Text("Hello from Stacked The First\nUsing style.Colors[ImGuiCol_ModalWindowDarkening] for darkening.");
|
||||
static int item = 1;
|
||||
ImGui::Combo("Combo", &item, "aaaa\0bbbb\0cccc\0dddd\0eeee\0\0");
|
||||
static float color[4] = { 0.4f,0.7f,0.0f,0.5f };
|
||||
ImGui::ColorEdit4("color", color); // This is to test behavior of stacked regular popups over a modal
|
||||
|
||||
if (ImGui::Button("Add another modal.."))
|
||||
ImGui::OpenPopup("Stacked 2");
|
||||
|
@ -1481,6 +1488,25 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
|
||||
ImGui::TreePop();
|
||||
}
|
||||
|
||||
if (ImGui::TreeNode("Menus inside a regular window"))
|
||||
{
|
||||
ImGui::TextWrapped("Below we are testing adding menu items to a regular window. It's rather unusual but should work!");
|
||||
ImGui::Separator();
|
||||
// NB: As a quirk in this very specific example, we want to differentiate the parent of this menu from the parent of the various popup menus above.
|
||||
// To do so we are encloding the items in a PushID()/PopID() block to make them two different menusets. If we don't, opening any popup above and hovering our menu here
|
||||
// would open it. This is because once a menu is active, we allow to switch to a sibling menu by just hovering on it, which is the desired behavior for regular menus.
|
||||
ImGui::PushID("foo");
|
||||
ImGui::MenuItem("Menu item", "CTRL+M");
|
||||
if (ImGui::BeginMenu("Menu inside a regular window"))
|
||||
{
|
||||
ShowExampleMenuFile();
|
||||
ImGui::EndMenu();
|
||||
}
|
||||
ImGui::PopID();
|
||||
ImGui::Separator();
|
||||
ImGui::TreePop();
|
||||
}
|
||||
}
|
||||
|
||||
if (ImGui::CollapsingHeader("Columns"))
|
||||
|
@ -1788,6 +1814,34 @@ void ImGui::ShowTestWindow(bool* p_open)
|
|||
}
|
||||
#endif
|
||||
|
||||
if (ImGui::TreeNode("Hovering"))
|
||||
{
|
||||
// Testing IsWindowHovered() function
|
||||
ImGui::BulletText(
|
||||
"IsWindowHovered() = %d\n"
|
||||
"IsWindowHovered(_AllowWhenBlockedByPopup) = %d\n"
|
||||
"IsWindowHovered(_AllowWhenBlockedByActiveItem) = %d\n",
|
||||
ImGui::IsWindowHovered(),
|
||||
ImGui::IsWindowHovered(ImGuiHoveredFlags_AllowWhenBlockedByPopup),
|
||||
ImGui::IsWindowHovered(ImGuiHoveredFlags_AllowWhenBlockedByActiveItem));
|
||||
|
||||
// Testing IsItemHovered() function (because BulletText is an item itself and that would affect the output of IsItemHovered, we pass all lines in a single items to shorten the code)
|
||||
ImGui::Button("ITEM");
|
||||
ImGui::BulletText(
|
||||
"IsItemHovered() = %d\n"
|
||||
"IsItemHovered(_AllowWhenBlockedByPopup) = %d\n"
|
||||
"IsItemHovered(_AllowWhenBlockedByActiveItem) = %d\n"
|
||||
"IsItemHovered(_AllowWhenOverlapped) = %d\n"
|
||||
"IsItemhovered(_RectOnly) = %d\n",
|
||||
ImGui::IsItemHovered(),
|
||||
ImGui::IsItemHovered(ImGuiHoveredFlags_AllowWhenBlockedByPopup),
|
||||
ImGui::IsItemHovered(ImGuiHoveredFlags_AllowWhenBlockedByActiveItem),
|
||||
ImGui::IsItemHovered(ImGuiHoveredFlags_AllowWhenOverlapped),
|
||||
ImGui::IsItemHovered(ImGuiHoveredFlags_RectOnly));
|
||||
|
||||
ImGui::TreePop();
|
||||
}
|
||||
|
||||
if (ImGui::TreeNode("Dragging"))
|
||||
{
|
||||
ImGui::TextWrapped("You can use ImGui::GetMouseDragDelta(0) to query for the dragged amount on any widget.");
|
||||
|
@ -1946,6 +2000,7 @@ void ImGui::ShowStyleEditor(ImGuiStyle* ref)
|
|||
for (int i = 0; i < atlas->Fonts.Size; i++)
|
||||
{
|
||||
ImFont* font = atlas->Fonts[i];
|
||||
ImGui::PushID(font);
|
||||
bool font_details_opened = ImGui::TreeNode(font, "Font %d: \'%s\', %.2f px, %d glyphs", i, font->ConfigData ? font->ConfigData[0].Name : "", font->FontSize, font->Glyphs.Size);
|
||||
ImGui::SameLine(); if (ImGui::SmallButton("Set as default")) ImGui::GetIO().FontDefault = font;
|
||||
if (font_details_opened)
|
||||
|
@ -2006,6 +2061,7 @@ void ImGui::ShowStyleEditor(ImGuiStyle* ref)
|
|||
}
|
||||
ImGui::TreePop();
|
||||
}
|
||||
ImGui::PopID();
|
||||
}
|
||||
static float window_scale = 1.0f;
|
||||
ImGui::DragFloat("this window scale", &window_scale, 0.005f, 0.3f, 2.0f, "%.1f"); // scale only this window
|
||||
|
@ -2162,7 +2218,7 @@ static void ShowExampleAppFixedOverlay(bool* p_open)
|
|||
ImVec2 window_pos = ImVec2((corner & 1) ? ImGui::GetIO().DisplaySize.x - DISTANCE : DISTANCE, (corner & 2) ? ImGui::GetIO().DisplaySize.y - DISTANCE : DISTANCE);
|
||||
ImVec2 window_pos_pivot = ImVec2((corner & 1) ? 1.0f : 0.0f, (corner & 2) ? 1.0f : 0.0f);
|
||||
ImGui::SetNextWindowPos(window_pos, ImGuiCond_Always, window_pos_pivot);
|
||||
ImGui::PushStyleColor(ImGuiCol_WindowBg, ImVec4(0.0f, 0.0f, 0.0f, 0.3f));
|
||||
ImGui::PushStyleColor(ImGuiCol_WindowBg, ImVec4(0.0f, 0.0f, 0.0f, 0.3f)); // Transparent background
|
||||
if (ImGui::Begin("Example: Fixed Overlay", p_open, ImGuiWindowFlags_NoTitleBar|ImGuiWindowFlags_NoResize|ImGuiWindowFlags_AlwaysAutoResize|ImGuiWindowFlags_NoMove|ImGuiWindowFlags_NoSavedSettings|ImGuiWindowFlags_NoFocusOnAppearing|ImGuiWindowFlags_NoNavFocus|ImGuiWindowFlags_NoNavInputs))
|
||||
{
|
||||
ImGui::Text("Simple overlay\nin the corner of the screen.\n(right-click to change position)");
|
||||
|
@ -2352,6 +2408,7 @@ struct ExampleAppConsole
|
|||
|
||||
void AddLog(const char* fmt, ...) IM_FMTARGS(2)
|
||||
{
|
||||
// FIXME-OPT
|
||||
char buf[1024];
|
||||
va_list args;
|
||||
va_start(args, fmt);
|
||||
|
@ -2752,10 +2809,10 @@ static void ShowExampleAppPropertyEditor(bool* p_open)
|
|||
static void ShowDummyObject(const char* prefix, int uid)
|
||||
{
|
||||
ImGui::PushID(uid); // Use object uid as identifier. Most commonly you could also use the object pointer as a base ID.
|
||||
ImGui::AlignFirstTextHeightToWidgets(); // Text and Tree nodes are less high than regular widgets, here we add vertical spacing to make the tree lines equal high.
|
||||
ImGui::AlignTextToFramePadding(); // Text and Tree nodes are less high than regular widgets, here we add vertical spacing to make the tree lines equal high.
|
||||
bool node_open = ImGui::TreeNode("Object", "%s_%u", prefix, uid);
|
||||
ImGui::NextColumn();
|
||||
ImGui::AlignFirstTextHeightToWidgets();
|
||||
ImGui::AlignTextToFramePadding();
|
||||
ImGui::Text("my sailor is rich");
|
||||
ImGui::NextColumn();
|
||||
if (node_open)
|
||||
|
@ -2770,7 +2827,7 @@ static void ShowExampleAppPropertyEditor(bool* p_open)
|
|||
}
|
||||
else
|
||||
{
|
||||
ImGui::AlignFirstTextHeightToWidgets();
|
||||
ImGui::AlignTextToFramePadding();
|
||||
// Here we use a Selectable (instead of Text) to highlight on hover
|
||||
//ImGui::Text("Field_%d", i);
|
||||
char label[32];
|
||||
|
|
|
@ -1,7 +1,8 @@
|
|||
// dear imgui, v1.52 WIP
|
||||
// dear imgui, v1.53 WIP
|
||||
// (drawing and font code)
|
||||
|
||||
// Contains implementation for
|
||||
// - Default styles
|
||||
// - ImDrawList
|
||||
// - ImDrawData
|
||||
// - ImFontAtlas
|
||||
|
@ -116,6 +117,113 @@ namespace IMGUI_STB_NAMESPACE
|
|||
using namespace IMGUI_STB_NAMESPACE;
|
||||
#endif
|
||||
|
||||
//-----------------------------------------------------------------------------
|
||||
// Style functions
|
||||
//-----------------------------------------------------------------------------
|
||||
|
||||
void ImGui::StyleColorsClassic(ImGuiStyle* dst)
|
||||
{
|
||||
ImGuiStyle* style = dst ? dst : &ImGui::GetStyle();
|
||||
ImVec4* colors = style->Colors;
|
||||
|
||||
colors[ImGuiCol_Text] = ImVec4(0.90f, 0.90f, 0.90f, 1.00f);
|
||||
colors[ImGuiCol_TextDisabled] = ImVec4(0.60f, 0.60f, 0.60f, 1.00f);
|
||||
colors[ImGuiCol_WindowBg] = ImVec4(0.00f, 0.00f, 0.00f, 0.70f);
|
||||
colors[ImGuiCol_ChildWindowBg] = ImVec4(0.00f, 0.00f, 0.00f, 0.00f);
|
||||
colors[ImGuiCol_PopupBg] = ImVec4(0.05f, 0.05f, 0.10f, 0.90f);
|
||||
colors[ImGuiCol_Border] = ImVec4(0.70f, 0.70f, 0.70f, 0.40f);
|
||||
colors[ImGuiCol_BorderShadow] = ImVec4(0.00f, 0.00f, 0.00f, 0.00f);
|
||||
colors[ImGuiCol_FrameBg] = ImVec4(0.80f, 0.80f, 0.80f, 0.30f); // Background of checkbox, radio button, plot, slider, text input
|
||||
colors[ImGuiCol_FrameBgHovered] = ImVec4(0.90f, 0.80f, 0.80f, 0.40f);
|
||||
colors[ImGuiCol_FrameBgActive] = ImVec4(0.90f, 0.65f, 0.65f, 0.45f);
|
||||
colors[ImGuiCol_TitleBg] = ImVec4(0.24f, 0.24f, 0.50f, 0.83f);
|
||||
colors[ImGuiCol_TitleBgCollapsed] = ImVec4(0.40f, 0.40f, 0.80f, 0.20f);
|
||||
colors[ImGuiCol_TitleBgActive] = ImVec4(0.33f, 0.33f, 0.65f, 0.87f);
|
||||
colors[ImGuiCol_MenuBarBg] = ImVec4(0.40f, 0.40f, 0.55f, 0.80f);
|
||||
colors[ImGuiCol_ScrollbarBg] = ImVec4(0.20f, 0.25f, 0.30f, 0.60f);
|
||||
colors[ImGuiCol_ScrollbarGrab] = ImVec4(0.40f, 0.40f, 0.80f, 0.30f);
|
||||
colors[ImGuiCol_ScrollbarGrabHovered] = ImVec4(0.40f, 0.40f, 0.80f, 0.40f);
|
||||
colors[ImGuiCol_ScrollbarGrabActive] = ImVec4(0.80f, 0.50f, 0.50f, 0.40f);
|
||||
colors[ImGuiCol_ComboBg] = ImVec4(0.20f, 0.20f, 0.20f, 0.99f);
|
||||
colors[ImGuiCol_CheckMark] = ImVec4(0.90f, 0.90f, 0.90f, 0.50f);
|
||||
colors[ImGuiCol_SliderGrab] = ImVec4(1.00f, 1.00f, 1.00f, 0.30f);
|
||||
colors[ImGuiCol_SliderGrabActive] = ImVec4(0.80f, 0.50f, 0.50f, 1.00f);
|
||||
colors[ImGuiCol_Button] = ImVec4(0.67f, 0.40f, 0.40f, 0.60f);
|
||||
colors[ImGuiCol_ButtonHovered] = ImVec4(0.67f, 0.40f, 0.40f, 1.00f);
|
||||
colors[ImGuiCol_ButtonActive] = ImVec4(0.80f, 0.50f, 0.50f, 1.00f);
|
||||
colors[ImGuiCol_Header] = ImVec4(0.40f, 0.40f, 0.90f, 0.45f);
|
||||
colors[ImGuiCol_HeaderHovered] = ImVec4(0.45f, 0.45f, 0.90f, 0.80f);
|
||||
colors[ImGuiCol_HeaderActive] = ImVec4(0.53f, 0.53f, 0.87f, 0.80f);
|
||||
colors[ImGuiCol_Separator] = ImVec4(0.50f, 0.50f, 0.50f, 1.00f);
|
||||
colors[ImGuiCol_SeparatorHovered] = ImVec4(0.60f, 0.60f, 0.70f, 1.00f);
|
||||
colors[ImGuiCol_SeparatorActive] = ImVec4(0.70f, 0.70f, 0.90f, 1.00f);
|
||||
colors[ImGuiCol_ResizeGrip] = ImVec4(1.00f, 1.00f, 1.00f, 0.30f);
|
||||
colors[ImGuiCol_ResizeGripHovered] = ImVec4(1.00f, 1.00f, 1.00f, 0.60f);
|
||||
colors[ImGuiCol_ResizeGripActive] = ImVec4(1.00f, 1.00f, 1.00f, 0.90f);
|
||||
colors[ImGuiCol_CloseButton] = ImVec4(0.50f, 0.50f, 0.90f, 0.50f);
|
||||
colors[ImGuiCol_CloseButtonHovered] = ImVec4(0.70f, 0.70f, 0.90f, 0.60f);
|
||||
colors[ImGuiCol_CloseButtonActive] = ImVec4(0.70f, 0.70f, 0.70f, 1.00f);
|
||||
colors[ImGuiCol_PlotLines] = ImVec4(1.00f, 1.00f, 1.00f, 1.00f);
|
||||
colors[ImGuiCol_PlotLinesHovered] = ImVec4(0.90f, 0.70f, 0.00f, 1.00f);
|
||||
colors[ImGuiCol_PlotHistogram] = ImVec4(0.90f, 0.70f, 0.00f, 1.00f);
|
||||
colors[ImGuiCol_PlotHistogramHovered] = ImVec4(1.00f, 0.60f, 0.00f, 1.00f);
|
||||
colors[ImGuiCol_TextSelectedBg] = ImVec4(0.00f, 0.00f, 1.00f, 0.35f);
|
||||
colors[ImGuiCol_ModalWindowDarkening] = ImVec4(0.20f, 0.20f, 0.20f, 0.35f);
|
||||
colors[ImGuiCol_NavHighlight] = colors[ImGuiCol_HeaderHovered];
|
||||
colors[ImGuiCol_NavWindowingHighlight] = ImVec4(1.00f, 1.00f, 1.00f, 0.12f);
|
||||
}
|
||||
|
||||
void ImGui::StyleColorsDark(ImGuiStyle* dst)
|
||||
{
|
||||
ImGuiStyle* style = dst ? dst : &ImGui::GetStyle();
|
||||
ImVec4* colors = style->Colors;
|
||||
|
||||
colors[ImGuiCol_Text] = ImVec4(1.00f, 1.00f, 1.00f, 1.00f);
|
||||
colors[ImGuiCol_TextDisabled] = ImVec4(0.40f, 0.40f, 0.40f, 1.00f);
|
||||
colors[ImGuiCol_WindowBg] = ImVec4(0.06f, 0.06f, 0.06f, 0.94f);
|
||||
colors[ImGuiCol_Border] = ImVec4(1.00f, 1.00f, 1.00f, 0.19f);
|
||||
colors[ImGuiCol_ChildWindowBg] = ImVec4(1.00f, 1.00f, 1.00f, 0.00f);
|
||||
colors[ImGuiCol_PopupBg] = ImVec4(0.00f, 0.00f, 0.00f, 0.94f);
|
||||
colors[ImGuiCol_FrameBg] = ImVec4(0.16f, 0.29f, 0.48f, 0.54f);
|
||||
colors[ImGuiCol_FrameBgHovered] = ImVec4(0.26f, 0.59f, 0.98f, 0.40f);
|
||||
colors[ImGuiCol_FrameBgActive] = ImVec4(0.26f, 0.59f, 0.98f, 0.67f);
|
||||
colors[ImGuiCol_TitleBg] = ImVec4(0.04f, 0.04f, 0.04f, 1.00f);
|
||||
colors[ImGuiCol_TitleBgCollapsed] = ImVec4(0.00f, 0.00f, 0.00f, 0.51f);
|
||||
colors[ImGuiCol_TitleBgActive] = ImVec4(0.18f, 0.18f, 0.18f, 1.00f);
|
||||
colors[ImGuiCol_MenuBarBg] = ImVec4(0.14f, 0.14f, 0.14f, 1.00f);
|
||||
colors[ImGuiCol_ScrollbarBg] = ImVec4(0.02f, 0.02f, 0.02f, 0.53f);
|
||||
colors[ImGuiCol_ScrollbarGrab] = ImVec4(0.31f, 0.31f, 0.31f, 1.00f);
|
||||
colors[ImGuiCol_ScrollbarGrabHovered] = ImVec4(0.41f, 0.41f, 0.41f, 1.00f);
|
||||
colors[ImGuiCol_ScrollbarGrabActive] = ImVec4(0.51f, 0.51f, 0.51f, 1.00f);
|
||||
colors[ImGuiCol_ComboBg] = ImVec4(0.14f, 0.14f, 0.14f, 0.99f);
|
||||
colors[ImGuiCol_CheckMark] = ImVec4(0.26f, 0.59f, 0.98f, 1.00f);
|
||||
colors[ImGuiCol_SliderGrab] = ImVec4(0.24f, 0.52f, 0.88f, 1.00f);
|
||||
colors[ImGuiCol_SliderGrabActive] = ImVec4(0.26f, 0.59f, 0.98f, 1.00f);
|
||||
colors[ImGuiCol_Button] = ImVec4(0.26f, 0.59f, 0.98f, 0.40f);
|
||||
colors[ImGuiCol_ButtonHovered] = ImVec4(0.26f, 0.59f, 0.98f, 1.00f);
|
||||
colors[ImGuiCol_ButtonActive] = ImVec4(0.06f, 0.53f, 0.98f, 1.00f);
|
||||
colors[ImGuiCol_Header] = ImVec4(0.26f, 0.59f, 0.98f, 0.31f);
|
||||
colors[ImGuiCol_HeaderHovered] = ImVec4(0.26f, 0.59f, 0.98f, 0.80f);
|
||||
colors[ImGuiCol_HeaderActive] = ImVec4(0.26f, 0.59f, 0.98f, 1.00f);
|
||||
colors[ImGuiCol_Separator] = colors[ImGuiCol_Border];//ImVec4(0.61f, 0.61f, 0.61f, 1.00f);
|
||||
colors[ImGuiCol_SeparatorHovered] = ImVec4(0.26f, 0.59f, 0.98f, 0.78f);
|
||||
colors[ImGuiCol_SeparatorActive] = ImVec4(0.26f, 0.59f, 0.98f, 1.00f);
|
||||
colors[ImGuiCol_ResizeGrip] = ImVec4(0.26f, 0.59f, 0.98f, 0.25f);
|
||||
colors[ImGuiCol_ResizeGripHovered] = ImVec4(0.26f, 0.59f, 0.98f, 0.67f);
|
||||
colors[ImGuiCol_ResizeGripActive] = ImVec4(0.26f, 0.59f, 0.98f, 0.95f);
|
||||
colors[ImGuiCol_CloseButton] = ImVec4(0.41f, 0.41f, 0.41f, 0.50f);
|
||||
colors[ImGuiCol_CloseButtonHovered] = ImVec4(0.98f, 0.39f, 0.36f, 1.00f);
|
||||
colors[ImGuiCol_CloseButtonActive] = ImVec4(0.98f, 0.39f, 0.36f, 1.00f);
|
||||
colors[ImGuiCol_PlotLines] = ImVec4(0.61f, 0.61f, 0.61f, 1.00f);
|
||||
colors[ImGuiCol_PlotLinesHovered] = ImVec4(1.00f, 0.43f, 0.35f, 1.00f);
|
||||
colors[ImGuiCol_PlotHistogram] = ImVec4(0.90f, 0.70f, 0.00f, 1.00f);
|
||||
colors[ImGuiCol_PlotHistogramHovered] = ImVec4(1.00f, 0.60f, 0.00f, 1.00f);
|
||||
colors[ImGuiCol_TextSelectedBg] = ImVec4(0.26f, 0.59f, 0.98f, 0.35f);
|
||||
colors[ImGuiCol_ModalWindowDarkening] = ImVec4(0.80f, 0.80f, 0.80f, 0.35f);
|
||||
colors[ImGuiCol_NavHighlight] = ImVec4(0.26f, 0.59f, 0.98f, 1.00f);
|
||||
colors[ImGuiCol_NavWindowingHighlight] = ImVec4(1.00f, 1.00f, 1.00f, 0.12f);
|
||||
}
|
||||
|
||||
//-----------------------------------------------------------------------------
|
||||
// ImDrawList
|
||||
//-----------------------------------------------------------------------------
|
||||
|
@ -222,7 +330,7 @@ void ImDrawList::UpdateTextureID()
|
|||
|
||||
// Try to merge with previous command if it matches, else use current command
|
||||
ImDrawCmd* prev_cmd = CmdBuffer.Size > 1 ? curr_cmd - 1 : NULL;
|
||||
if (prev_cmd && prev_cmd->TextureId == curr_texture_id && memcmp(&prev_cmd->ClipRect, &GetCurrentClipRect(), sizeof(ImVec4)) == 0 && prev_cmd->UserCallback == NULL)
|
||||
if (curr_cmd->ElemCount == 0 && prev_cmd && prev_cmd->TextureId == curr_texture_id && memcmp(&prev_cmd->ClipRect, &GetCurrentClipRect(), sizeof(ImVec4)) == 0 && prev_cmd->UserCallback == NULL)
|
||||
CmdBuffer.pop_back();
|
||||
else
|
||||
curr_cmd->TextureId = curr_texture_id;
|
||||
|
@ -339,7 +447,7 @@ void ImDrawList::ChannelsMerge()
|
|||
if (int sz = ch.CmdBuffer.Size) { memcpy(cmd_write, ch.CmdBuffer.Data, sz * sizeof(ImDrawCmd)); cmd_write += sz; }
|
||||
if (int sz = ch.IdxBuffer.Size) { memcpy(_IdxWritePtr, ch.IdxBuffer.Data, sz * sizeof(ImDrawIdx)); _IdxWritePtr += sz; }
|
||||
}
|
||||
AddDrawCmd();
|
||||
UpdateClipRect(); // We call this instead of AddDrawCmd(), so that empty channels won't produce an extra draw call.
|
||||
_ChannelsCount = 1;
|
||||
}
|
||||
|
||||
|
@ -1034,6 +1142,43 @@ void ImDrawData::ScaleClipRects(const ImVec2& scale)
|
|||
}
|
||||
}
|
||||
|
||||
//-----------------------------------------------------------------------------
|
||||
// Shade functions
|
||||
//-----------------------------------------------------------------------------
|
||||
|
||||
// Generic linear color gradient, write to RGB fields, leave A untouched.
|
||||
void ImGui::ShadeVertsLinearColorGradientKeepAlpha(ImDrawVert* vert_start, ImDrawVert* vert_end, ImVec2 gradient_p0, ImVec2 gradient_p1, ImU32 col0, ImU32 col1)
|
||||
{
|
||||
ImVec2 gradient_extent = gradient_p1 - gradient_p0;
|
||||
float gradient_inv_length2 = 1.0f / ImLengthSqr(gradient_extent);
|
||||
for (ImDrawVert* vert = vert_start; vert < vert_end; vert++)
|
||||
{
|
||||
float d = ImDot(vert->pos - gradient_p0, gradient_extent);
|
||||
float t = ImClamp(d * gradient_inv_length2, 0.0f, 1.0f);
|
||||
int r = ImLerp((int)(col0 >> IM_COL32_R_SHIFT) & 0xFF, (int)(col1 >> IM_COL32_R_SHIFT) & 0xFF, t);
|
||||
int g = ImLerp((int)(col0 >> IM_COL32_G_SHIFT) & 0xFF, (int)(col1 >> IM_COL32_G_SHIFT) & 0xFF, t);
|
||||
int b = ImLerp((int)(col0 >> IM_COL32_B_SHIFT) & 0xFF, (int)(col1 >> IM_COL32_B_SHIFT) & 0xFF, t);
|
||||
vert->col = (r << IM_COL32_R_SHIFT) | (g << IM_COL32_G_SHIFT) | (b << IM_COL32_B_SHIFT) | (vert->col & IM_COL32_A_MASK);
|
||||
}
|
||||
}
|
||||
|
||||
// Scan and shade backward from the end of given vertices. Assume vertices are text only (= vert_start..vert_end going left to right) so we can break as soon as we are out the gradient bounds.
|
||||
void ImGui::ShadeVertsLinearAlphaGradientForLeftToRightText(ImDrawVert* vert_start, ImDrawVert* vert_end, float gradient_p0_x, float gradient_p1_x)
|
||||
{
|
||||
float gradient_extent_x = gradient_p1_x - gradient_p0_x;
|
||||
float gradient_inv_length2 = 1.0f / (gradient_extent_x * gradient_extent_x);
|
||||
int full_alpha_count = 0;
|
||||
for (ImDrawVert* vert = vert_end - 1; vert >= vert_start; vert--)
|
||||
{
|
||||
float d = (vert->pos.x - gradient_p0_x) * (gradient_extent_x);
|
||||
float alpha_mul = 1.0f - ImClamp(d * gradient_inv_length2, 0.0f, 1.0f);
|
||||
if (alpha_mul >= 1.0f && ++full_alpha_count > 2)
|
||||
return; // Early out
|
||||
int a = (int)(((vert->col >> IM_COL32_A_SHIFT) & 0xFF) * alpha_mul);
|
||||
vert->col = (vert->col & ~IM_COL32_A_MASK) | (a << IM_COL32_A_SHIFT);
|
||||
}
|
||||
}
|
||||
|
||||
//-----------------------------------------------------------------------------
|
||||
// ImFontConfig
|
||||
//-----------------------------------------------------------------------------
|
||||
|
@ -1438,7 +1583,10 @@ bool ImFontAtlasBuildWithStbTruetype(ImFontAtlas* atlas)
|
|||
const int font_offset = stbtt_GetFontOffsetForIndex((unsigned char*)cfg.FontData, cfg.FontNo);
|
||||
IM_ASSERT(font_offset >= 0);
|
||||
if (!stbtt_InitFont(&tmp.FontInfo, (unsigned char*)cfg.FontData, font_offset))
|
||||
{
|
||||
ImGui::MemFree(tmp_array);
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
// Allocate packing character data and flag packed characters buffer as non-packed (x0=y0=x1=y1=0)
|
||||
|
@ -1540,7 +1688,6 @@ bool ImFontAtlasBuildWithStbTruetype(ImFontAtlas* atlas)
|
|||
const float off_x = cfg.GlyphOffset.x;
|
||||
const float off_y = cfg.GlyphOffset.y + (float)(int)(dst_font->Ascent + 0.5f);
|
||||
|
||||
dst_font->FallbackGlyph = NULL; // Always clear fallback so FindGlyph can return NULL. It will be set again in BuildLookupTable()
|
||||
for (int i = 0; i < tmp.RangesCount; i++)
|
||||
{
|
||||
stbtt_pack_range& range = tmp.Ranges[i];
|
||||
|
@ -1582,14 +1729,12 @@ void ImFontAtlasBuildSetupFont(ImFontAtlas* atlas, ImFont* font, ImFontConfig* f
|
|||
{
|
||||
if (!font_config->MergeMode)
|
||||
{
|
||||
font->ContainerAtlas = atlas;
|
||||
font->ConfigData = font_config;
|
||||
font->ConfigDataCount = 0;
|
||||
font->ClearOutputData();
|
||||
font->FontSize = font_config->SizePixels;
|
||||
font->ConfigData = font_config;
|
||||
font->ContainerAtlas = atlas;
|
||||
font->Ascent = ascent;
|
||||
font->Descent = descent;
|
||||
font->Glyphs.resize(0);
|
||||
font->MetricsTotalSurface = 0;
|
||||
}
|
||||
font->ConfigDataCount++;
|
||||
}
|
||||
|
@ -1814,6 +1959,7 @@ const ImWchar* ImFontAtlas::GetGlyphRangesThai()
|
|||
static const ImWchar ranges[] =
|
||||
{
|
||||
0x0020, 0x00FF, // Basic Latin
|
||||
0x2010, 0x205E, // Punctuations
|
||||
0x0E00, 0x0E7F, // Thai
|
||||
0,
|
||||
};
|
||||
|
@ -1866,7 +2012,8 @@ ImFont::ImFont()
|
|||
{
|
||||
Scale = 1.0f;
|
||||
FallbackChar = (ImWchar)'?';
|
||||
Clear();
|
||||
DisplayOffset = ImVec2(0.0f, 1.0f);
|
||||
ClearOutputData();
|
||||
}
|
||||
|
||||
ImFont::~ImFont()
|
||||
|
@ -1879,13 +2026,12 @@ ImFont::~ImFont()
|
|||
if (g.Font == this)
|
||||
g.Font = NULL;
|
||||
*/
|
||||
Clear();
|
||||
ClearOutputData();
|
||||
}
|
||||
|
||||
void ImFont::Clear()
|
||||
void ImFont::ClearOutputData()
|
||||
{
|
||||
FontSize = 0.0f;
|
||||
DisplayOffset = ImVec2(0.0f, 1.0f);
|
||||
Glyphs.clear();
|
||||
IndexAdvanceX.clear();
|
||||
IndexLookup.clear();
|
||||
|
|
|
@ -1,4 +1,4 @@
|
|||
// dear imgui, v1.52 WIP
|
||||
// dear imgui, v1.53 WIP
|
||||
// (internals)
|
||||
|
||||
// You may use this file to debug, understand or extend ImGui features but we don't provide any guarantee of forward compatibility!
|
||||
|
@ -45,13 +45,12 @@ struct ImGuiMouseCursorData;
|
|||
struct ImGuiPopupRef;
|
||||
struct ImGuiWindow;
|
||||
|
||||
typedef int ImGuiLayoutType; // enum ImGuiLayoutType_
|
||||
typedef int ImGuiButtonFlags; // enum ImGuiButtonFlags_
|
||||
typedef int ImGuiTreeNodeFlags; // enum ImGuiTreeNodeFlags_
|
||||
typedef int ImGuiSliderFlags; // enum ImGuiSliderFlags_
|
||||
typedef int ImGuiSeparatorFlags; // enum ImGuiSeparatorFlags_
|
||||
typedef int ImGuiItemFlags; // enum ImGuiItemFlags_
|
||||
typedef int ImGuiNavHighlightFlags;
|
||||
typedef int ImGuiLayoutType; // enum: horizontal or vertical // enum ImGuiLayoutType_
|
||||
typedef int ImGuiButtonFlags; // flags: for ButtonEx(), ButtonBehavior() // enum ImGuiButtonFlags_
|
||||
typedef int ImGuiItemFlags; // flags: for PushItemFlag() // enum ImGuiItemFlags_
|
||||
typedef int ImGuiNavHighlightFlags; // flags: for RenderNavHighlight() // enum ImGuiNavHighlightFlags_
|
||||
typedef int ImGuiSeparatorFlags; // flags: for Separator() - internal // enum ImGuiSeparatorFlags_
|
||||
typedef int ImGuiSliderFlags; // flags: for SliderBehavior() // enum ImGuiSliderFlags_
|
||||
|
||||
//-------------------------------------------------------------------------
|
||||
// STB libraries
|
||||
|
@ -81,9 +80,8 @@ extern IMGUI_API ImGuiContext* GImGui; // Current implicit ImGui context pointe
|
|||
// Helpers
|
||||
//-----------------------------------------------------------------------------
|
||||
|
||||
#define IM_ARRAYSIZE(_ARR) ((int)(sizeof(_ARR)/sizeof(*_ARR)))
|
||||
#define IM_PI 3.14159265358979323846f
|
||||
#define IM_OFFSETOF(_TYPE,_ELM) ((size_t)&(((_TYPE*)0)->_ELM))
|
||||
#define IM_PI 3.14159265358979323846f
|
||||
#define IM_OFFSETOF(_TYPE,_ELM) ((size_t)&(((_TYPE*)0)->_ELM))
|
||||
|
||||
// Helpers: UTF-8 <> wchar
|
||||
IMGUI_API int ImTextStrToUtf8(char* buf, int buf_size, const ImWchar* in_text, const ImWchar* in_text_end); // return output UTF-8 bytes count
|
||||
|
@ -109,6 +107,7 @@ IMGUI_API void ImTriangleBarycentricCoords(const ImVec2& a, const ImVec
|
|||
// Helpers: String
|
||||
IMGUI_API int ImStricmp(const char* str1, const char* str2);
|
||||
IMGUI_API int ImStrnicmp(const char* str1, const char* str2, int count);
|
||||
IMGUI_API void ImStrncpy(char* dst, const char* src, int count);
|
||||
IMGUI_API char* ImStrdup(const char* str);
|
||||
IMGUI_API int ImStrlenW(const ImWchar* str);
|
||||
IMGUI_API const ImWchar*ImStrbolW(const ImWchar* buf_mid_line, const ImWchar* buf_begin); // Find beginning-of-line
|
||||
|
@ -129,7 +128,9 @@ static inline ImVec2& operator+=(ImVec2& lhs, const ImVec2& rhs)
|
|||
static inline ImVec2& operator-=(ImVec2& lhs, const ImVec2& rhs) { lhs.x -= rhs.x; lhs.y -= rhs.y; return lhs; }
|
||||
static inline ImVec2& operator*=(ImVec2& lhs, const float rhs) { lhs.x *= rhs; lhs.y *= rhs; return lhs; }
|
||||
static inline ImVec2& operator/=(ImVec2& lhs, const float rhs) { lhs.x /= rhs; lhs.y /= rhs; return lhs; }
|
||||
static inline ImVec4 operator+(const ImVec4& lhs, const ImVec4& rhs) { return ImVec4(lhs.x+rhs.x, lhs.y+rhs.y, lhs.z+rhs.z, lhs.w+rhs.w); }
|
||||
static inline ImVec4 operator-(const ImVec4& lhs, const ImVec4& rhs) { return ImVec4(lhs.x-rhs.x, lhs.y-rhs.y, lhs.z-rhs.z, lhs.w-rhs.w); }
|
||||
static inline ImVec4 operator*(const ImVec4& lhs, const ImVec4& rhs) { return ImVec4(lhs.x*rhs.x, lhs.y*rhs.y, lhs.z*rhs.z, lhs.w*rhs.w); }
|
||||
#endif
|
||||
|
||||
static inline int ImMin(int lhs, int rhs) { return lhs < rhs ? lhs : rhs; }
|
||||
|
@ -142,11 +143,13 @@ static inline int ImClamp(int v, int mn, int mx)
|
|||
static inline float ImClamp(float v, float mn, float mx) { return (v < mn) ? mn : (v > mx) ? mx : v; }
|
||||
static inline ImVec2 ImClamp(const ImVec2& f, const ImVec2& mn, ImVec2 mx) { return ImVec2(ImClamp(f.x,mn.x,mx.x), ImClamp(f.y,mn.y,mx.y)); }
|
||||
static inline float ImSaturate(float f) { return (f < 0.0f) ? 0.0f : (f > 1.0f) ? 1.0f : f; }
|
||||
static inline void ImSwap(int& a, int& b) { int tmp = a; a = b; b = tmp; }
|
||||
static inline void ImSwap(float& a, float& b) { float tmp = a; a = b; b = tmp; }
|
||||
static inline int ImLerp(int a, int b, float t) { return (int)(a + (b - a) * t); }
|
||||
static inline float ImLerp(float a, float b, float t) { return a + (b - a) * t; }
|
||||
static inline ImVec2 ImLerp(const ImVec2& a, const ImVec2& b, float t) { return ImVec2(a.x + (b.x - a.x) * t, a.y + (b.y - a.y) * t); }
|
||||
static inline ImVec2 ImLerp(const ImVec2& a, const ImVec2& b, const ImVec2& t) { return ImVec2(a.x + (b.x - a.x) * t.x, a.y + (b.y - a.y) * t.y); }
|
||||
static inline ImVec4 ImLerp(const ImVec4& a, const ImVec4& b, float t) { return ImVec4(a.x + (b.x - a.x) * t, a.y + (b.y - a.y) * t, a.z + (b.z - a.z) * t, a.w + (b.w - a.w) * t); }
|
||||
static inline float ImLengthSqr(const ImVec2& lhs) { return lhs.x*lhs.x + lhs.y*lhs.y; }
|
||||
static inline float ImLengthSqr(const ImVec4& lhs) { return lhs.x*lhs.x + lhs.y*lhs.y + lhs.z*lhs.z + lhs.w*lhs.w; }
|
||||
static inline float ImInvLength(const ImVec2& lhs, float fail_value) { float d = lhs.x*lhs.x + lhs.y*lhs.y; if (d > 0.0f) return 1.0f / sqrtf(d); return fail_value; }
|
||||
|
@ -154,6 +157,7 @@ static inline float ImFloor(float f)
|
|||
static inline ImVec2 ImFloor(const ImVec2& v) { return ImVec2((float)(int)v.x, (float)(int)v.y); }
|
||||
static inline float ImDot(const ImVec2& a, const ImVec2& b) { return a.x * b.x + a.y * b.y; }
|
||||
static inline ImVec2 ImRotate(const ImVec2& v, float cos_a, float sin_a) { return ImVec2(v.x * cos_a - v.y * sin_a, v.x * sin_a + v.y * cos_a); }
|
||||
static inline float ImLinearSweep(float current, float target, float speed) { if (current < target) return ImMin(current + speed, target); if (current > target) return ImMax(current - speed, target); return current; }
|
||||
|
||||
// We call C++ constructor on own allocated memory via the placement "new(ptr) Type()" syntax.
|
||||
// Defining a custom placement new() with a dummy parameter allows us to bypass including <new> which on some platforms complains when user has disabled exceptions.
|
||||
|
@ -182,7 +186,8 @@ enum ImGuiButtonFlags_
|
|||
ImGuiButtonFlags_AlignTextBaseLine = 1 << 8, // vertically align button to match text baseline - ButtonEx() only // FIXME: Should be removed and handled by SmallButton(), not possible currently because of DC.CursorPosPrevLine
|
||||
ImGuiButtonFlags_NoKeyModifiers = 1 << 9, // disable interaction if a key modifier is held
|
||||
ImGuiButtonFlags_AllowOverlapMode = 1 << 10, // require previous frame HoveredId to either match id or be null before being usable
|
||||
ImGuiButtonFlags_NoNavOverride = 1 << 11 // don't override navigation id when activated
|
||||
ImGuiButtonFlags_NoHoldingActiveID = 1 << 11, // don't set ActiveId while holding the mouse (ImGuiButtonFlags_PressedOnClick only)
|
||||
ImGuiButtonFlags_NoNavFocus = 1 << 12 // don't override navigation focus when activated
|
||||
};
|
||||
|
||||
enum ImGuiSliderFlags_
|
||||
|
@ -255,7 +260,7 @@ enum ImGuiNavHighlightFlags_
|
|||
{
|
||||
ImGuiNavHighlightFlags_TypeDefault = 1 << 0,
|
||||
ImGuiNavHighlightFlags_TypeThin = 1 << 1,
|
||||
ImGuiNavHighlightFlags_AlwaysRender = 1 << 2
|
||||
ImGuiNavHighlightFlags_AlwaysDraw = 1 << 2
|
||||
};
|
||||
|
||||
enum ImGuiCorner
|
||||
|
@ -443,8 +448,10 @@ struct ImGuiContext
|
|||
ImGuiID HoveredId; // Hovered widget
|
||||
bool HoveredIdAllowOverlap;
|
||||
ImGuiID HoveredIdPreviousFrame;
|
||||
float HoveredIdTimer;
|
||||
ImGuiID ActiveId; // Active widget
|
||||
ImGuiID ActiveIdPreviousFrame;
|
||||
float ActiveIdTimer;
|
||||
bool ActiveIdIsAlive; // Active widget has been seen this frame
|
||||
bool ActiveIdIsJustActivated; // Set at the time of activation for one frame
|
||||
bool ActiveIdAllowOverlap; // Active widget allows another widget to steal active id (generally for overlapping widgets, but not always)
|
||||
|
@ -452,8 +459,8 @@ struct ImGuiContext
|
|||
ImVec2 ActiveIdClickOffset; // Clicked offset from upper-left corner, if applicable (currently only set by ButtonBehavior)
|
||||
ImGuiWindow* ActiveIdWindow;
|
||||
ImGuiInputSource ActiveIdSource; // Activating with mouse or nav (gamepad/keyboard)
|
||||
ImGuiWindow* MovedWindow; // Track the child window we clicked on to move a window (only apply to moving with mouse)
|
||||
ImGuiID MovedWindowMoveId; // == MovedWindow->RootWindow->MoveId
|
||||
ImGuiWindow* MovingWindow; // Track the child window we clicked on to move a window.
|
||||
ImGuiID MovingWindowMoveId; // == MovingWindow->MoveId
|
||||
ImVector<ImGuiIniData> Settings; // .ini Settings
|
||||
float SettingsDirtyTimer; // Save .ini Settings on disk when time reaches zero
|
||||
ImVector<ImGuiColMod> ColorModifiers; // Stack for PushStyleColor()/PopStyleColor()
|
||||
|
@ -463,13 +470,14 @@ struct ImGuiContext
|
|||
ImVector<ImGuiPopupRef> CurrentPopupStack; // Which level of BeginPopup() we are in (reset every frame)
|
||||
|
||||
// Navigation data (for gamepad/keyboard)
|
||||
ImGuiWindow* NavWindow; // Nav/focused window for navigation
|
||||
ImGuiID NavId; // Nav/focused item for navigation
|
||||
ImGuiID NavActivateId, NavActivateDownId; // ~~ IsNavInputPressed(ImGuiNavInput_PadActivate) ? NavId : 0, etc.
|
||||
ImGuiID NavInputId; // ~~ IsNavInputPressed(ImGuiNavInput_PadInput) ? NavId : 0, etc.
|
||||
ImGuiWindow* NavWindow; // Focused window for navigation. Could be called 'FocusWindow'
|
||||
ImGuiID NavId; // Focused item for navigation
|
||||
ImGuiID NavActivateId; // ~~ IsNavInputPressed(ImGuiNavInput_PadActivate) ? NavId : 0, also set when calling ActivateItem()
|
||||
ImGuiID NavActivateDownId; // ~~ IsNavInputPressed(ImGuiNavInput_PadActivate) ? NavId : 0
|
||||
ImGuiID NavInputId; // ~~ IsNavInputPressed(ImGuiNavInput_PadInput) ? NavId : 0
|
||||
ImGuiID NavJustTabbedId; // Just tabbed to this id.
|
||||
ImGuiID NavJustNavigatedId; // Just navigated to this id (result of a successfully MoveRequest)
|
||||
ImGuiID NavNextActivateId; // Set by ActivateItem(), queued until next frame
|
||||
ImGuiID NavJustMovedToId; // Just navigated to this id (result of a successfully MoveRequest)
|
||||
ImRect NavScoringRectScreen; // Rectangle used for scoring, in screen space. Based of window->DC.NavRefRectRel[], modified for directional navigation scoring.
|
||||
ImGuiWindow* NavWindowingTarget;
|
||||
float NavWindowingDisplayAlpha;
|
||||
|
@ -492,9 +500,8 @@ struct ImGuiContext
|
|||
ImGuiDir NavMoveDirLast; // Direction of the previous move request
|
||||
ImGuiID NavMoveResultId; // Best move request candidate
|
||||
ImGuiID NavMoveResultParentId; //
|
||||
float NavMoveResultDistBox; // Best move request candidate box distance to current NavId
|
||||
float NavMoveResultDistCenter; // Best move request candidate center distance to current NavId
|
||||
float NavMoveResultDistAxial;
|
||||
float NavMoveResultDistCenter;
|
||||
ImRect NavMoveResultRectRel; // Best move request candidate bounding box in window relative space
|
||||
|
||||
// Storage for SetNexWindow** and SetNextTreeNode*** functions
|
||||
|
@ -571,8 +578,10 @@ struct ImGuiContext
|
|||
HoveredId = 0;
|
||||
HoveredIdAllowOverlap = false;
|
||||
HoveredIdPreviousFrame = 0;
|
||||
HoveredIdTimer = 0.0f;
|
||||
ActiveId = 0;
|
||||
ActiveIdPreviousFrame = 0;
|
||||
ActiveIdTimer = 0.0f;
|
||||
ActiveIdIsAlive = false;
|
||||
ActiveIdIsJustActivated = false;
|
||||
ActiveIdAllowOverlap = false;
|
||||
|
@ -580,13 +589,13 @@ struct ImGuiContext
|
|||
ActiveIdClickOffset = ImVec2(-1,-1);
|
||||
ActiveIdWindow = NULL;
|
||||
ActiveIdSource = ImGuiInputSource_None;
|
||||
MovedWindow = NULL;
|
||||
MovedWindowMoveId = 0;
|
||||
MovingWindow = NULL;
|
||||
MovingWindowMoveId = 0;
|
||||
SettingsDirtyTimer = 0.0f;
|
||||
|
||||
NavWindow = NULL;
|
||||
NavId = NavActivateId = NavActivateDownId = NavInputId = 0;
|
||||
NavJustTabbedId = NavJustNavigatedId = NavNextActivateId = 0;
|
||||
NavJustTabbedId = NavJustMovedToId = NavNextActivateId = 0;
|
||||
NavScoringRectScreen = ImRect();
|
||||
NavWindowingTarget = NULL;
|
||||
NavWindowingDisplayAlpha = 0.0f;
|
||||
|
@ -607,7 +616,7 @@ struct ImGuiContext
|
|||
NavMoveDir = NavMoveDirLast = ImGuiDir_None;
|
||||
NavMoveResultId = 0;
|
||||
NavMoveResultParentId = 0;
|
||||
NavMoveResultDistBox = NavMoveResultDistCenter = NavMoveResultDistAxial = 0.0f;
|
||||
NavMoveResultDistAxial = NavMoveResultDistCenter = 0.0f;
|
||||
|
||||
SetNextWindowPosVal = ImVec2(0.0f, 0.0f);
|
||||
SetNextWindowSizeVal = ImVec2(0.0f, 0.0f);
|
||||
|
@ -659,7 +668,7 @@ enum ImGuiItemFlags_
|
|||
{
|
||||
ImGuiItemFlags_AllowKeyboardFocus = 1 << 0, // true
|
||||
ImGuiItemFlags_ButtonRepeat = 1 << 1, // false // Button() will return true multiple times based on io.KeyRepeatDelay and io.KeyRepeatRate settings.
|
||||
//ImGuiItemFlags_Disabled = 1 << 2, // false // All widgets appears are disabled
|
||||
ImGuiItemFlags_Disabled = 1 << 2, // false // FIXME-WIP: Disable interactions but doesn't affect visuals. Should be: grey out and disable interactions with widgets that affect data + view widgets (WIP)
|
||||
ImGuiItemFlags_NoNav = 1 << 3, // false
|
||||
ImGuiItemFlags_NoNavDefaultFocus = 1 << 4, // false
|
||||
ImGuiItemFlags_SelectableDontClosePopup = 1 << 5, // false // MenuItem/Selectable() automatically closes current Popup window
|
||||
|
@ -844,6 +853,17 @@ public:
|
|||
ImRect MenuBarRect() const { float y1 = Pos.y + TitleBarHeight(); return ImRect(Pos.x, y1, Pos.x + SizeFull.x, y1 + MenuBarHeight()); }
|
||||
};
|
||||
|
||||
// Backup and restore just enough data to be able to use IsItemHovered() on item A after another B in the same window has overwritten the data.
|
||||
struct ImGuiItemHoveredDataBackup
|
||||
{
|
||||
ImGuiID LastItemId;
|
||||
ImRect LastItemRect;
|
||||
bool LastItemRectHoveredRect;
|
||||
|
||||
void Backup() { ImGuiWindow* window = GImGui->CurrentWindow; LastItemId = window->DC.LastItemId; LastItemRect = window->DC.LastItemRect; LastItemRectHoveredRect = window->DC.LastItemRectHoveredRect; }
|
||||
void Restore() { ImGuiWindow* window = GImGui->CurrentWindow; window->DC.LastItemId = LastItemId; window->DC.LastItemRect = LastItemRect; window->DC.LastItemRectHoveredRect = LastItemRectHoveredRect; }
|
||||
};
|
||||
|
||||
//-----------------------------------------------------------------------------
|
||||
// Internal API
|
||||
// No guarantee of forward compatibility here.
|
||||
|
@ -865,9 +885,11 @@ namespace ImGui
|
|||
IMGUI_API void EndFrame(); // Ends the ImGui frame. Automatically called by Render()! you most likely don't need to ever call that yourself directly. If you don't need to render you can call EndFrame() but you'll have wasted CPU already. If you don't need to render, don't create any windows instead!
|
||||
|
||||
IMGUI_API void SetActiveID(ImGuiID id, ImGuiWindow* window);
|
||||
IMGUI_API void SetActiveIDNoNav(ImGuiID id, ImGuiWindow* window);
|
||||
IMGUI_API ImGuiID GetActiveID();
|
||||
IMGUI_API void SetFocusID(ImGuiID id, ImGuiWindow* window);
|
||||
IMGUI_API void ClearActiveID();
|
||||
IMGUI_API void SetHoveredID(ImGuiID id);
|
||||
IMGUI_API ImGuiID GetHoveredID();
|
||||
IMGUI_API void KeepAliveID(ImGuiID id);
|
||||
|
||||
IMGUI_API void ItemSize(const ImVec2& size, float text_offset_y = 0.0f);
|
||||
|
@ -887,9 +909,11 @@ namespace ImGui
|
|||
IMGUI_API void ClosePopup(ImGuiID id);
|
||||
IMGUI_API bool IsPopupOpen(ImGuiID id);
|
||||
IMGUI_API bool BeginPopupEx(ImGuiID id, ImGuiWindowFlags extra_flags);
|
||||
IMGUI_API void BeginTooltipEx(ImGuiWindowFlags extra_flags, bool override_previous_tooltip = true);
|
||||
|
||||
IMGUI_API int CalcTypematicPressedRepeatAmount(float t, float t_prev, float repeat_delay, float repeat_rate);
|
||||
|
||||
IMGUI_API void Scrollbar(ImGuiLayoutType direction);
|
||||
IMGUI_API void VerticalSeparator(); // Vertical separator, for menu bars (use current line height). not exposed because it is misleading what it doesn't have an effect on regular layout.
|
||||
|
||||
// FIXME-WIP: New Columns API
|
||||
|
@ -909,9 +933,9 @@ namespace ImGui
|
|||
IMGUI_API void RenderFrame(ImVec2 p_min, ImVec2 p_max, ImU32 fill_col, bool border = true, float rounding = 0.0f);
|
||||
IMGUI_API void RenderFrameBorder(ImVec2 p_min, ImVec2 p_max, float rounding = 0.0f);
|
||||
IMGUI_API void RenderColorRectWithAlphaCheckerboard(ImVec2 p_min, ImVec2 p_max, ImU32 fill_col, float grid_step, ImVec2 grid_off, float rounding = 0.0f, int rounding_corners_flags = ~0);
|
||||
IMGUI_API void RenderCollapseTriangle(ImVec2 pos, bool is_open, float scale = 1.0f);
|
||||
IMGUI_API void RenderTriangle(ImVec2 pos, ImGuiDir dir, float scale = 1.0f);
|
||||
IMGUI_API void RenderBullet(ImVec2 pos);
|
||||
IMGUI_API void RenderCheckMark(ImVec2 pos, ImU32 col);
|
||||
IMGUI_API void RenderCheckMark(ImVec2 pos, ImU32 col, float sz);
|
||||
IMGUI_API void RenderNavHighlight(const ImRect& bb, ImGuiID id, ImGuiNavHighlightFlags flags = ImGuiNavHighlightFlags_TypeDefault); // Navigation highlight
|
||||
IMGUI_API void RenderRectFilledRangeH(ImDrawList* draw_list, const ImRect& rect, ImU32 col, float x_start_norm, float x_end_norm, float rounding);
|
||||
IMGUI_API const char* FindRenderedTextEnd(const char* text, const char* text_end = NULL); // Find the optional ## from which we stop displaying text.
|
||||
|
@ -946,6 +970,10 @@ namespace ImGui
|
|||
IMGUI_API int ParseFormatPrecision(const char* fmt, int default_value);
|
||||
IMGUI_API float RoundScalar(float value, int decimal_precision);
|
||||
|
||||
// Shade functions
|
||||
IMGUI_API void ShadeVertsLinearColorGradientKeepAlpha(ImDrawVert* vert_start, ImDrawVert* vert_end, ImVec2 gradient_p0, ImVec2 gradient_p1, ImU32 col0, ImU32 col1);
|
||||
IMGUI_API void ShadeVertsLinearAlphaGradientForLeftToRightText(ImDrawVert* vert_start, ImDrawVert* vert_end, float gradient_p0_x, float gradient_p1_x);
|
||||
|
||||
} // namespace ImGui
|
||||
|
||||
// ImFontAtlas internals
|
||||
|
|
Loading…
Reference in New Issue